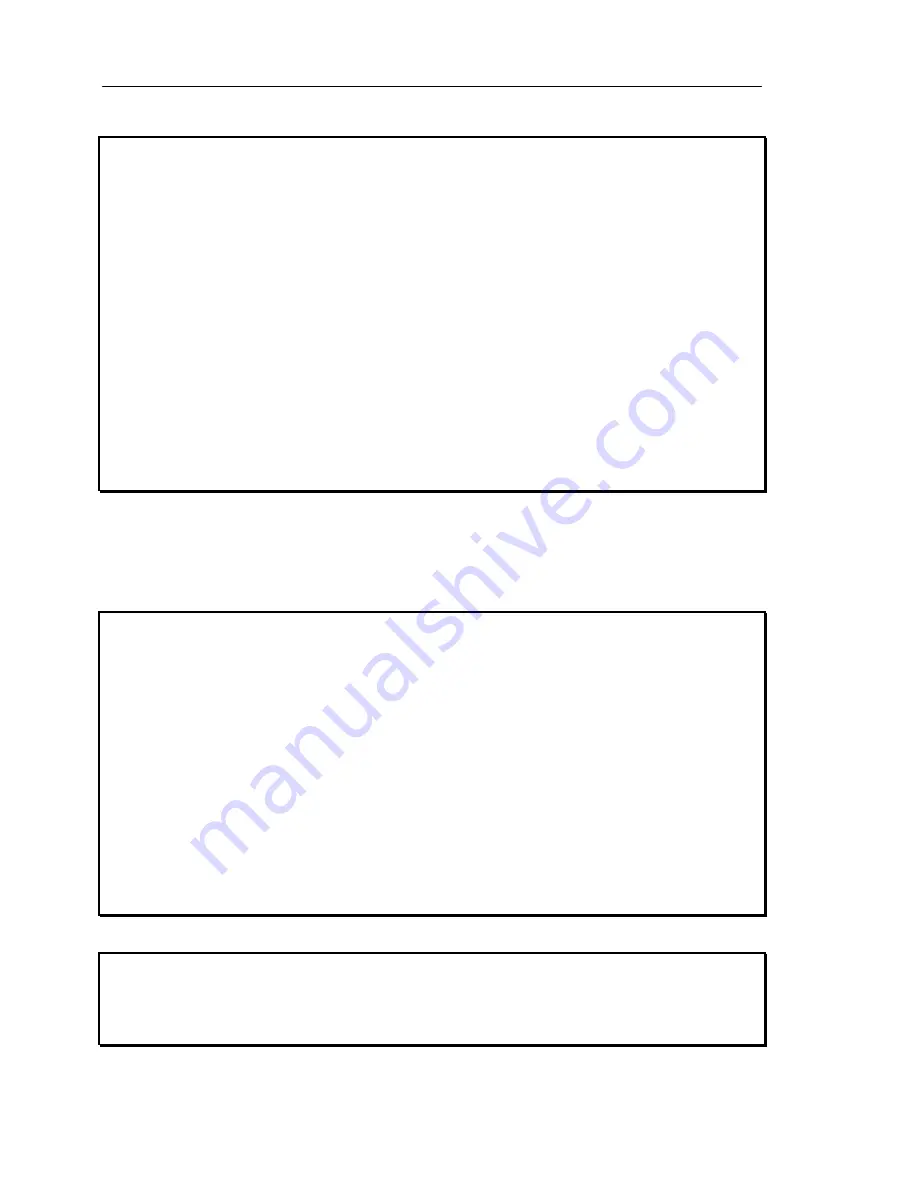
Chapter 4: Software
R-Engine-D
4-12
This function places a string of the current value of the real time clock in the char* realTime.
The text string has a format of “year1000 year100 year10 year1 month10 month1 day10 day1 hour10
hour1 min10 min1 second10 second1”. For example” 19991220081020” presents year1999, december,
20
th
, eight clock 10 minutes, and 20 second.
This function returns 0 on success and returns 1 in case of error, such as the clock failing to respond.
Void rtc_init
Arguments:
char* t
Return value:
none
This function is used to initialize and set a value into the real-time clock. The argument
t
should be a null-
terminated byte array that contains the new time value to be used.
The byte array should correspond to {
weekday, year10, year1, month10, month1, day10, day1, hour10,
hour1, minute10, minute1, second10, second1,
0 }.
If, for example, the time to be initialized into the real time clock is June 5, 1998, Friday, 13:55:30, the byte
array would be initialized to:
unsigned char t[14] = { 5, 9, 8, 0, 6, 0, 5, 1, 3, 5, 5, 3, 0 };
Delay
In many applications it becomes useful to pause before executing any further code. There are functions
provided to make this process easy. For applications that require precision timing, you should use
hardware timers provided on-board for this purpose.
void delay0
Arguments:
unsigned int t
Return value:
none
This function is just a simple software loop. The actual time that it waits depends on processor speed as
well as interrupt latency. The code is functionally identical to:
While(t) { t--; }
Passing in a
t
value of 600 causes a delay of approximately 1 ms.
void delay_ms
Arguments:
unsigned int
Return value:
none
This function is similar to delay0, but the passed in argument is in units of milliseconds instead of loop
iterations. Again, this function is highly dependent upon the processor speed.
unsigned int crc16
Arguments:
unsigned char *wptr, unsigned int count
Return value:
unsigned int value
This function returns a simple 16-bit CRC on a byte-array of
count
size pointed to by
wptr
.