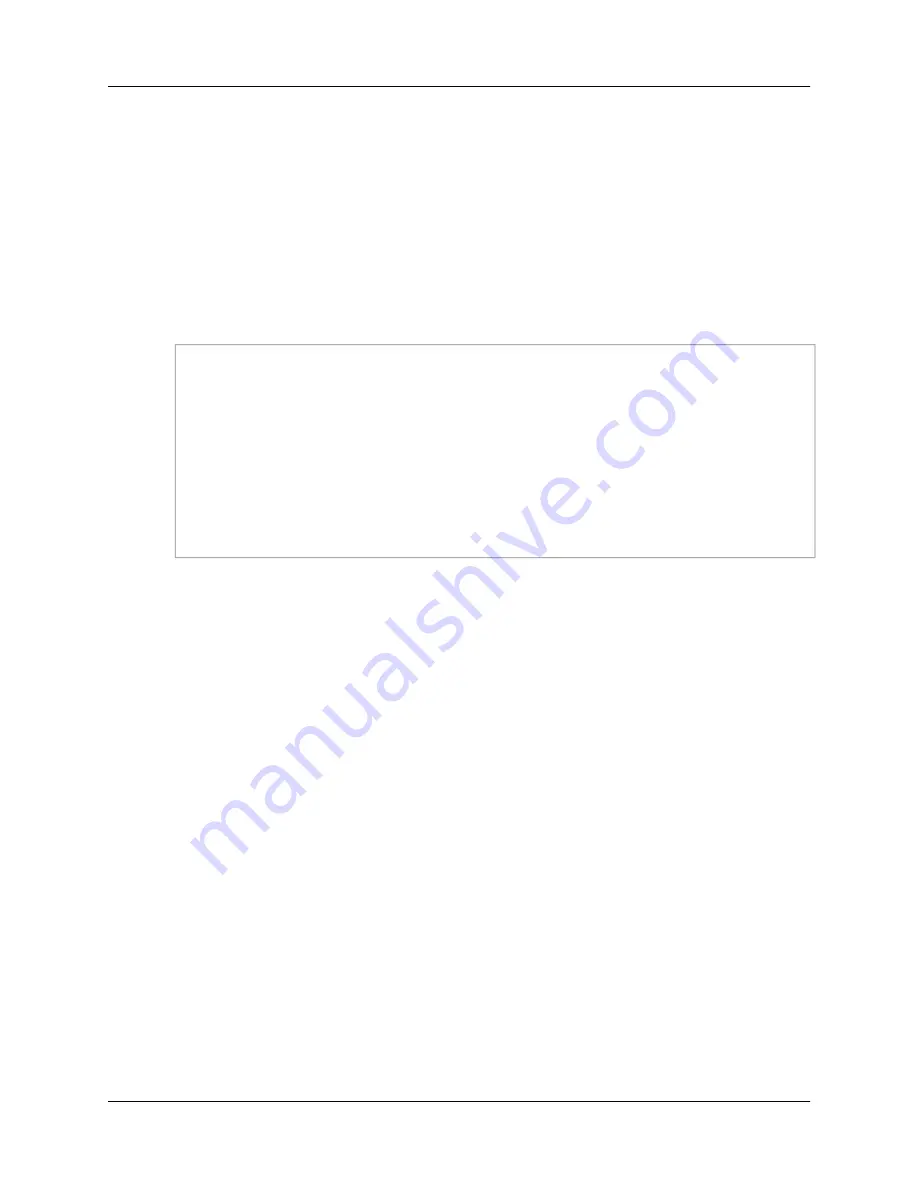
Note
The AWS SDK for Java provides thread-safe clients for accessing Amazon Redshift. As a best
practice, your applications should create one client and reuse the client between threads.
The
AmazonRedshiftClient
class defines methods that map to underlying Amazon Redshift Query
API actions. (These actions are described in the Amazon Redshift
API Reference
). When you call a
method, you must create a corresponding request object and response object. The request object includes
information that you must pass with the actual request. The response object include information returned
from Amazon Redshift in response to the request.
For example, the
AmazonRedshiftClient
class provides the
createCluster
method to provision a
cluster. This method maps to the underlying
CreateCluster
API action. You create a
CreateClusterRequest
object to pass information with the
createCluster
method.
AmazonRedshiftClient client = new AmazonRedshiftClient(credentials);
client.setEndpoint("https://redshift.us-east-1.amazonaws.com/");
CreateClusterRequest request = new CreateClusterRequest()
.withClusterIdentifier("exampleclusterusingjava")
.withMasterUsername("masteruser")
.withMasterUserPassword("12345678Aa")
.withNodeType("dw.hs1.xlarge")
.withNumberOfNodes(2);
Cluster createResponse = client.createCluster(request);
System.out.println("Created cluster " + createResponse.getClusterIdentifier());
Running Java Examples for Amazon Redshift
Using Eclipse
General Process of Running Java Code Examples Using Eclipse
1. Create a new AWS Java Project in Eclipse.
Follow the steps in
Setting Up the AWS Toolkit for Eclipse
in the AWS Toolkit for Eclipse Getting
Started Guide. These steps include configuring the
AwsCredentials.properties
file that contains
your AWS security credentials.
2. Copy the sample code from the section of this document that you are reading and paste it into your
project as a new Java class file.
3. Run the code.
Running Java Examples for Amazon Redshift from
the Command Line
General Process of Running Java Code Examples from the Command Line
1. Set up and test your environment as follows:
a. Create a directory to work in and in it create
src
,
bin
, and
sdk
subfolders.
b. Download the AWS SDK for Java and unzip it to the
sdk
subfolder you created. After you unzip the
SDK, you should have four subdirectories in the
sdk
folder, including a
lib
and
third-party
folder.
API Version 2012-12-01
118
Amazon Redshift Management Guide
Running Java Examples Using Eclipse