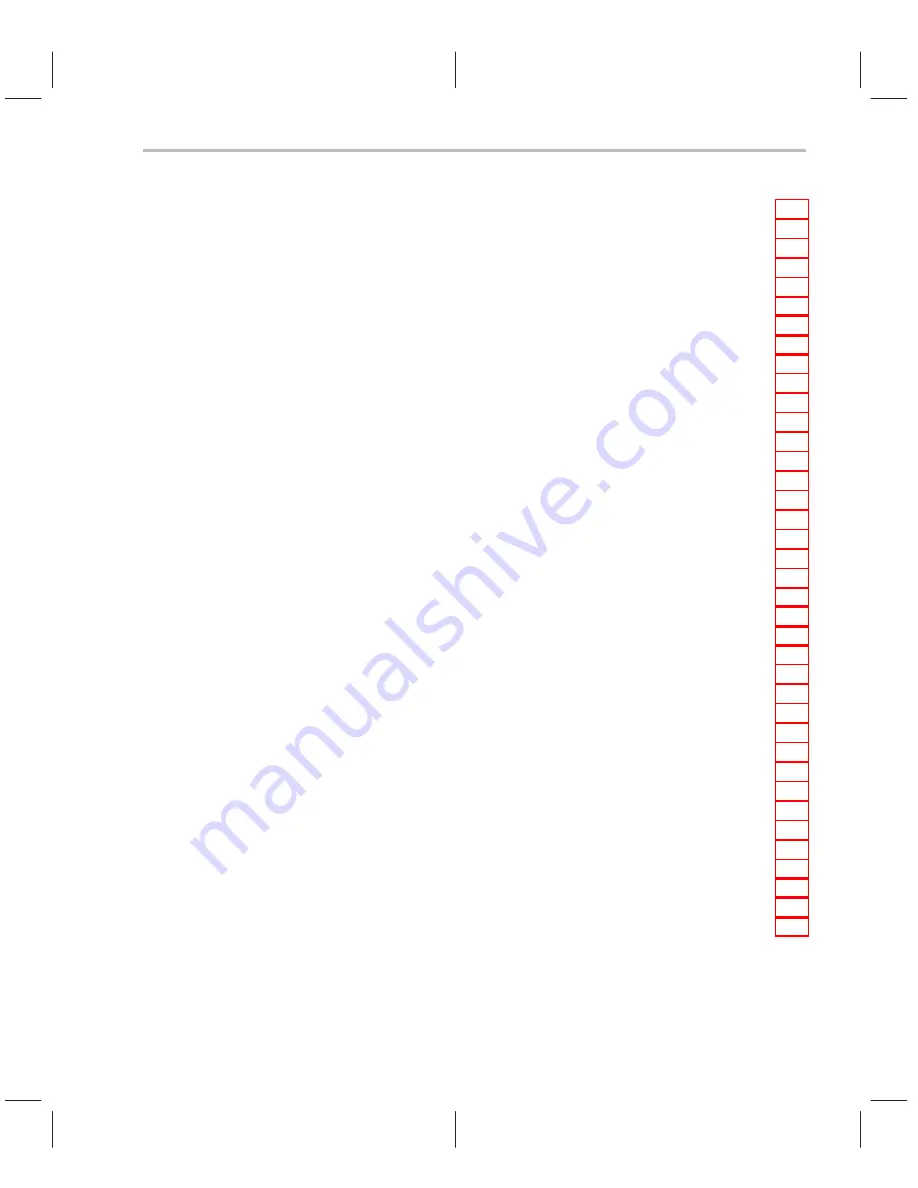
Contents
ix
Contents
6.4
Using Word Access for Short Data and Doubleword Access for
Floating-Point Data
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.4.1
Unrolled Dot Product C Code
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.4.2
Translating C Code to Linear Assembly
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.4.3
Drawing a Dependency Graph
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.4.4
Linear Assembly Resource Allocation
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.4.5
Final Assembly
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.4.6
Comparing Performance
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.5
Software Pipelining
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.5.1
Modulo Iteration Interval Scheduling
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.5.2
Using the Assembly Optimizer to Create Optimized Loops
. . . . . . . . . . . . . . .
6.5.3
Final Assembly
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.5.4
Comparing Performance
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.6
Modulo Scheduling of Multicycle Loops
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.6.1
Weighted Vector Sum C Code
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.6.2
Translating C Code to Linear Assembly
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.6.3
Determining the Minimum Iteration Interval
. . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.6.4
Drawing a Dependency Graph
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.6.5
Linear Assembly Resource Allocation
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.6.6
Modulo Iteration Interval Scheduling
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.6.7
Using the Assembly Optimizer for the Weighted Vector Sum
. . . . . . . . . . . . .
6.6.8
Final Assembly
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.7
Loop Carry Paths
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.7.1
IIR Filter C Code
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.7.2
Translating C Code to Linear Assembly (Inner Loop)
. . . . . . . . . . . . . . . . . . . .
6.7.3
Drawing a Dependency Graph
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.7.4
Determining the Minimum Iteration Interval
. . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.7.5
Linear Assembly Resource Allocation
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.7.6
Modulo Iteration Interval Scheduling
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.7.7
Using the Assembly Optimizer for the IIR Filter
. . . . . . . . . . . . . . . . . . . . . . . . .
6.7.8
Final Assembly
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.8
If-Then-Else Statements in a Loop
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.8.1
If-Then-Else C Code
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.8.2
Translating C Code to Linear Assembly
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.8.3
Drawing a Dependency Graph
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.8.4
Determining the Minimum Iteration Interval
. . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.8.5
Linear Assembly Resource Allocation
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.8.6
Final Assembly
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.8.7
Comparing Performance
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .