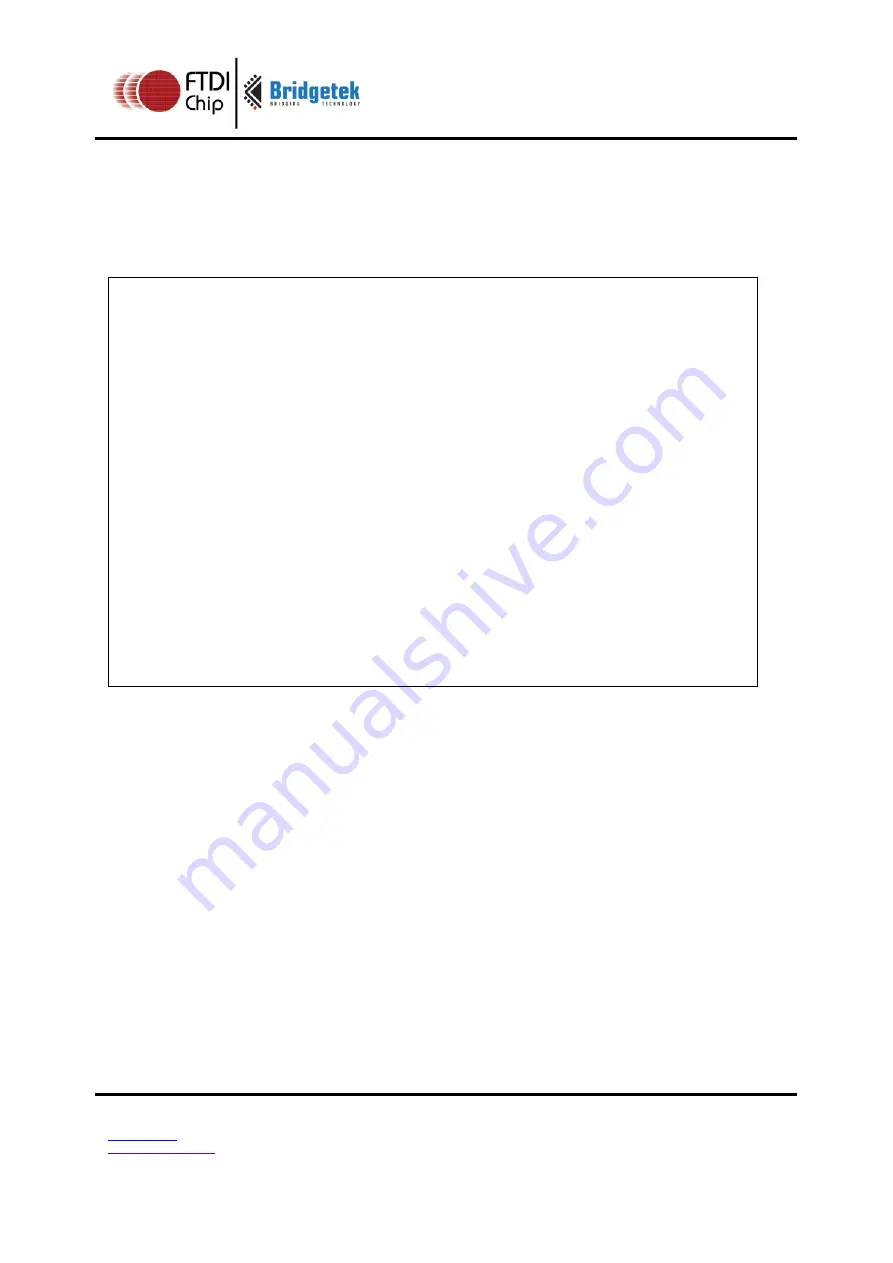
FT800 Series Programmer Guide
Version 2.1
Document Reference No.: BRT_000030 Clearance No.: BRT#037
30
Copyright © Bridgetek Limited
2.5.8
Screenshot
The code below demonstrates how to utilize the registers and RAM_SCREENSHOT to
capture the current screen with full pixel value. Each pixel is represented in 32 bits and
BGRA format. However, this process may cause the flicking and tearing effect.
#define SCREEN_WIDTH 480
#define SCREEN_HEIGHT 272
uint32 screenshot
[
SCREEN_WIDTH
*
SCREEN_HEIGHT
];
wr8
(
REG_SCREENSHOT_EN
,
1
);
for
(
int
ly
=
0
;
ly
<
SCREEN_HEIGHT
;
ly
++)
{
wr16
(
REG_SCREENSHOT_Y
,
ly
);
wr8
(
REG_SCREENSHOT_START
,
1
);
//Read 64 bit registers to see if it is busy
while
(
rd32
(
REG_SCREENSHOT_BUSY
)
|
rd32
(
REG_SCREENSHOT_BUSY
+
4
));
wr8
(
REG_SCREENSHOT_READ
,
1
);
for
(
int
lx
=
0
;
lx
<
SCREEN_WIDTH
;
lx
++)
{
//Read 32 bit pixel value from RAM_SCREENSHOT
//The pixel format is BGRA: Blue is in lowest address and Alpha
is in highest address
screenshot
[
ly
*
SCREEN_HEIGHT
+
lx
]
=
rd32
(
RAM_SCREENSHOT
+
lx
*
4
);
}
wr8
(
REG_SCREENSHOT_READ
,
0
);
}
wr8
(
REG_SCREENSHOT_EN
,
0
);
Code Snippet 12 Screenshot with full pixel value
2.5.9
Performance
The graphics engine has no frame buffer: it uses dynamic compositing to build up each
display line during scan out. Because of this, there is a finite amount of time available to
draw each line. This time depends on the scan out parameters (REG_PCLK and
REG_HCYCLE) but is never less than 2048 internal clock cycles.
Some performance limits:
The display list length must be less than 2048 instructions, because the
graphics engine fetches display list commands one per clock.
The graphics engine performance rending pixels is 4 pixels per clock, for any
line with 2048 display commands the total pixels performance drawn must be
less than 8192.
For some bitmap formats, the drawing rate is 1 pixel per clock. These are
TEXT8X8, TEXTVGA and PALETTED.
For bilinear filtered pixels, the drawing rate is reduced to ¼ pixel per clock.
Most bitmap formats draw at 1 pixel per clock, and the above formats
(TEXT8X8, TEXTVGA and PALETTED) draw at 1 pixel every 4 clocks.