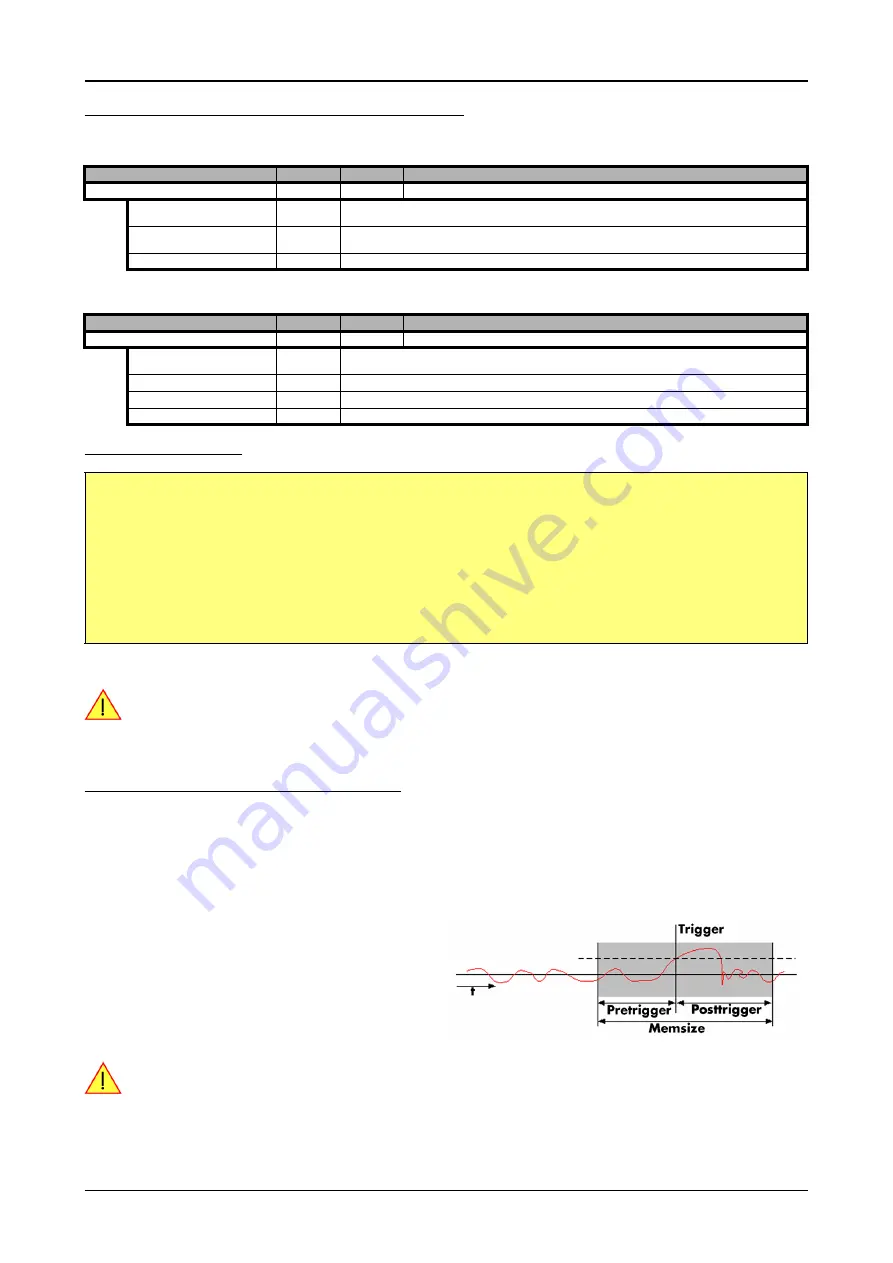
72
M3i.48xx / M3i.48xx-exp Manual
Standard Single acquisition mode
Acquisition modes
Commands and Status information for data transfer buffers.
As explained above the data transfer is performed with the same command and status registers like the card control. It is possible to send
commands for card control and data transfer at the same time as shown in the examples further below.
The data transfer can generate one of the following status information:
Example of data transfer
To keep the example simple it does no error checking. Please be sure to check for errors if using these command in real world programs!
Users should take care to explicitly send the M2CMD_DATA_STOPDMA command prior to invalidating the
buffer, to avoid crashes due to race conditions when using higher-latency data transportation layers, such
as to remote Ethernet devices.
Standard Single acquisition mode
The standard single mode is the easiest and mostly used mode to acquire analog data with a Spectrum acquisition card. In standard single
recording mode the card is working totally independent from the PC, after the card setup is done. The advantage of the Spectrum boards is
that regardless to the system usage the card will sample with equidistant time intervals.
The sampled and converted data is stored in the on-board memory and is held there for being read out after the acquisition. This mode allows
sampling at very high conversion rates without the need to transfer the data into the memory of the host system at high speed.
After the recording is done, the data can be read out by the user and is transferred via the bus into PC memory.
This standard recording mode is the most common mode for all an-
alog and digital acquisition and oscilloscope boards. The data is
written to a programmed amount of the on-board memory (mem-
size). That part of memory is used as a ring buffer, and recording
is done continuously until a trigger event is detected. After the trig-
ger event, a certain programmable amount of data is recorded
(post trigger) and then the recording finishes. Due to the continuous
ring buffer recording, there are also samples prior to the trigger
event in the memory (pretrigger).
When the card is started the pre trigger area is filled up with data first. While doing this the board’s trigger
detection is not armed. If you use a huge pre trigger size and a slow sample rate it can take up some time
after starting the board before a trigger event will be detected.
Register
Value
Direction
Description
SPC_M2CMD
100
write only
Executes a command for the card or data transfer
M2CMD_DATA_STARTDMA
10000h
Starts the DMA transfer for an already defined buffer. In acquisition mode it may be that the card hasn’t received a
trigger yet, in that case the transfer start is delayed until the card receives the trigger event
M2CMD_DATA_WAITDMA
20000h
Waits until the data transfer has ended or until at least the amount of bytes defined by notify size are available. This
wait function also takes the timeout parameter described above into account.
M2CMD_DATA_STOPDMA
40000h
Stops a running DMA transfer. Data is invalid afterwards.
Register
Value
Direction
Description
SPC_M2STATUS
110
read only
Reads out the current status information
M2STAT_DATA_BLOCKREADY
100h
The next data block as defined in the notify size is available. It is at least the amount of data available but it also can
be more data.
M2STAT_DATA_END
200h
The data transfer has completed. This status information will only occur if the notify size is set to zero.
M2STAT_DATA_OVERRUN
400h
The data transfer had on overrun (acquisition) or underrun (replay) while doing FIFO transfer.
M2STAT_DATA_ERROR
800h
An internal error occurred while doing data transfer.
void* pvData = (void*) new int8[1024];
// transfer data from PC memory to card memory
spcm_dwDefTransfer_i64 (hDrv, SPCM_BUF_DATA, SPCM_DIR_PCTOCARD , 0, pvData, 0, 1024);
spcm_dwSetParam_i32 (hDrv, SPC_M2CMD, M2CMD_DATA_STARTDMA | M2CMD_DATA_WAITDMA);
// transfer the same data back to PC memory
spcm_dwDefTransfer_i64 (hDrv, SPCM_BUF_DATA, SPCM_DIR_CARDTOPC , 0, pvData, 0, 1024);
spcm_dwSetParam_i32 (hDrv, SPC_M2CMD, M2CMD_DATA_STARTDMA | M2CMD_DATA_WAITDMA);
// explicitely stop DMA tranfer prior to invalidating buffer
spcm_dwSetParam_i32 (hDrv, SPC_M2CMD, M2CMD_DATA_STOPDMA);
delete [] (int8*) pvData;