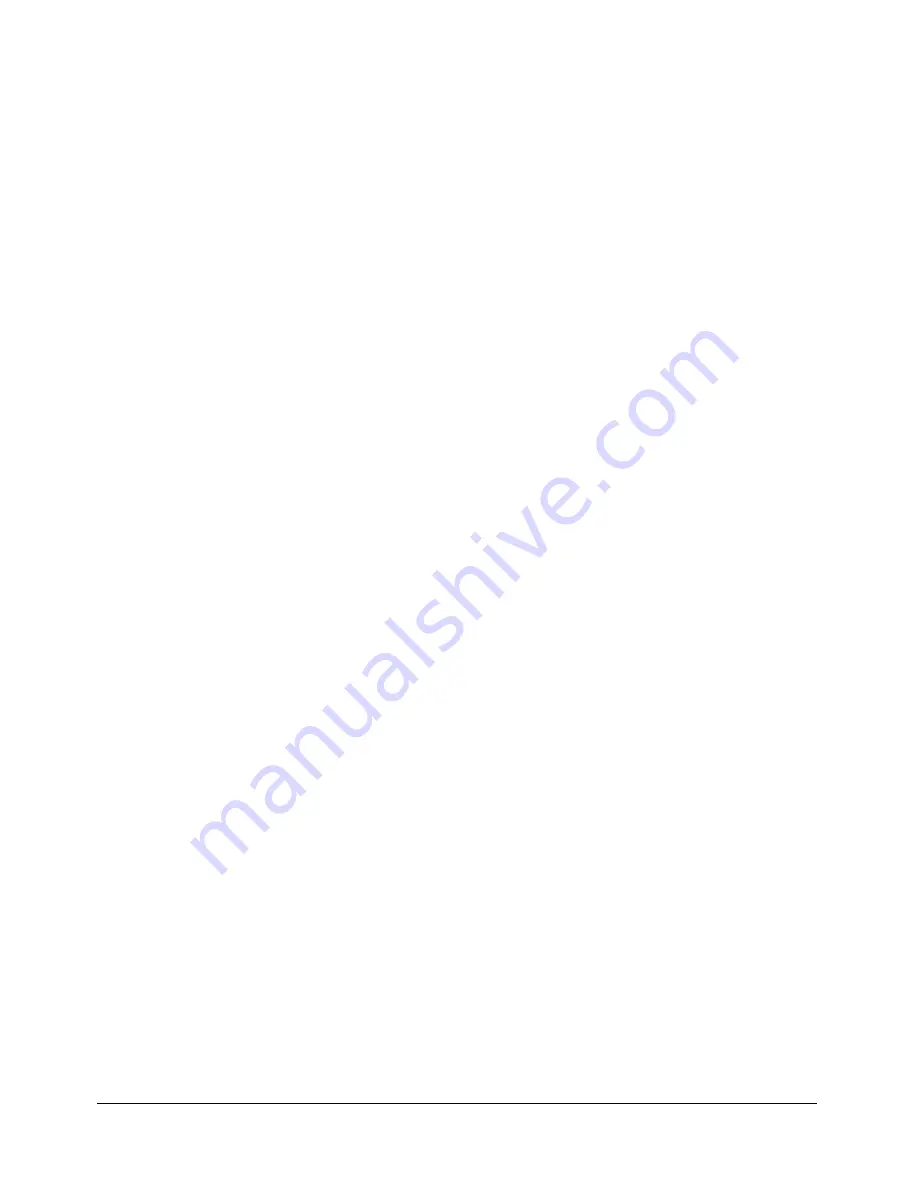
try..catch..finally
221
The following example demonstrates a
try..catch
statement. The code within the
try
block is
executed. If an exception is thrown by any code within the
try
block, control passes to the
catch
block, which shows the error message in a text field using the
Error.toString()
method.
In the same directory as Account.as, create a new FLA document and enter the following
ActionScript:
import Account;
var account:Account = new Account();
try {
var returnVal = account.getAccountInfo();
if (returnVal != 0) {
throw new Error("Error getting account information.");
}
trace("success");
} catch (e) {
this.createTextField("status_txt", this.getNextHighestDepth(), 0, 0, 100,
22);
status_txt.autoSize = true;
status_txt.text = e.toString();
}
The following example shows a
try
code block with multiple, typed
catch
code blocks.
Depending on the type of error that occurred, the
try
code block throws a different type of
object. In this case,
myRecordSet
is an instance of a (hypothetical) class named RecordSet whose
sortRows()
method can throw two types of errors, RecordSetException and MalformedRecord.
In the following example, the RecordSetException and MalformedRecord objects are subclasses of
the Error class. Each is defined in its own AS class file. (For more information, see
Chapter 2,
“Creating Custom Classes with ActionScript 2.0,” on page 45
.)
// In RecordSetException.as:
class RecordSetException extends Error {
var message = "Record set exception occurred.";
}
// In MalformedRecord.as:
class MalformedRecord extends Error {
var message = "Malformed record exception occurred.";
}
Within the RecordSet class’s
sortRows()
method, one of these previously defined error objects is
thrown, depending on the type of exception that occurred. The following example shows how
this code might look:
class RecordSet {
function sortRows() {
var returnVal:Number = randomNum();
if (returnVal == 1) {
throw new RecordSetException();
} else if (returnVal == 2) {
throw new MalformedRecord();
}
}
Summary of Contents for FLEX-FLEX ACTIONSCRIPT LANGUAGE
Page 1: ...Flex ActionScript Language Reference...
Page 8: ......
Page 66: ...66 Chapter 2 Creating Custom Classes with ActionScript 2 0...
Page 76: ......
Page 133: ...break 133 See also for for in do while while switch case continue throw try catch finally...
Page 135: ...case 135 See also break default strict equality switch...
Page 146: ...146 Chapter 5 ActionScript Core Language Elements See also break continue while...
Page 808: ...808 Chapter 7 ActionScript for Flash...
Page 810: ...810 Appendix A Deprecated Flash 4 operators...
Page 815: ...Other keys 815 Num Lock 144 186 187 _ 189 191 192 219 220 221 222 Key Key code...
Page 816: ...816 Appendix B Keyboard Keys and Key Code Values...
Page 822: ...822 Index...