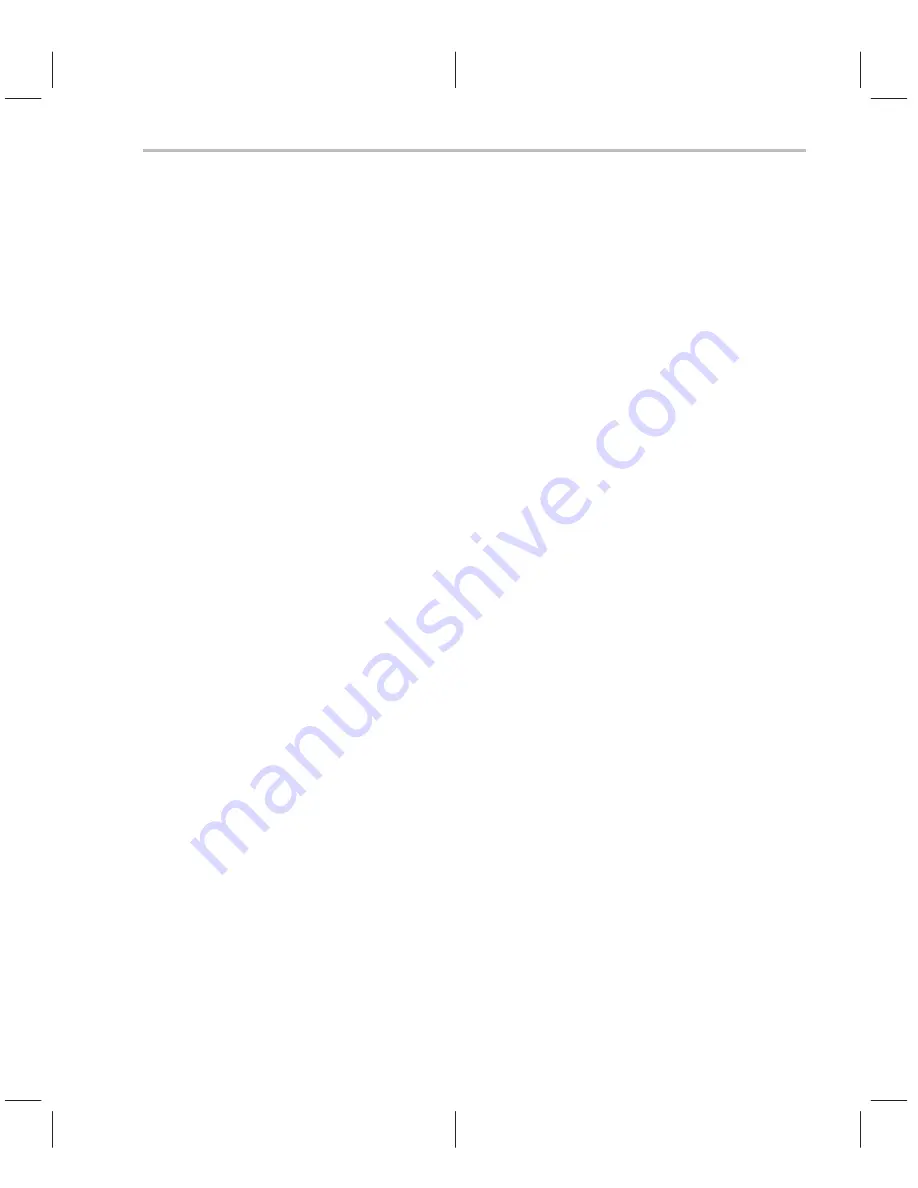
Low-Level PPP Support
C-9
Point to Point Protocol
C.1.6.4 Creating a Packet
Along with decoding any packet that is received on the physical hardware and
stripping off all but the protocol and payload fields, the SI module must supply
the received data as a packet (PKT) object to the pppInput() function. The fol-
lowing example code illustrates the creation of a packet object for holding new
packet data. This function assumes a packet with a max payload (MRU) of
1500 bytes
HANDLE SIModuleCreatePacket( calling parameters )
{
HANDLE hPkt;
HANDLE hFrag;
uint Offset,ValidSize;
UINT8 *pb;
// Create a packet with up to 1500 bytes of payload
// (If we informed the IF module of our header requirements, we
// know we’ll have room for our 2 byte header)
if( !(hPkt = IFCreatePacket( 1500, 0, 0 )) )
return( 0 );
// Get the memory frag for this packet
hFrag = PktGetFrag( hPkt );
// Get pointers to packet headers
pb = FragGetBufParams( hFrag, 0, 0, 0 );
Offset = PktGetSizeLLC( hPkt );
// The value of ”Offset” is that assigned by IFCreatePacket().
// Its probably 14 bytes, but we better make sure its at least 2
// bytes. We’ll take off 2 bytes (the size of our header) and start
// writing our data there. We know we can write up to 1502 bytes.
if( Offset <= 2 )
Offset = 0;
else
Offset –= 2;
// Set pb to point to where we start writing data
pb += Offset;
//–––––––––––––––––––––––––––––––––––––––––––––––––––––––––––
// Fill in the packet buffer here. The pointer ’pb’ now
// where the 2 byte protocol field should start.
//–––––––––––––––––––––––––––––––––––––––––––––––––––––––––––
//
// Fixup packet frag info
//
// The value of ’Offset’ is already valid, but we need to provide
// the size of the data. This size includes 2 byte protocol
// value. For example, for a 1500 byte IP packet, the value
// of ’PACKETSIZE’ is 1502