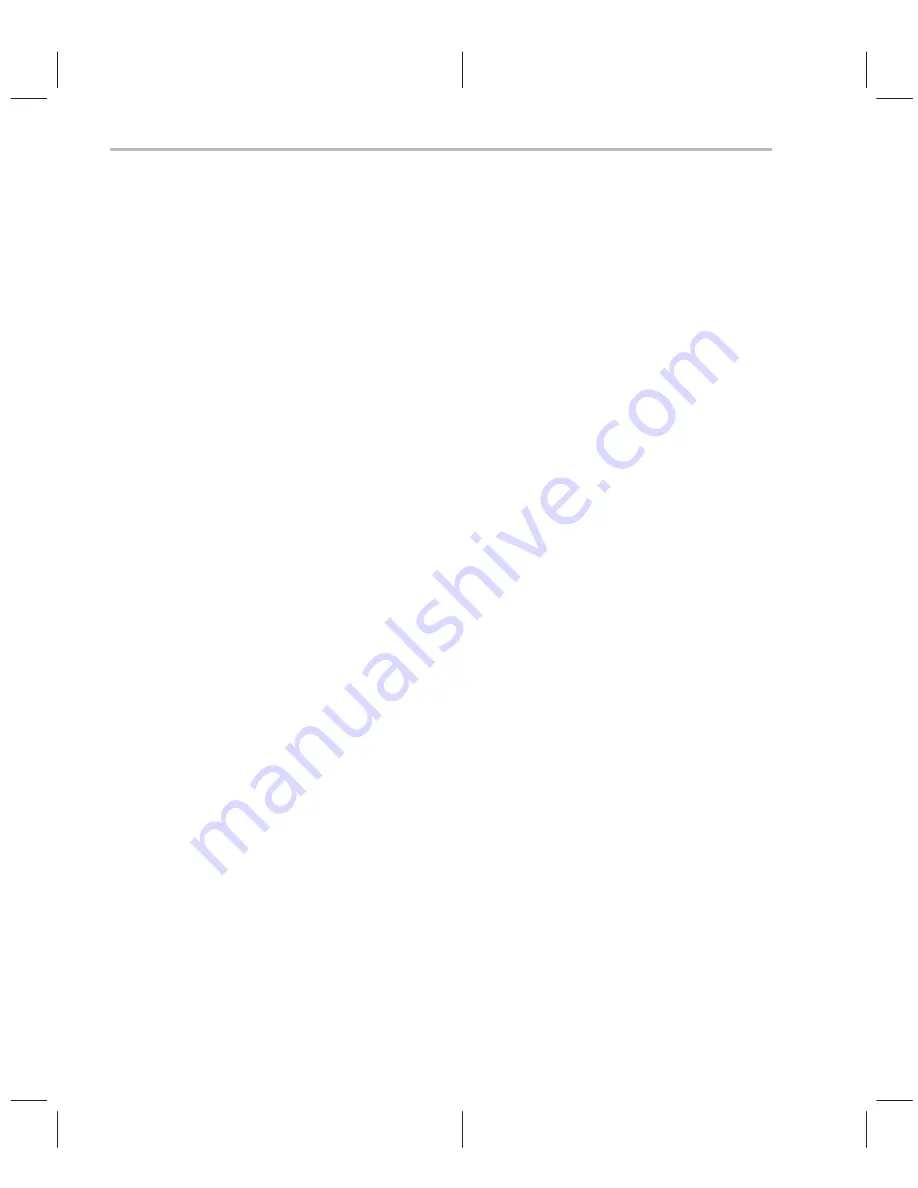
Writing CGI Functions
E-6
httpSendFullResponse()
Send full HTTP response, including a sta-
tus code and an HTML file
or
httpSendStatusLine()
Send HTTP status response, including a
status code and content type
httpSendClientStr()
Used after httpSendStatusLine() to send
content data
As an example of using these function, consider the two response MACROS
included in
hpptif.h
.
//
// Common error responses
//
#define http404(Sock) httpSendFullResponse(Sock, HTTP_NOT_FOUND, “404.htm”)
#define http501(Sock) httpSendStatusLine(Sock, HTTP_NOT_IMPLEMENTED, \
(char *)CONTENT_TYPE_HTML)
The first MACRO, http404() is used to send a “file not found” response to the
client. This includes the HTML text defined for the 404 error. An example of
creating a
404.htm
file appears earlier in this appendix.
The second MACRO, http501() is used to send a 501 – Not Implemented error
to the HTTP client. Here, no additional data is sent.
Under normal circumstances, the CGI function will use the httpSendStatus-
Line() function to send an OK message to the client, followed by the httpSend-
ClientStr() function to send client data in the form of a NULL terminated string.
Note that an additional carriage return and line feed are required to separate
the header from the HTML data.
For example, the following code sends a quick “Success” message.
// Send response status
httpSendStatusLine( Sock, HTTP_OK, CONTENT_TYPE_HTML );
// Terminate the response header
httpSendClientStr( Sock, CRLF );
// Send the Success Message
httpSendClientStr( Sock, ”<html><h1>Success!</h1><br></html>”);
Note that the httpSendClientStr() function replaces the httpSendClientData()
function from earlier releases of the NDK. For data sizes that can be repre-
sented by an integer, client data can also be sent simply by calling the sockets
send() function.