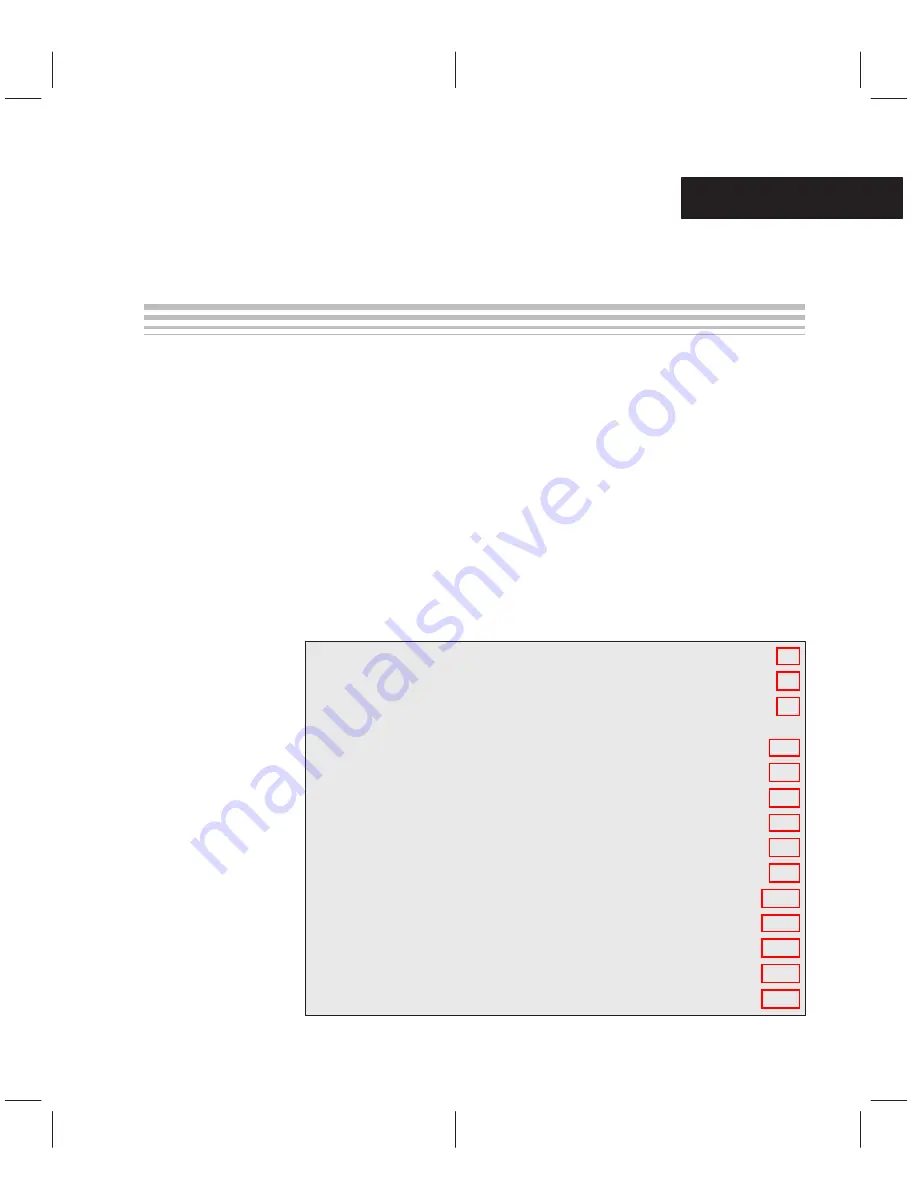
6-1
Optimizing Assembly Code
via Linear Assembly
This chapter describes methods that help you develop more efficient
assembly language programs, understand the code produced by the
assembly optimizer, and perform manual optimization.
This chapter encompasses phase 3 of the code development flow. After you
have developed and optimized your C code using the ’C6000 compiler, extract
the inefficient areas from your C code and rewrite them in linear assembly (as-
sembly code that has not been register-allocated and is unscheduled).
The assembly code shown in this chapter has been hand-optimized in order
to direct your attention to particular coding issues. The actual output from the
assembly optimizer may look different, depending on the version you are us-
ing.
Topic
Page
6.1
Assembly Code
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.2
Assembly Optimizer Options and Directives
. . . . . . . . . . . . . . . . . . . .
6.3
Writing Parallel Code
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.4
Using Word Access for Short Data and Doubleword Access
for Floating-Point Data
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.5
Software Pipelining
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.6
Modulo Scheduling of Multicycle Loops
. . . . . . . . . . . . . . . . . . . . . . .
6.7
Loop Carry Paths
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.8
If-Then-Else Statements in a Loop
. . . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.9
Loop Unrolling
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.10 Live-Too-Long Issues
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.11 Redundant Load Elimination
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.12 Memory Banks
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
6.13 Software Pipelining the Outer Loop
. . . . . . . . . . . . . . . . . . . . . . . . . . .
6.14 Outer Loop Conditionally Executed With Inner Loop
. . . . . . . . . . .
Chapter 6