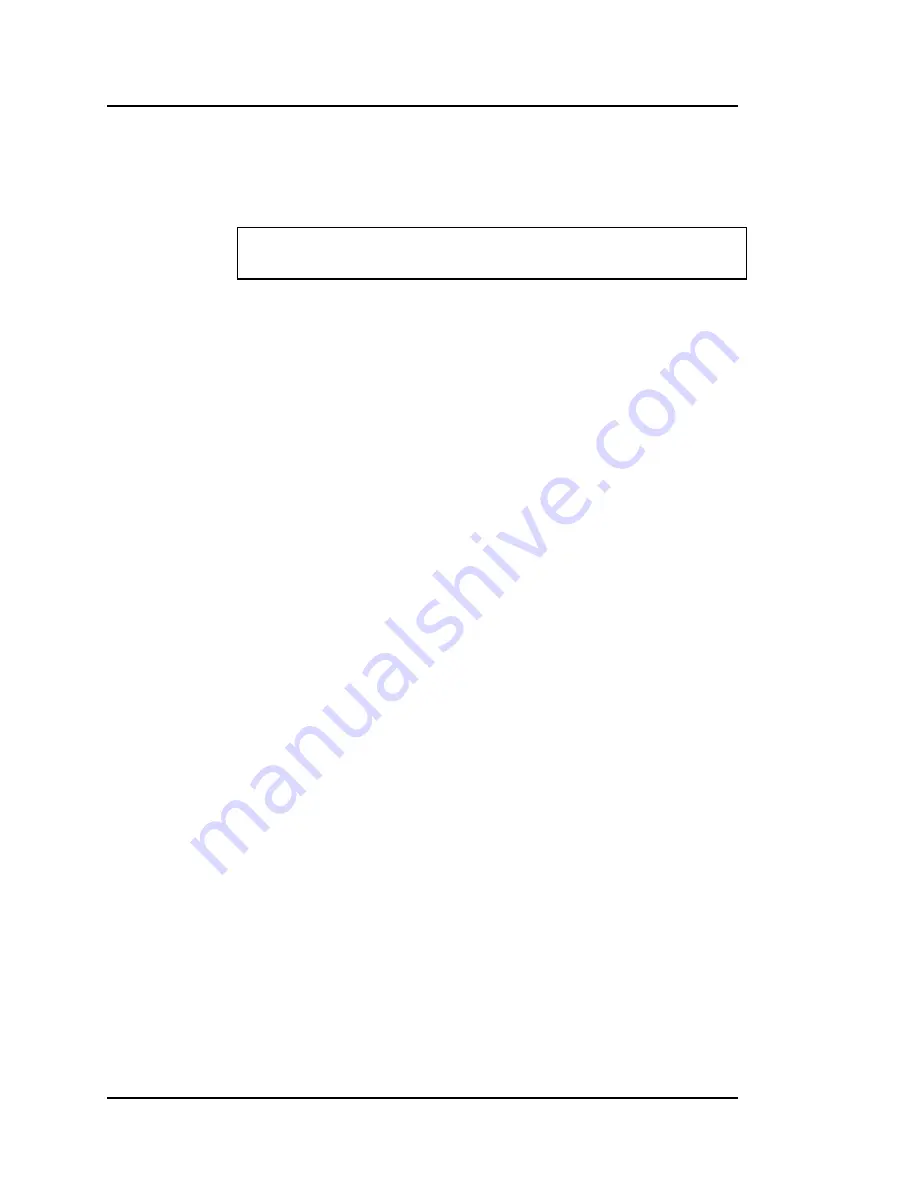
Danaher Motion
06/2005
BASIC Moves Development Studio
M-SS-005-03 Rev
E
23
Bit-wise expressions differ from logical expressions in that there may be
many results from a single operation. Bitwise operations are frequently used
to mask I/O bits. For example, the standard SERVO
STAR
MC inputs can be
operated on with BAnd (Bitwise And) to mask off some of the inputs. The
following example masks off all bits of the digital outputs, except the
rightmost four:
Dim Shared MaskedBits As Long
MaskedBits = System.Dout Band 0xF
‘Mask all but rightmost four bits
All bitwise operations produce 32-bit result from two 32-bit numbers. Bitwise
operations are really 32 independent, bit-by-bit operations. For example:
15
(Binary 1111)
BAnd 5
(Binary 101)
is 5
(Binary 101)
BNot 15 is 0xFFFFFFF0.
2.2.9. Math
Functions
MC-BASIC provides a large assortment of mathematical functions. All take
either numeric type as input (Double and Long) and all but Int and Sgn
produce Double results. Int and Sgn produce Long results. For detailed
information on any of the math functions (including examples), refer to the
SERVOSTAR
®
MC Reference Manual
.
Abs(x)
Returns the absolute value of X.
Acos(x)
Returns the arc cosine of X. X is assumed to be in radians.
Asin(x)
Returns the arc sine of X. X is assumed to be in radians.
Atan2(Y, X)
Returns the inverse tangent of Y/X in radians. The range of the result is
between ±PI radians (±180°). Atan2 is an improvement over ATN(Y/X)
in that it works correctly if X is zero, and if X<0.
Atn(X)
Returns the inverse tangent of X in radians. The range of the result is
between
±
PI/2 radians (
±
90
°
).
Cos(X)
Returns the cosine of X. X is assumed to be in radians.
Exp(X)
Returns ex.
Int(X)
Returns the largest long value less than or equal to a numeric
expression (for example, ?Int(12.5) returns 12
?Int(-12.5) returns -13)
Log(X)
Returns the natural logarithm of X. X must be > 0. If you want the base-
10 log, use Y = LOG(X)/LOG(10).
Sgn(X)
Returns the arithmetic sign of X. If X > 0, return 1. If X < 0, return -1. If X
= 0, return 0.
Sin(X)
Returns the sine of X. X is assumed to be in radians.
Sqrt(X)
Returns the positive square root of X. X must be >= 0.
Tan(X)
Returns the tangent of X. X is assumed to be in radians.
Round(X)
Returns the integer nearest to X. If X falls exactly between two integers
(for example, 1.5), return the even integer. So 1.5 and 2.5 round to 2.