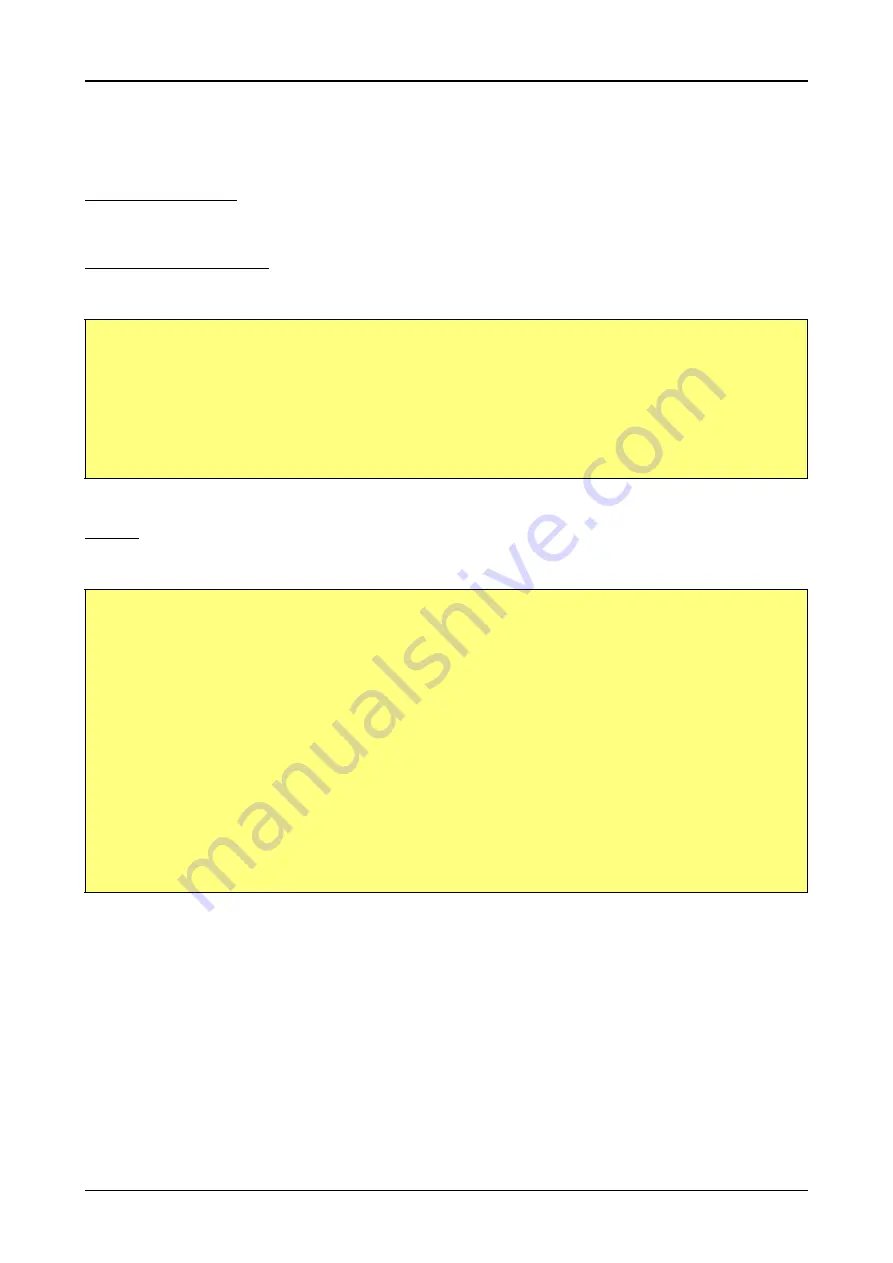
Continuous memory for increased data transfer rate
(c) Spectrum GmbH
135
As memory allocation is organized completely different compared to Windows the amount of data that is available for a continuous DMA
buffer is unfortunately limited to a maximum of 8 MByte. On most systems it will even be only 4 MBytes.
Usage of the buffer
The usage of the continuous memory is very simple. It is just necessary to read the start address of the continuous memory from the driver and
use this address instead of a self allocated user buffer for data transfer.
Function spcm_dwGetContBuf
This function reads out the internal continuous memory buffer (in bytes) if one has been allocated. If no buffer has been allocated the function
returns a size of zero and a NULL pointer.
Please note that it is not possible to free the continuous memory for the user application.
Example
The following example shows a simple standard single mode data acquisition setup (for a card with 12/14/16 bit per resolution one sample
equals 2 bytes) with the read out of data afterwards. To keep this example simple there is no error checking implemented.
uint32 _stdcall spcm_dwGetContBuf_i64 ( // Return value is an error code
drv_handle hDevice, // handle to an already opened device
uint32 dwBufType, // type of the buffer to read as listed above under SPCM_BUF_XXXX
void** ppvDataBuffer, // address of available data buffer
uint64* pqwContBufLen); // length of available continuous buffer
uint32 _stdcall spcm_dwGetContBuf_i64m (// Return value is an error code
drv_handle hDevice, // handle to an already opened device
uint32 dwBufType, // type of the buffer to read as listed above under SPCM_BUF_XXXX
void** ppvDataBuffer, // address of available data buffer
uint32* pdwContBufLenH, // high part of length of available continuous buffer
uint32* pdwContBufLenL); // low part of length of available continuous buffer
int32 lMemsize = 16384; // recording length is set to 16 kSamples
spcm_dwSetParam_i32 (hDrv, SPC_CHENABLE, CHANNEL0); // only one channel activated
spcm_dwSetParam_i32 (hDrv, SPC_CARDMODE, SPC_REC_STD_SINGLE); // set the standard single recording mode
spcm_dwSetParam_i32 (hDrv, SPC_MEMSIZE, lMemsize); // recording length in samples
spcm_dwSetParam_i32 (hDrv, SPC_POSTTRIGGER, 8192); // samples to acquire after trigger = 8k
// now we start the acquisition and wait for the interrupt that signalizes the end
spcm_dwSetParam_i32 (hDrv, SPC_M2CMD, M2CMD_CARD_START | M2CMD_CARD_ENABLETRIGGER | M2CMD_CARD_WAITREADY);
// we now try to use a continuous buffer for data transfer or allocate our own buffer in case there’s none
spcm_dwGetContBuf_i64 (hDrv, SPCM_BUF_DATA, &pvData, &qwContBufLen);
if (qwContBufLen < (2 * lMemsize))
pvData = new int16[lMemsize];
// read out the data
spcm_dwDefTransfer_i64 (hDrv, SPCM_BUF_DATA, SPCM_DIR_CARDTOPC , 0, pvData, 0, 2 * lMemsize);
spcm_dwSetParam_i32 (hDrv, SPC_M2CMD, M2CMD_DATA_STARTDMA | M2CMD_DATA_WAITDMA);
// ... Use the data here for analysis/calculation/storage
// delete our own buffer in case we have created one
if (qwContBufLen < (2 * lMemsize))
delete[] pvData;