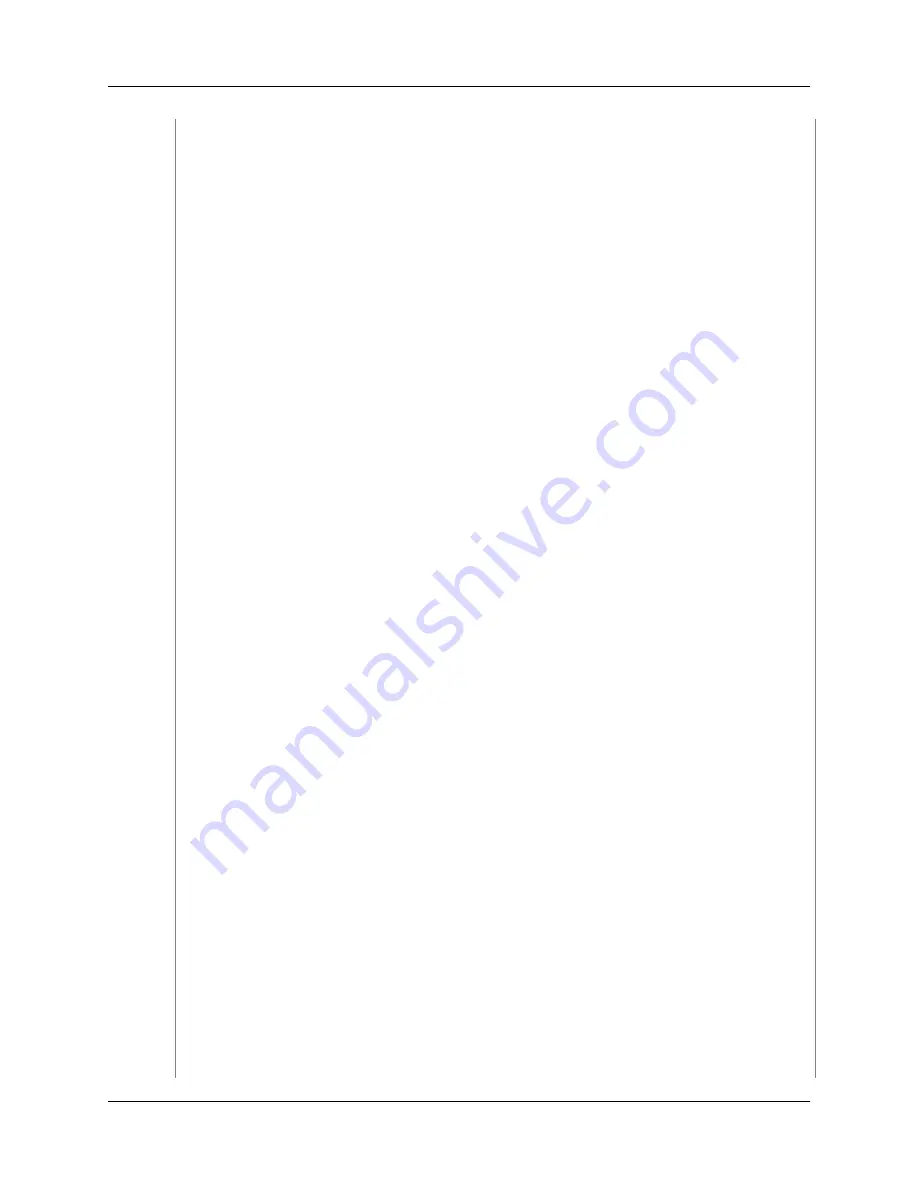
import com.amazonaws.services.redshift.model.DescribeClusterSnapshotsResult;
import com.amazonaws.services.redshift.model.Snapshot;
public class CreateAndDescribeSnapshot {
public static AmazonRedshiftClient client;
public static String clusterIdentifier = "***provide cluster identifier***";
public static long sleepTime = 10;
public static void main(String[] args) throws IOException {
AWSCredentials credentials = new PropertiesCredentials(
CreateAndDescribeSnapshot.class
.getResourceAsStream("AwsCredentials.properties"));
client = new AmazonRedshiftClient(credentials);
try {
// Unique snapshot identifier
String snapshotId = "my-snapshot-" + (new SimpleDateFormat("yyyy-
MM-dd-HH-mm-ss")).format(new Date());
Date createDate = createManualSnapshot(snapshotId);
waitForSnapshotAvailable(snapshotId);
describeSnapshots();
deleteManualSnapshotsBefore(createDate);
describeSnapshots();
} catch (Exception e) {
System.err.println("Operation failed: " + e.getMessage());
}
}
private static Date createManualSnapshot(String snapshotId) {
CreateClusterSnapshotRequest request = new CreateClusterSnapshotRequest()
.withClusterIdentifier(clusterIdentifier)
.withSnapshotIdentifier(snapshotId);
Snapshot snapshot = client.createClusterSnapshot(request);
System.out.format("Created cluster snapshot: %s\n", snapshotId);
return snapshot.getSnapshotCreateTime();
}
private static void describeSnapshots() {
DescribeClusterSnapshotsRequest request = new DescribeClusterSnapshots
Request()
.withClusterIdentifier(clusterIdentifier);
DescribeClusterSnapshotsResult result = client.describeClusterSnap
shots(request);
printResultSnapshots(result);
}
private static void deleteManualSnapshotsBefore(Date creationDate) {
API Version 2012-12-01
60
Amazon Redshift Management Guide
Managing Snapshots Using AWS SDK for Java