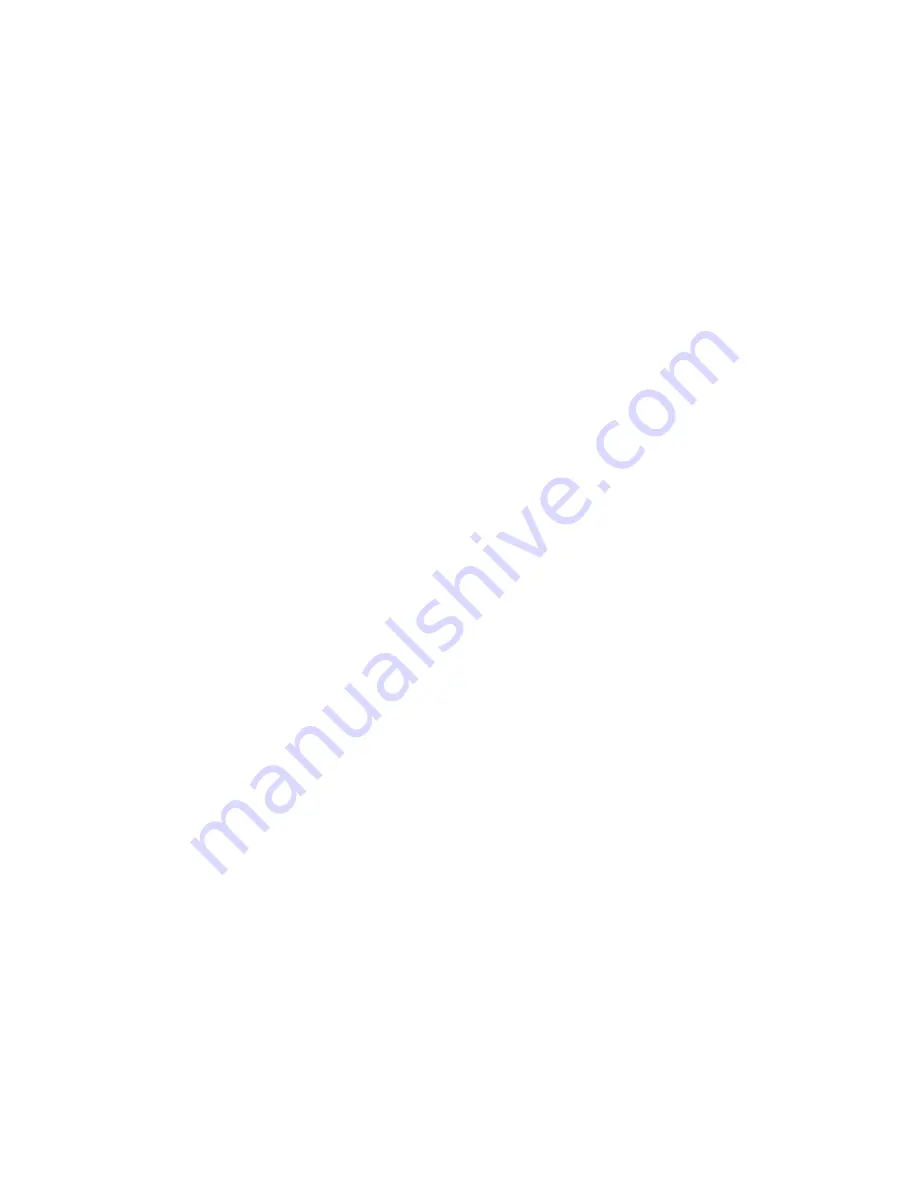
168
Chapter 9: Creating Classes with ActionScript 2.0
For example, the following code declares an interface named MyInterface that contains two
methods,
method_1()
and
method_2()
. The first method takes no parameters and has no return
type (specified as
Void
). The second method declaration takes a single parameter of type String,
and specifies a return type of Boolean.
interface MyInterface {
function method_1():Void;
function method_2(param:String):Boolean;
}
Interfaces cannot contain any variable declarations or assignments. Functions declared in an
interface cannot contain curly braces. For example, the following interface won’t compile.
interface BadInterface{
// Compiler error. Variable declarations not allowed in interfaces.
var illegalVar;
// Compiler error. Function bodies not allowed in interfaces.
function illegalMethod(){
}
}
The rules for naming interfaces and storing them in packages are the same as those for classes; see
“Creating and using classes” on page 161
and
“Using packages” on page 171
.
Interfaces as data types
Like a class, an interface defines a new data type. Any class that implements an interface can be
considered to be of the type defined by the interface. This is useful for determining if a given
object implements a given interface. For example, consider the following interface.
interface Movable {
function moveUp();
function moveDown();
}
Now consider the class Box that implements the Movable interface.
class Box implements Movable {
var x_pos, y_pos;
function moveUp() {
// method definition
}
function moveDown() {
// method definition
}
}
Then, in another script where you create an instance of the Box class, you could declare a variable
to be of the Movable type.
var newBox:Movable = new Box();
At runtime, in Flash Player 7 and later, you can cast an expression to an interface type. If the
expression is an object that implements the interface or has a superclass that implements the
interface, the object is returned. Otherwise,
null
is returned. This is useful if you want to make
sure that a particular object implements a certain interface.
Содержание FLASH MX 2004 - ACTIONSCRIPT
Страница 1: ...ActionScript Reference Guide...
Страница 8: ...8 Contents...
Страница 12: ......
Страница 24: ...24 Chapter 1 What s New in Flash MX 2004 ActionScript...
Страница 54: ...54 Chapter 2 ActionScript Basics...
Страница 80: ...80 Chapter 3 Writing and Debugging Scripts...
Страница 82: ......
Страница 110: ...110 Chapter 5 Creating Interaction with ActionScript...
Страница 112: ......
Страница 120: ...120 Chapter 6 Using the Built In Classes...
Страница 176: ......
Страница 192: ...192 Chapter 10 Working with External Data...
Страница 202: ...202 Chapter 11 Working with External Media...
Страница 204: ......
Страница 782: ...782 Chapter 12 ActionScript Dictionary...
Страница 793: ...Other keys 793 221 222 Key Key code...
Страница 794: ...794 Appendix C Keyboard Keys and Key Code Values...
Страница 798: ...798 Appendix D Writing Scripts for Earlier Versions of Flash Player...
Страница 806: ...806 Appendix E Object Oriented Programming with ActionScript 1...
Страница 816: ...816 Index...