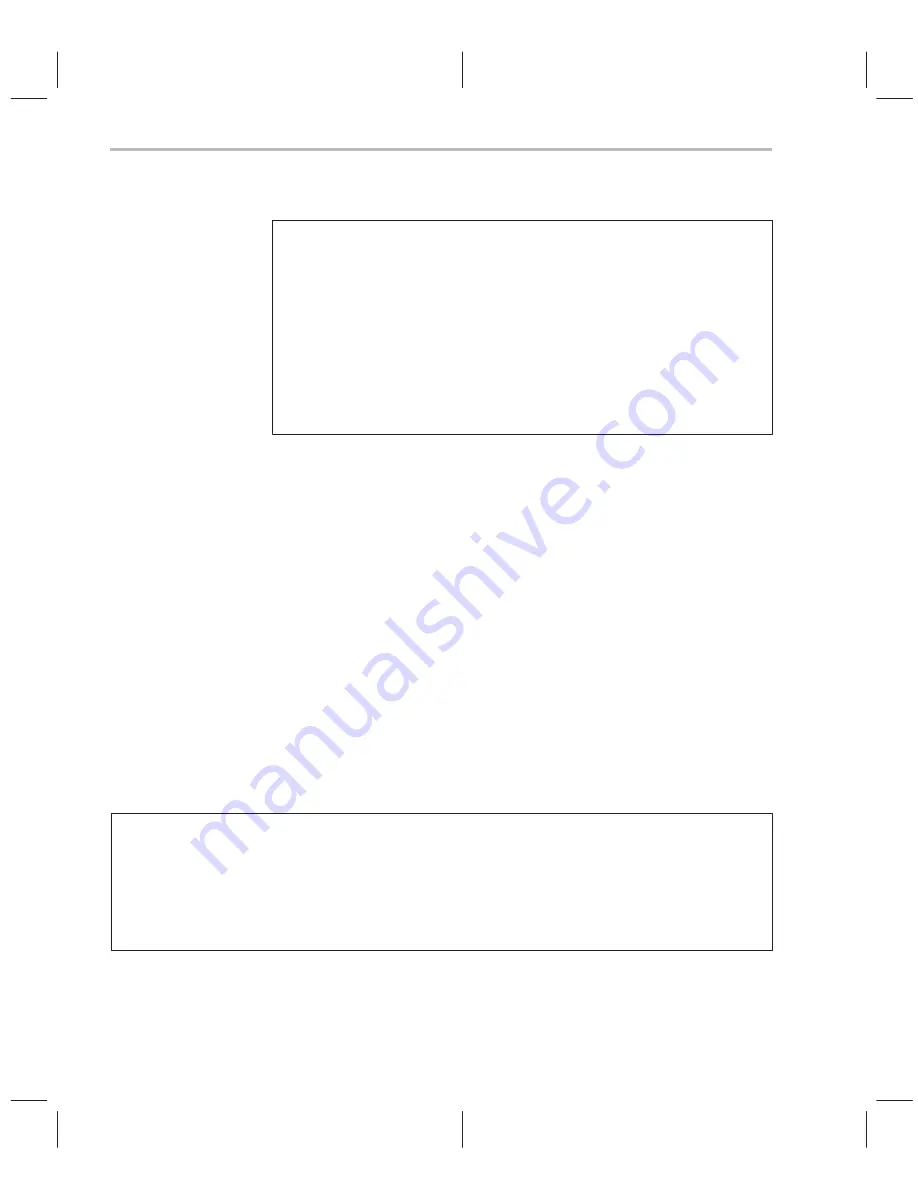
Using Word Access for Short Data and Doubleword Access for Floating-Point Data
6-20
Example 6–14. Floating-Point Dot Product C Code (Unrolled)
float dotp(float a[], float b[])
{
int i;
float sum0, sum1, sum;
sum0 = 0;
sum1 = 0;
for(i=0; i<100; i+=2){
sum0 += a[i] * b[i];
sum1 += a[i + 1] * b[i + 1];
}
sum = sum0 + sum1;
return(sum);
}
6.4.2
Translating C Code to Linear Assembly
The first step in optimizing your code is to translate the C code to linear assem-
bly.
6.4.2.1
Fixed-Point Dot Product
Example 6–15 shows the list of ’C6000 instructions that execute the unrolled
fixed-point dot product loop. Symbolic variable names are used instead of ac-
tual registers. Using symbolic names for data and pointers makes code easier
to write and allows the optimizer to allocate registers. However, you must use
the .reg assembly optimizer directive. See the
TMS320C6000 Optimizing
C/C++ Compiler User’s Guide for more information on writing linear assembly
code.
Example 6–15. Linear Assembly for Fixed-Point Dot Product Inner Loop with LDW
LDW
*a++,ai_i1
; load ai & a1 from memory
LDW
*b++,bi_i1
; load bi & b1 from memory
MPY
ai_i1,bi_i1,pi
; ai * bi
MPYH
ai_i1,bi_i1,pi1 ; ai+1 * bi+1
ADD
pi,sum0,sum0
; sum0 += (ai * bi)
ADD
pi1,sum1,sum1
; sum1 += (ai+1 * bi+1)
[cntr] SUB
cntr,1,cntr
; decrement loop counter
[cntr] B
LOOP
; branch to loop
The two load word (LDW) instructions load a[i], a[i+1], b[i], and b[i+1] on each
iteration.