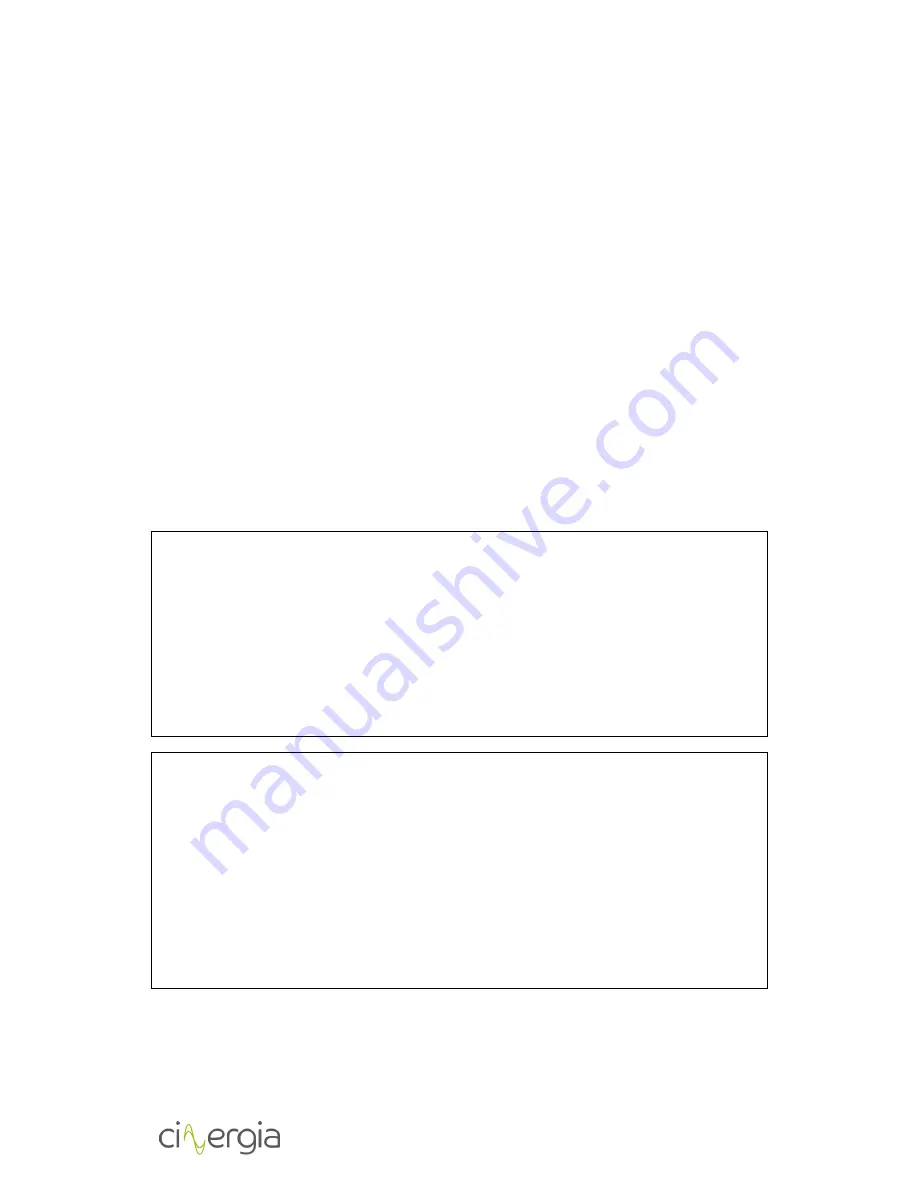
49
/ 55
6.1.
IQ MANAGEMENT
Many of the parameters of this equipment are defined as IQ numbers (Texas Instruments
nomenclature). An IQ number refers to a 32 bit signed fixed point number where the number
of fractional bits is specified. For instance, IQ21 means that the number has 21 fractional bits,
10 integer bits and 1 bit is for the sign.
For the representation of the negative numbers:
And for the positive numbers:
As an example, 1.4142 in IQ10 representation:
Below there is a C# sample code for the representation:
IQ10 functions:
public double IQ10toFloat(double Var)
{
if (Var > 2147483648) //if the value is bigger than 2^31 (positive)
{
Var = Var - 4294967296;
// Var - 2^32
Var = Var / (1024); // Var/(2^10)
}
else
{
Var = Var / (1024);
}
return Var;
}
public UInt32 FloatToIQ10(double Var)
{
UInt32 Retorn=0;
if (Var <0 )
// if negative
{
Var = (1024*Var) + 4294967296;
// x*2^10 + 2^32
}
else
{
Var = Var * (1024);
}
Retorn = Convert.ToUInt32(Var);
return Retorn;
}