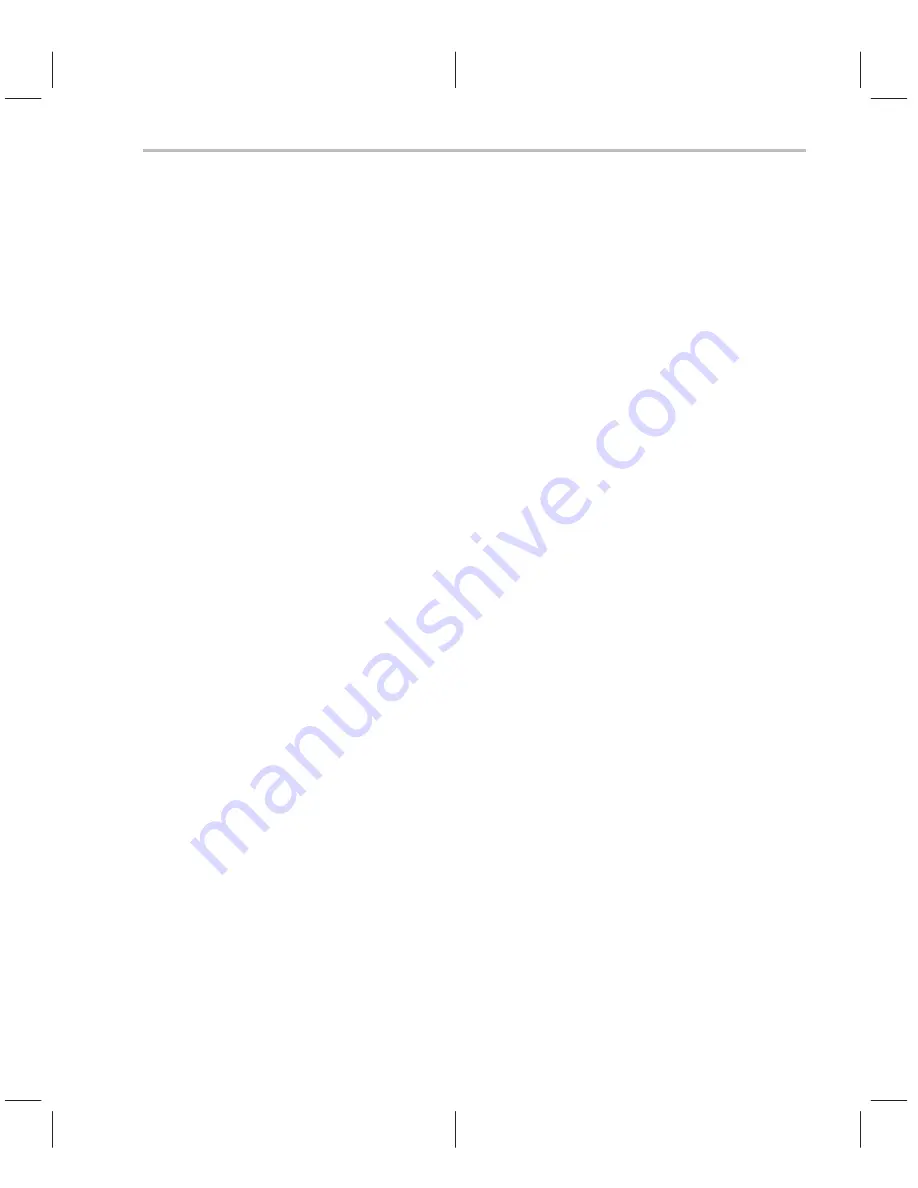
Adding WEB Content
E-3
Web Programming with the HTTP Server
An example implementation of AddWebFiles() is shown below. Note the addi-
tion of two more file creation calls. The first call to efs_createfile() creates the
file we declared in default.c as converted from the file default.htm. The second
call shows an alternate method of creating files. In this case, the HTML for the
file was typed directly into the source code. The third call creates a CGI file that
is actually a C function entrypoint. When a post is attempted to
sample.cgi
,
the function cgiSample() is called.
// Include our externally converted pages
#pragma DATA_SECTION(DEFAULT, ”HTMLDATA”);
#include ”default.c”
// Declare our 404.htm file content
static const char htm404[] =
”<html><body><h1>HTTP/1.0 404 Object Not Found</h1></body></html>”;
// Delare our CGI function entry point
static int cgiSample(int htmlSock, int ContentLength );
// Our function to initialize EFS with our WEB files
void AddWebFiles(void)
{
efs_createfile(”index.html”, DEFAULT_SIZE, DEFAULT);
efs_createfile(”404.htm”, strlen(htm404), (UINT8 *)htm404);
efs_createfile(”sample.cgi”, 0, (UINT8 *)cgiSample);
}
One the above code is run, the EFS system is ready for the HTTP server to
serve up the content. Note the inclusion of the #pragma to place the converted
WEB page into a memory section named HTMLDATA. This allows the page
to be place out of the way by specifying the section’s location in the linker com-
mand file.
E.1.4
Cleaning up HTML Files
Since the EFS system uses memory records to simulate file content from static
data, the system should be flushed or cleaned when the shutting down or re-
booting. In the example code, the function RemoveWebFiles() is called when
the EFS files are no longer required.
An implementation of RemoveWebFiles() that corresponds to the
AddWebFiles() function shown above would be as follows:
void RemoveWebFiles(void)
{
efs_destroyfile(”sample.cgi”);
efs_destroyfile(”404.htm”);
efs_destroyfile(”index.html”);
}