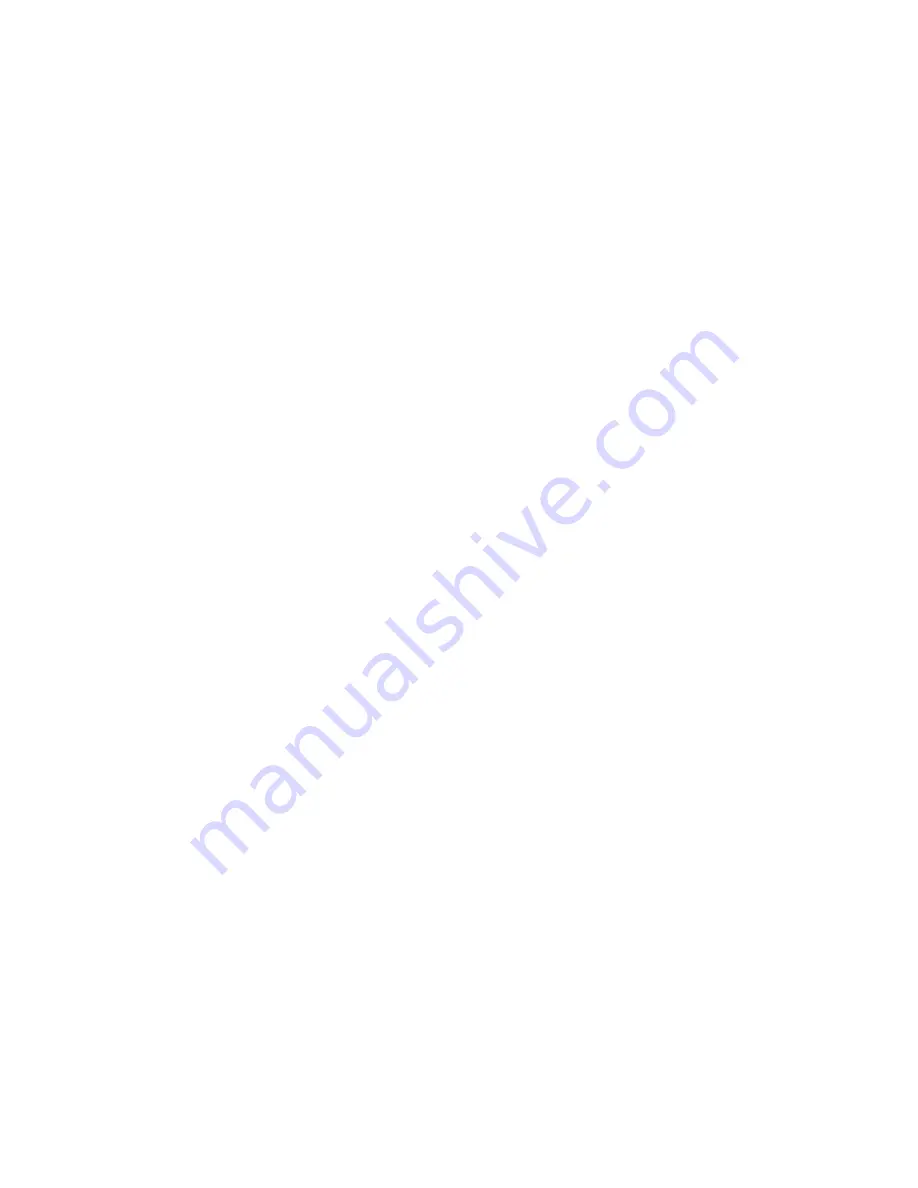
Preloading external media
201
Preloading MP3 and FLV files
To preload MP3 and FLV files, you can use the
setInterval()
function to create a “polling”
mechanism that checks the bytes loaded for a Sound or NetStream object at predetermined
intervals. To track the download progress of MP3 files, use the
Sound.getBytesLoaded()
and
Sound.getBytesTotal()
methods; to track the download progress of FLV files, use the
NetStream.bytesLoaded
and
NetStream.bytesTotal
properties.
The following code uses
setInterval()
to check the bytes loaded for a Sound or NetStream
object at predetermined intervals.
// Create a new Sound object to play the sound.
var songTrack = new Sound();
// Create the polling function that tracks download progress.
// This is the function that is "polled." It checks
// the download progress of the Sound object passed as a reference.
checkProgress = function (soundObj) {
var bytesLoaded = soundObj.getBytesLoaded();
var bytesTotal = soundObj.getBytesTotal();
var percentLoaded = Math.floor(bytesLoaded/bytesTotal * 100);
trace("%" + percent " loaded.");
}
// When the file has finished loading, clear the interval polling.
songTrack.onLoad = function () {
clearInterval(poll);
}
// Load streaming MP3 file and start calling checkProgress()
songTrack.loadSound("beethoven.mp3", true);
var poll = setInterval(checkProgress, 1000, songTrack);
You can use this same kind of polling technique to preload external FLV files. To get the total
bytes and current number of bytes loaded for an FLV file, use the
NetStream.bytesLoaded
and
NetStream.bytesTotal
properties.
Another way to preload FLV files is to use the
NetStream.setBufferTime()
method. This
method takes a single parameter that indicates the number of seconds of the FLV stream to
download before playback begins.
For more information, see
MovieClip.getBytesLoaded()
,
MovieClip.getBytesTotal()
,
NetStream.bytesLoaded
,
NetStream.bytesTotal
,
NetStream.setBufferTime()
,
setInterval()
,
Sound.getBytesLoaded()
, and
Sound.getBytesTotal()
in
Chapter 12,
“ActionScript Dictionary,” on page 205
.
Содержание FLASH MX 2004 - ACTIONSCRIPT
Страница 1: ...ActionScript Reference Guide...
Страница 8: ...8 Contents...
Страница 12: ......
Страница 24: ...24 Chapter 1 What s New in Flash MX 2004 ActionScript...
Страница 54: ...54 Chapter 2 ActionScript Basics...
Страница 80: ...80 Chapter 3 Writing and Debugging Scripts...
Страница 82: ......
Страница 110: ...110 Chapter 5 Creating Interaction with ActionScript...
Страница 112: ......
Страница 120: ...120 Chapter 6 Using the Built In Classes...
Страница 176: ......
Страница 192: ...192 Chapter 10 Working with External Data...
Страница 202: ...202 Chapter 11 Working with External Media...
Страница 204: ......
Страница 782: ...782 Chapter 12 ActionScript Dictionary...
Страница 793: ...Other keys 793 221 222 Key Key code...
Страница 794: ...794 Appendix C Keyboard Keys and Key Code Values...
Страница 798: ...798 Appendix D Writing Scripts for Earlier Versions of Flash Player...
Страница 806: ...806 Appendix E Object Oriented Programming with ActionScript 1...
Страница 816: ...816 Index...