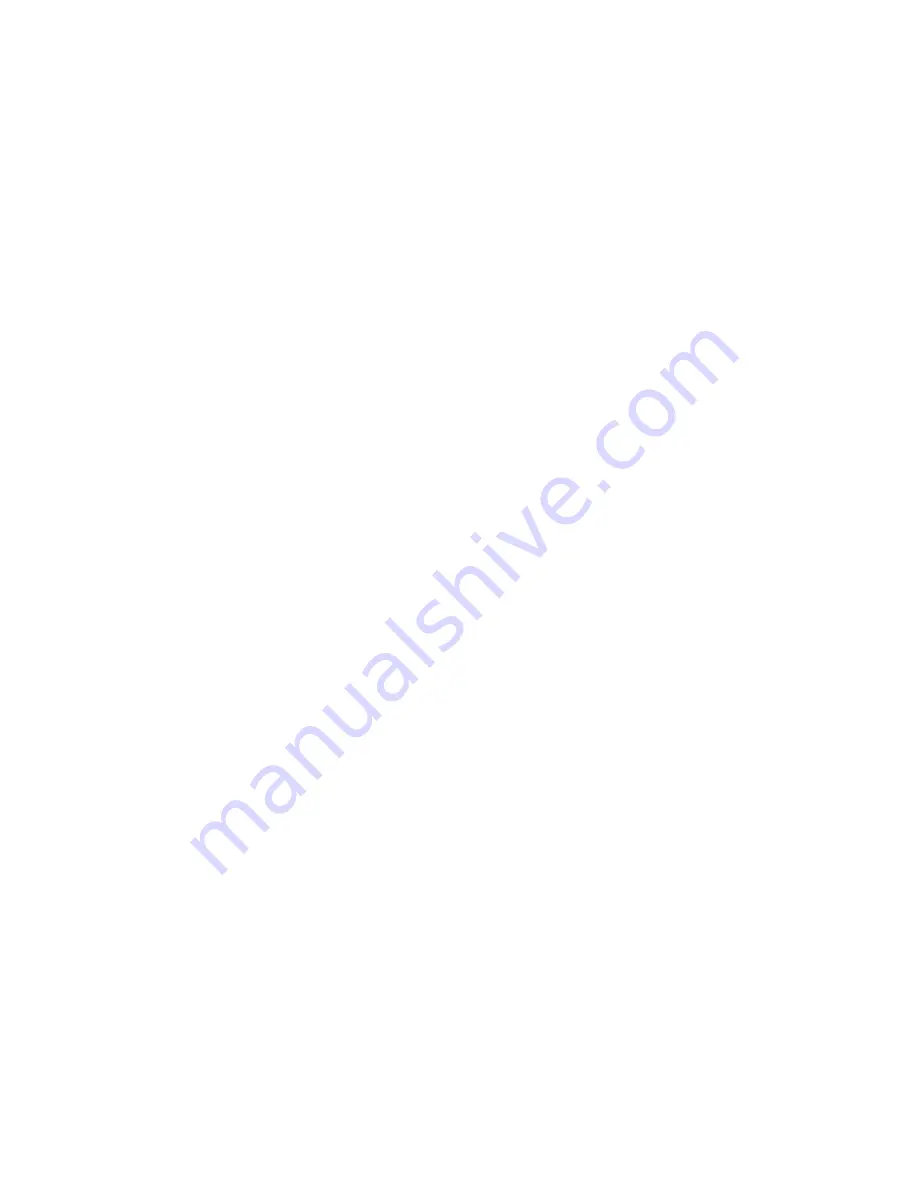
Creating and using classes
161
Creating and using classes
As discussed previously, a class consists of two parts: the
declaration
and the
body
. The class
declaration consists minimally of the
class
statement, followed by an identifier for the class
name, then left and right curly braces. Everything inside the braces is the class body.
class
className
{
// class body
}
You can define classes only in ActionScript (AS) files. For example, you can’t define a class on a
frame script in a FLA file. Also, the specified class name must match the name of the AS file that
contains it. For example, if you create a class called Shape, the AS file that contains the class
definition must be named Shape.as.
// In file Shape.as
class Shape {
// Shape class body
}
All AS class files that you create must be saved in one of the designated classpath directories—
directories where Flash looks for class definitions when compiling scripts. (See
“Understanding
the classpath” on page 169
.)
Class names must be identifiers; that is the first character must be a letter, underscore (
_
), or
dollar sign (
$
), and each subsequent character must be a letter, number, underscore, or dollar sign.
Also, the class name must be fully qualified within the file in which it is declared; that is, it must
reflect the directory in which it is stored. For example, to create a class named RequiredClass that
is stored in the myClasses/education/curriculum directory, you must declare the class in the
RequiredClass.as file like this:
class myClasses.education.curriculum.RequiredClass {
}
For this reason, it’s good practice to plan your directory structure before you begin creating
classes. Otherwise, if you decide to move class files after you create them, you will have to modify
the class declaration statements to reflect their new location.
Creating properties and methods
A class’s members consist of properties (variable declarations) and methods (function
declarations). You must declare all properties and methods inside the class body (the curly braces);
otherwise, an error will occur during compilation.
Any variable declared within a class, but outside a function, is a property of the class. For
example, the Person class discussed earlier has two properties,
age
and
name
, of type Number and
String, respectively.
class Person {
var age:Number;
var name:String;
}
Содержание FLASH MX 2004 - ACTIONSCRIPT
Страница 1: ...ActionScript Reference Guide...
Страница 8: ...8 Contents...
Страница 12: ......
Страница 24: ...24 Chapter 1 What s New in Flash MX 2004 ActionScript...
Страница 54: ...54 Chapter 2 ActionScript Basics...
Страница 80: ...80 Chapter 3 Writing and Debugging Scripts...
Страница 82: ......
Страница 110: ...110 Chapter 5 Creating Interaction with ActionScript...
Страница 112: ......
Страница 120: ...120 Chapter 6 Using the Built In Classes...
Страница 176: ......
Страница 192: ...192 Chapter 10 Working with External Data...
Страница 202: ...202 Chapter 11 Working with External Media...
Страница 204: ......
Страница 782: ...782 Chapter 12 ActionScript Dictionary...
Страница 793: ...Other keys 793 221 222 Key Key code...
Страница 794: ...794 Appendix C Keyboard Keys and Key Code Values...
Страница 798: ...798 Appendix D Writing Scripts for Earlier Versions of Flash Player...
Страница 806: ...806 Appendix E Object Oriented Programming with ActionScript 1...
Страница 816: ...816 Index...