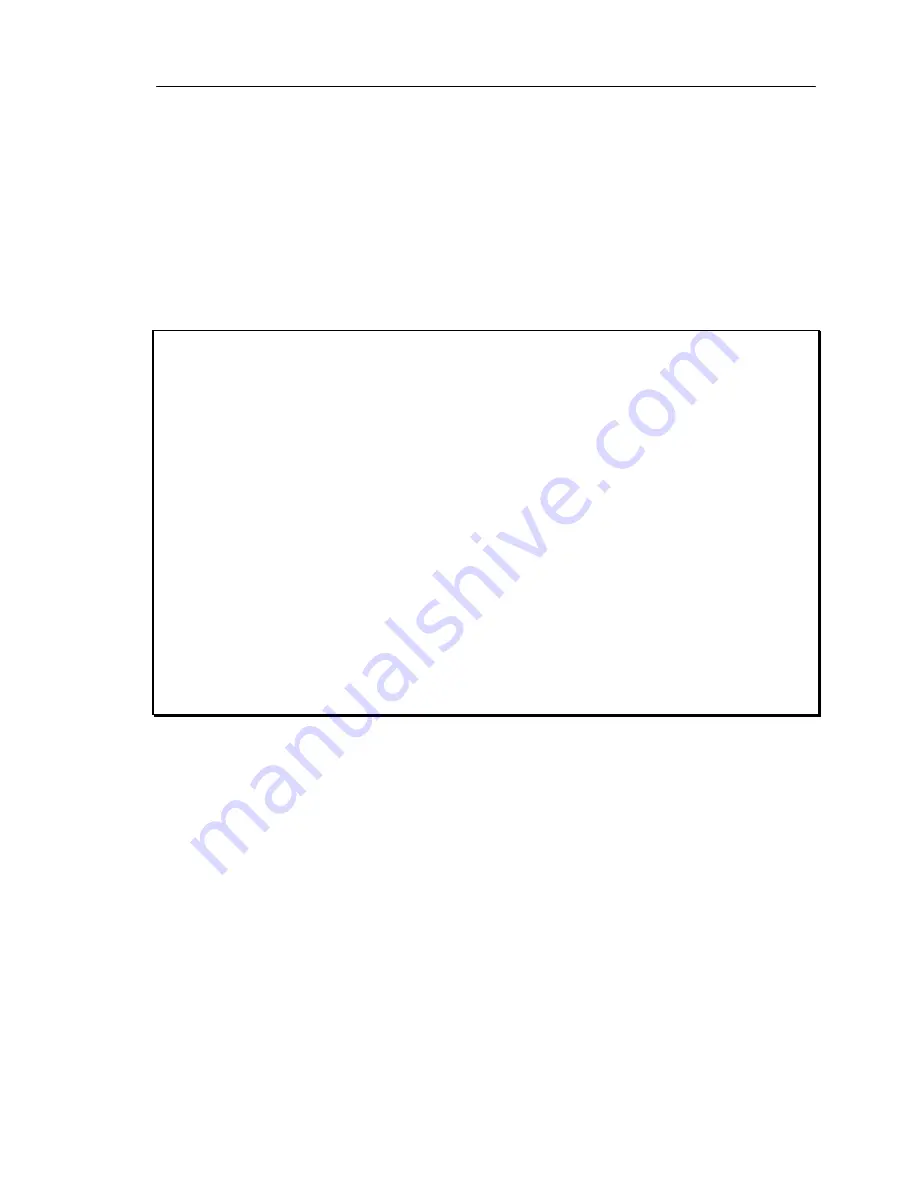
R-Engine-D
Chapter 4: Software
4-7
interrupt should be enabled, and the second is a function pointer to an appropriate interrupt service routine
that should be used to handle the interrupt. The TERN libraries will set up the interrupt vectors correctly
for the specified external interrupt line.
At the end of interrupt handlers, the appropriate in-service bit for the IR signal currently being handled
must be cleared. This can be done using the
Nonspecific EOI command
. At initialization time, interrupt
priority was placed in
Fully Nested
mode. This means the current highest priority interrupt will be handled
first, and a higher priority interrupt will interrupt any current interrupt handlers. So, if the user chooses to
clear the in-service bit for the interrupt currently being handled, the interrupt service routine just needs to
issue the nonspecific EOI command to clear the current highest priority IR.
To send the nonspecific EOI command, you need to write the
EOI
register word with 0x8000.
outport(0xff22, 0x8000);
void int
x
_init
Arguments: unsigned char i, void interrupt far(* int
x
_isr) () )
Return value: none
These functions can be used to initialize any one of the external interrupt channels (for pin locations and
other physical hardware details, see the Hardware chapter). The first argument
i
indicates whether this
particular interrupt should be enabled or disabled. The second argument is a function pointer, which will
act as the interrupt service routine. The overhead on the interrupt service routine, when executed, is about
20
µ
s.
By default, the interrupts are all disabled after initialization. To disable them again, you can repeat the call
but pass in 0 as the first argument.
The NMI (Non-Maskable Interrupt) is special in that it can not be masked (disabled). The default ISR will
return on interrupt.
void int0_init( unsigned char i, void interrupt far(* int0_isr)() );
void int1_init( unsigned char i, void interrupt far(* int1_isr)() );
void int2_init( unsigned char i, void interrupt far(* int2_isr)() );
void int3_init( unsigned char i, void interrupt far(* int3_isr)() );
void int4_init( unsigned char i, void interrupt far(* int4_isr)() );
void nmi_init(void interrupt far (* nmi_isr)());
4.3.3
I/O Initialization
Two ports of 16 I/O pins each are available on the R-Engine-D. Hardware details regarding these PIO lines
can be found in the Hardware chapter.
Several functions are provided for access to the PIO lines. At the beginning of any application where you
choose to use the PIO pins as input/output, you will probably need to initialize these pins in one of the four
available modes. Before selecting pins for this purpose, make sure that the peripheral mode operation of
the pin is not needed for a different use within the same application.
You should also confirm the PIO usage that is described above within
ae_init()
. During initialization,
several lines are reserved for TERN usage and you should understand that these are not available for your
application. There are several PIO lines that are used for other on-board purposes. These are all described
in some detail in the Hardware chapter of this technical manual. For a detailed discussion toward the I/O
ports, please refer to Chapter 14 of the AMD Am186ER User’s Manual.
Please see the sample program
ae_pio.c
in
tern\186\samples\ae
. You will also find that these
functions are used throughout TERN sample files, as most applications do find it necessary to re-configure
the PIO lines.
The function
pio_wr
and
pio_rd
can be quite slow when accessing the PIO pins. Depending on the pin
being used, it might require from 5-10 us. The maximum efficiency you can get from the PIO pins occur if