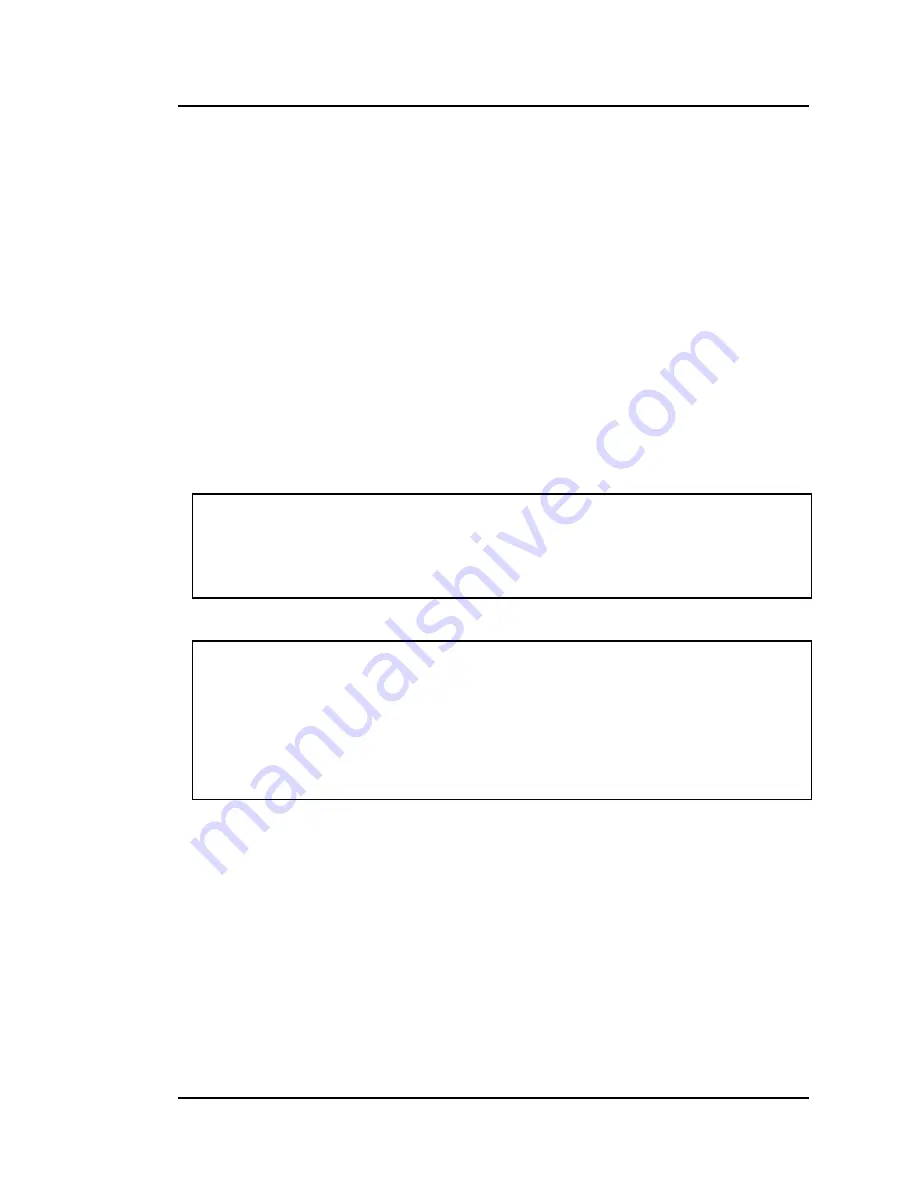
BASIC Moves Development Studio
06/2005
Danaher Motion
38 Rev
E
M-SS-005-03l
Pare
ntheses are not used in function
CALL
if no parameters are passed.
Parameters (either scalar or array) passed to the function are used within the
code of the function. Declare variable names and types of parameters in the
declaration line of the function. Parameters can be passed by reference or by
value. The default is by reference.
Arrays can only be passed by reference. Trying to pass a whole array by
value results in a translation error. On the other hand, array elements can be
passed by reference and by value.
To set up the return value, assign the return value to a virtual local variable
with the same name as the function somewhere within the code of the
function. This local variable is declared automatically during function
declaration and the function uses it to obtain the return value.
There is no explicit limit on the number of functions allowed in a task. All
functions must be located following the main program and must be contained
wholly outside of the main program.
MC-BASIC automatically checks the type of compliance between the
function declaration and the function call. Any mismatch (in number of
parameters, in parameters and returned value types) causes an error during
program loading. Automatic type casting applies only for long and double
returned values and “by value” parameters. For example:
Function LongReturnFunc(…) As Long
LongReturnFunc = “String” -> type mismatch in returned value
End Function
Function LongReturnFunc(…) As Long
LongReturnFunc = 43.7 -> valid type casting in returned value
End Function
A function can be recursive (can call itself). The following example defines a
recursive function to calculate the value of
N
:
FUNCTION
Factorial (ByVal N As Long) As Double
'By declaring N to be long, this truncates floating point numbers
'to integers
'The function returns a Double value
If N < 3 then 'This statement stops the recursion
Factorial = N '0!=0; 1!=1' 2!=2
Else
Factorial=N * Factorial(N-1) 'Recursive statement
End If
END FUNCTION
When writing a recursive function, you must have an
IF
statement to force
the function to return without the recursive call being executed. Otherwise,
the function never returns once it is called.
2. 4
L
IBRARIES
Some applications repeatedly use the same subroutines and functions. Such
subroutines and functions can be gathered into a library file. The library file
can be imported to another task with
IMPORT
on the
.
lib
file at the beginning
of the task. Then, each public subroutine and function defined in the
imported library file can be called within the importing task.