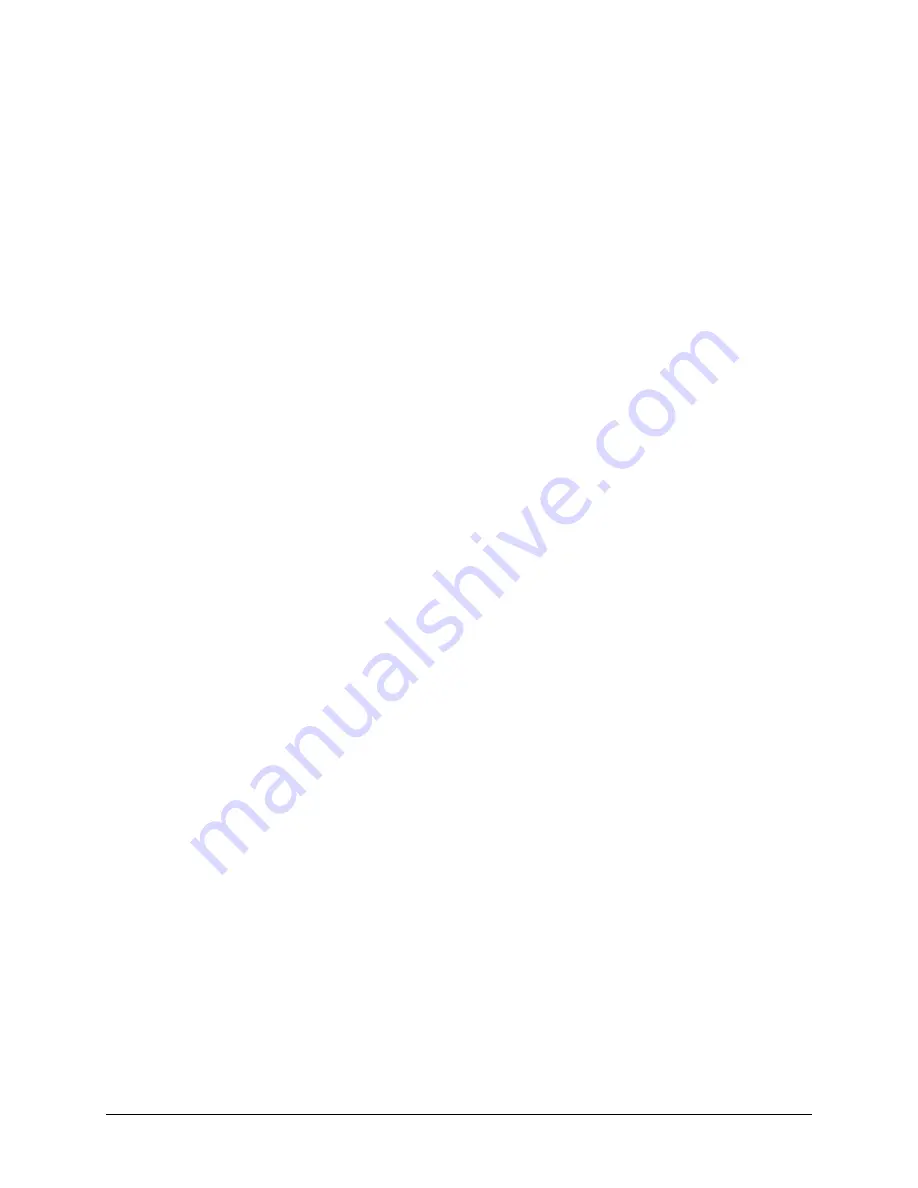
VTI Instruments Corporation
32
VM2710A Programming
/* We already have the high data; get the low and combine into 32 bits */
value = (temp << 16) | *data_lo;
/* Isolate the actual measurement part (remove the rest) */
int_value = value & 0x00ffffff;
/* Convert from an unsigned integer to a signed integer and sign extend */
if (int_value > 0xbfffff) int_value -= 0x1000000;
int_value = int_value - 0x00400000; /* Subtract the offset */
volt = (double)int_value * 3.0e-6; /* Multiply by the scale factor */
/* Gather the statistics: high, low and average */
accumulate = accu volt;
if (volt < voltmin)
{
voltmin = volt;
}
if (volt > voltmax)
{
voltmax = volt;
}
/* Keep track of how many readings we read */
i++;
}
/* Find out how much time it took to get the readings */
delta_time = Timer() - delta_time;
/* Compute the average */
accumulate = accumulate / i;
/* Print the information */
printf("Count = %d on %s at %s Elapsed time: %f seconds\n",
i, DateStr (), TimeStr (), delta_time);
printf("Average loop time: %f seconds per reading\n", delta_time/i);
printf("Average read rate: %f readings per second\n", i/delta_time);
printf("Readings Min: %f Average: %f Max: %f\n", voltmin, accumulate, voltmax);