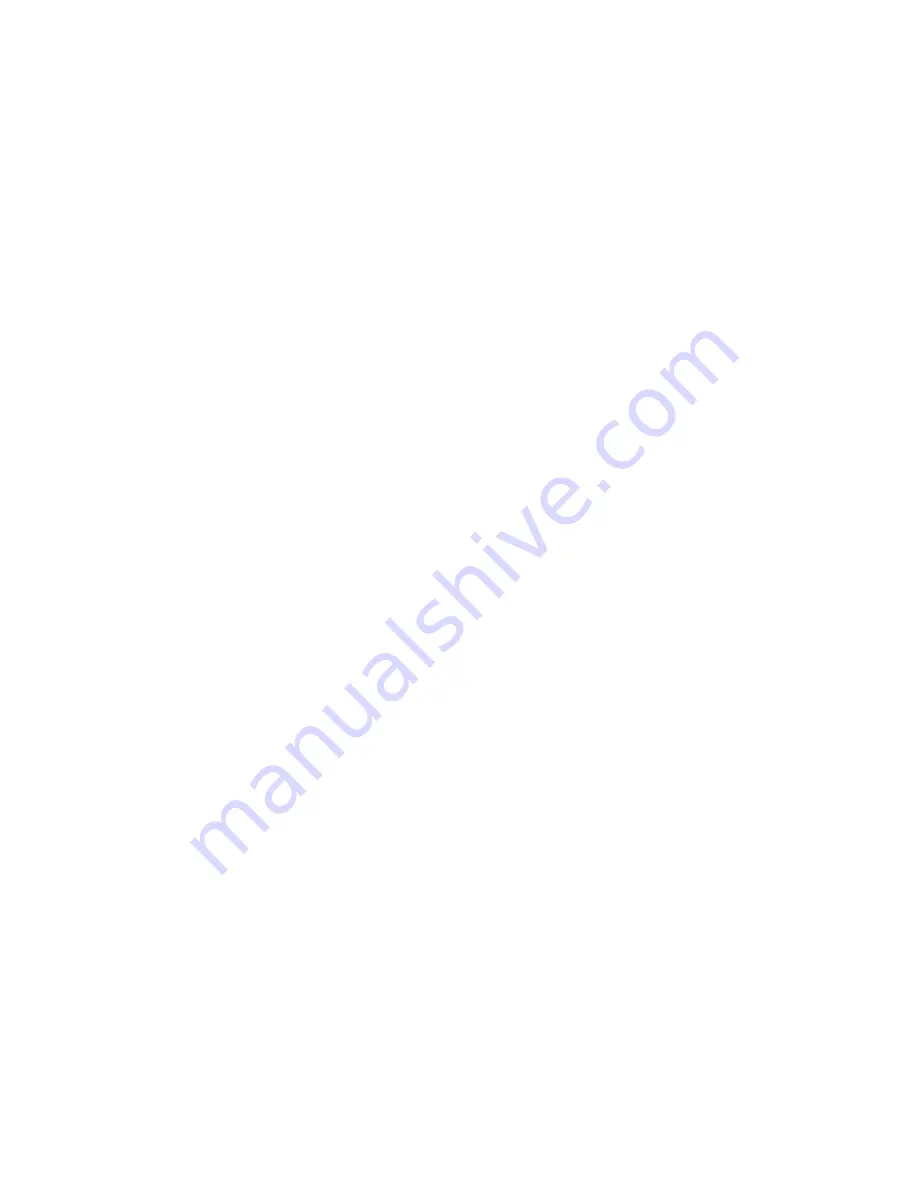
79
•
HEX (Char) Returns a HEX string that represents the character value passed in. Example:
HEX(“A”), HEX(var_name).
•
LStr(string, integer) Returns string
Return integer number of characters from the left of string. Example:
LStr(“abcdef”,3) returns “abc”
•
ltoa(integer) or itoa(integer) Returns string
Converts an integer to a string representation of the integer. Domain of return number:
-2147483648 to 2147483647. Example:
ltoa(31) or itoa(31)
•
Ltrim(string) Returns string
Remove leading spaces from string. Example:
Ltrim(“ test”) returns “test”
•
Padl(string, Length) Returns string
Pads a string with spaces on the left to the length specified. Returns a string. If Length
is less than the length of String, then String is returned. Example:
Padl(“ test”, 4)
•
Padr(string, Length) Returns string
Pads a string with spaces on the right to the length specified. Returns a string. If Length
is less than the length of String, then String is returned. Example:
Padr(“test “, 6)
•
replace(String, S1, S2) Search string for findstr and replace with newstr. If the string S1
cannot be found inside of String, just return the original string. Example:
replace(“abcdef”,”xyz”,”abc”) returns “abcdef”.
If the string to replace occurs more than once, replace each instance. Example:
replace(“abcabcabc”,”bc”,”Z”) returns “aZaZaZ”.
•
RStr(string, integer) Returns string
Return integer number of characters from the right of string. Example:
string=“abcdef”, RStr(string,3)=“def”
•
rstrstr(string, string2find) Returns integer
Searching Right to Left, finds string2find inside of string and returns the beginning location
of string2find.
Failure to find returns -1. Example:
string=“abcabc”, string2find=“bc”, returns 4
•
Rtrim(string) Returns string
Removes trailing spaces from string. Example:
Rtrim(“ test”) returns “test”
•
strlen(string) Returns Integer
Returns the number of characters in the string. Example:
strlen(“test”) returns “4”
•
strlwr(string) Returns string
Returns the lower case version of a string. Example:
strlwr(“TEST”) returns “test”