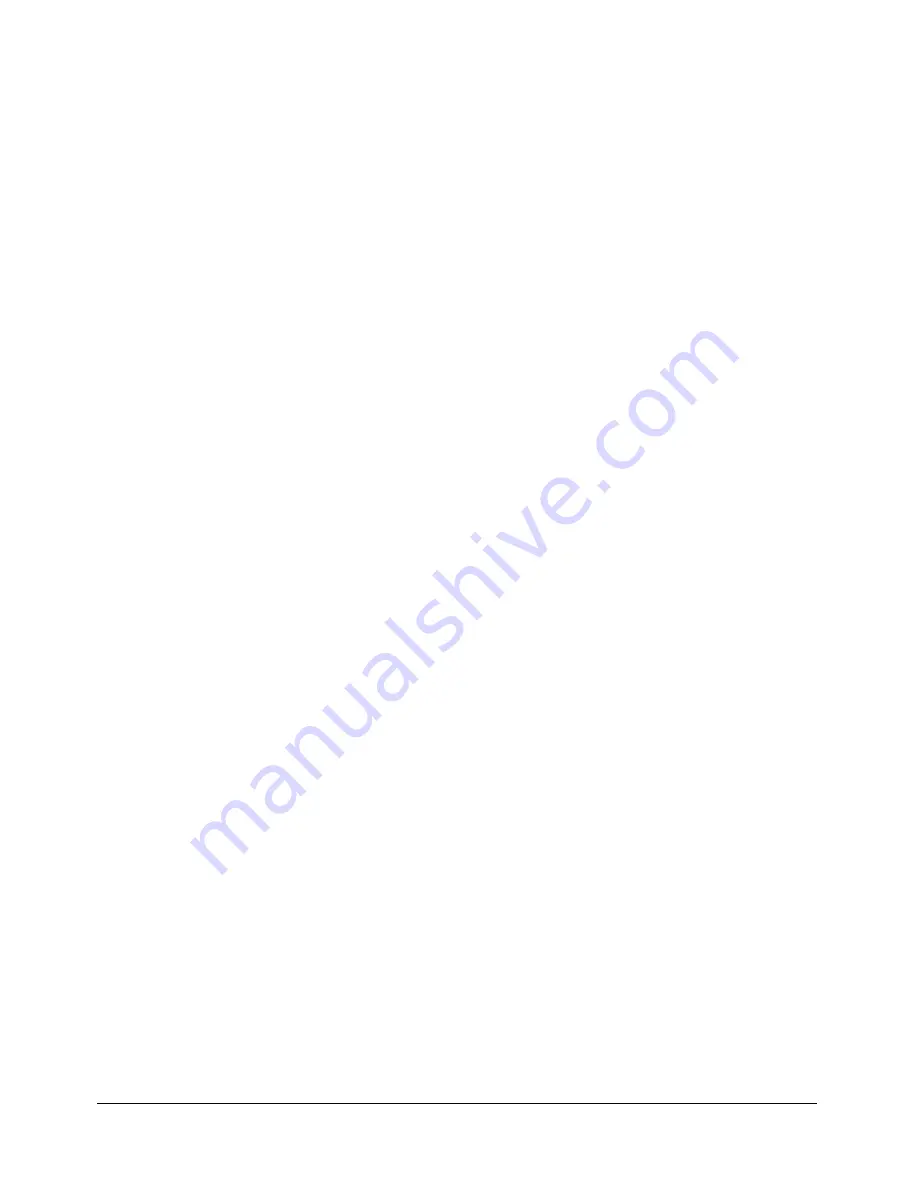
30
Chapter 1: ActionScript Basics
var myWebSite = "http://www.macromedia.com";
getURL(myWebSite); // browser displays www.macromedia.com
You can change the value of a variable in a script as many times as you want.
The type of data that a variable contains affects how and when the variable’s value changes.
Primitive data types, such as strings and numbers, are “passed by value”; this means that the
current value of the variable is used, rather than a reference to that value.
In the following example,
x
is set to 15 and that value is copied into
y
. When
x
is changed to 30
in line 3, the value of
y
remains 15, because
y
doesn’t look to
x
for its value; it contains the value
of
x
that it received in line 2.
var x:Number = 15;
var y:Number = x;
var x:Number = 30;
trace(x); //
30
trace(y);
// 15
In the following example, the variable
inValue
contains a primitive value, 3, so that value is
passed to the
sqr()
function and the returned value is 9:
function sqr(x:Number):Number {
var x:Number = x * x;
return x;
}
var inValue:Number = 3;
var out:Number = sqr(inValue);
trace(inValue); //3
trace(out);
//9
The value of the variable
inValue
does not change, even though the value of
x
in the function
changes.
The object data type can contain such a large amount of complex information that a variable with
this type doesn’t hold an actual value; it holds a reference to a value. This reference is similar to an
alias that points to the contents of the variable. When the variable needs to know its value,
the reference asks for the contents and returns the answer without transferring the value to
the variable.
The following example shows passing by reference:
var myArray:Array = ["tom", "josie"];
var newArray:Array = myArray;
myArray[1] = "jack";
trace(newArray); // tom,jack
This code creates an Array object called
myArray
that has two elements. The variable
newArray
is
created and is passed a reference to
myArray
. When the second element of
myArray
is changed to
"
jack
", it affects every variable with a reference to it. The
trace()
statement sends
tom,jack
to
the log file. Flash uses a zero-based index, which means that 0 is the first item in the array, 1 is the
second, and so on.
Summary of Contents for FLEX-FLEX ACTIONSCRIPT LANGUAGE
Page 1: ...Flex ActionScript Language Reference...
Page 8: ......
Page 66: ...66 Chapter 2 Creating Custom Classes with ActionScript 2 0...
Page 76: ......
Page 133: ...break 133 See also for for in do while while switch case continue throw try catch finally...
Page 135: ...case 135 See also break default strict equality switch...
Page 146: ...146 Chapter 5 ActionScript Core Language Elements See also break continue while...
Page 808: ...808 Chapter 7 ActionScript for Flash...
Page 810: ...810 Appendix A Deprecated Flash 4 operators...
Page 815: ...Other keys 815 Num Lock 144 186 187 _ 189 191 192 219 220 221 222 Key Key code...
Page 816: ...816 Appendix B Keyboard Keys and Key Code Values...
Page 822: ...822 Index...