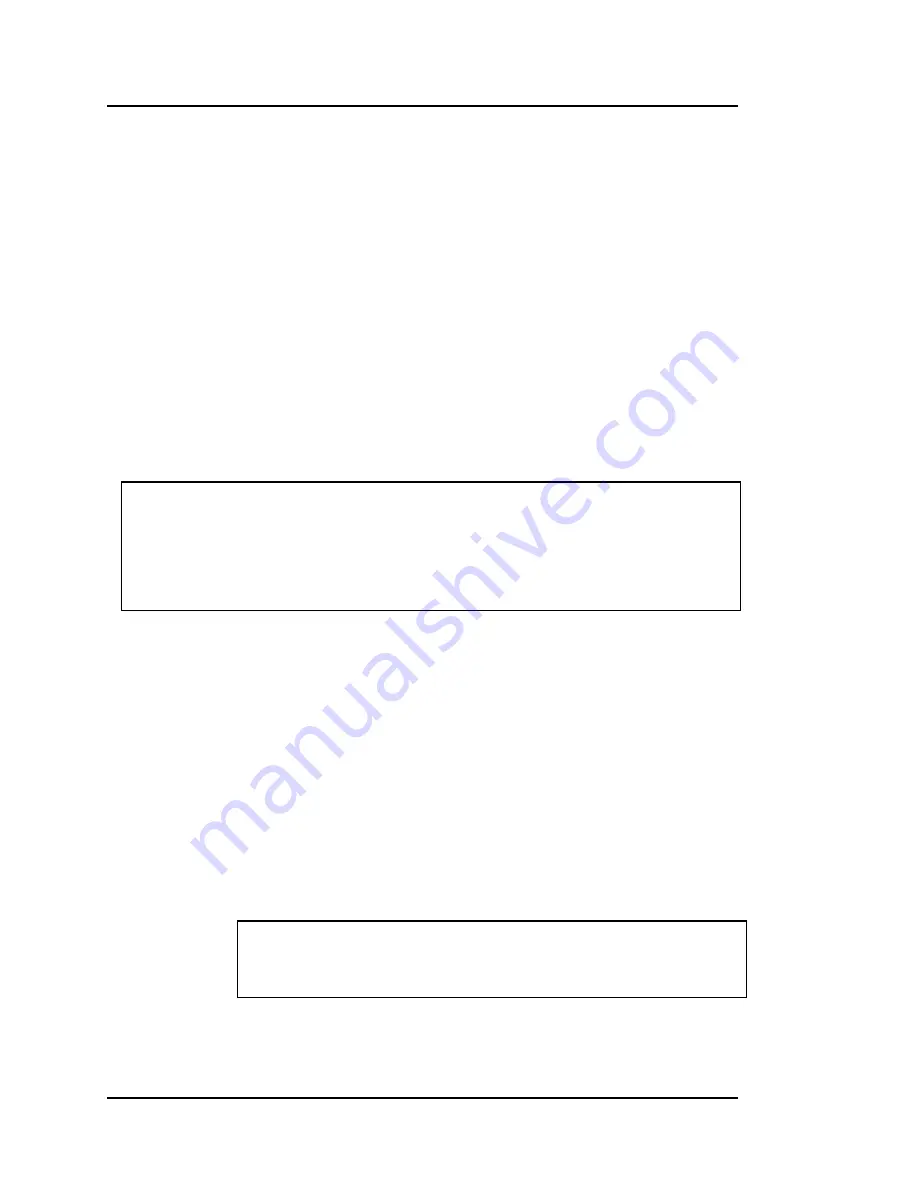
Danaher Motion
06/2005
BASIC Moves Development Studio
M-SS-005-03 Rev
E
37
When a variable is passed by reference (whether the variable is local to the
task or global), the address of the variable is passed to the subroutine, which
changes the value of the original variable (if the code of the subroutine is
written to do this).
When a variable is passed by value (ByVal) a local copy of the value of the
variable is passed to the subroutine, and the subroutine cannot change the
value of the original variable.
There is no explicit limit on the number of subroutines allowed in a task. All
subroutines must be located following the main program and must be
contained wholly outside the main program. Subroutines can only be called
from within the task where they reside. Subroutines may be recursive (can
call itself). Use
CALL
to execute a subroutine with the following syntax:
CALL
<subroutine_name>{(<par_1>{, …<par_n>})}
Pare
ntheses are not used in subroutine
CALL
if no parameters are passed.
MC-BASIC automatically checks the type of compliance between the
subroutine declaration and the subroutine call. Any mismatch (in number of
parameters or parameters types) causes an error during program loading.
Automatic type casting applies only for “by value” long and double
parameters.
SUB
MySub(RefPar as long, byval ValPar as double)
…
END SUB
CALL
MySub(LongVar, “String”) -> type mismatch in second parameter
CALL
MySub(LongVar) -> wrong number of parameters
CALL
MySub(LongVar, 2) -> a valid type casting for a by-value parameter
CALL
MySub(DoubleVar, 2.2) -> invalid type casting for a by-ref parameter
2.3.3. User-Defined
Functions
MC BASIC allows the definition of user functions to be used in programs in
the same manner as using BASIC's pre-defined functions. User-defined
functions are composed with multiple lines and may be recursive (can call
itself). Unlike BASIC's system functions, the scope of user-defined functions
is limited to the task in which it is defined.
Functions are different from subroutines in one respect. Functions always
return a value to the task that called the function. Otherwise, functions and
subroutines use the same syntax and follow the same rules of application
and behavior.
Because functions return a value, function calls should be treated as
expressions. Therefore, function called can
be combined within print
commands, assignment statements, mathematical operations and conditions
of flow control statements. They can also be passed as by-value parameters
of system or user-defined functions and subroutines.
<
function_name
>{(<
par_1
>{, …<
par_
n>})}
<
variable_name
> = <
function_name
>{(<
par_1
>{, …<
par_
n>})}
IF
<
function_name
>{(<
par_1
>{, …<
par_
n>})} > 10 THEN
?
LOG
( <
function_name
>{(<
par_1
>{, …<
par_
n>})} )