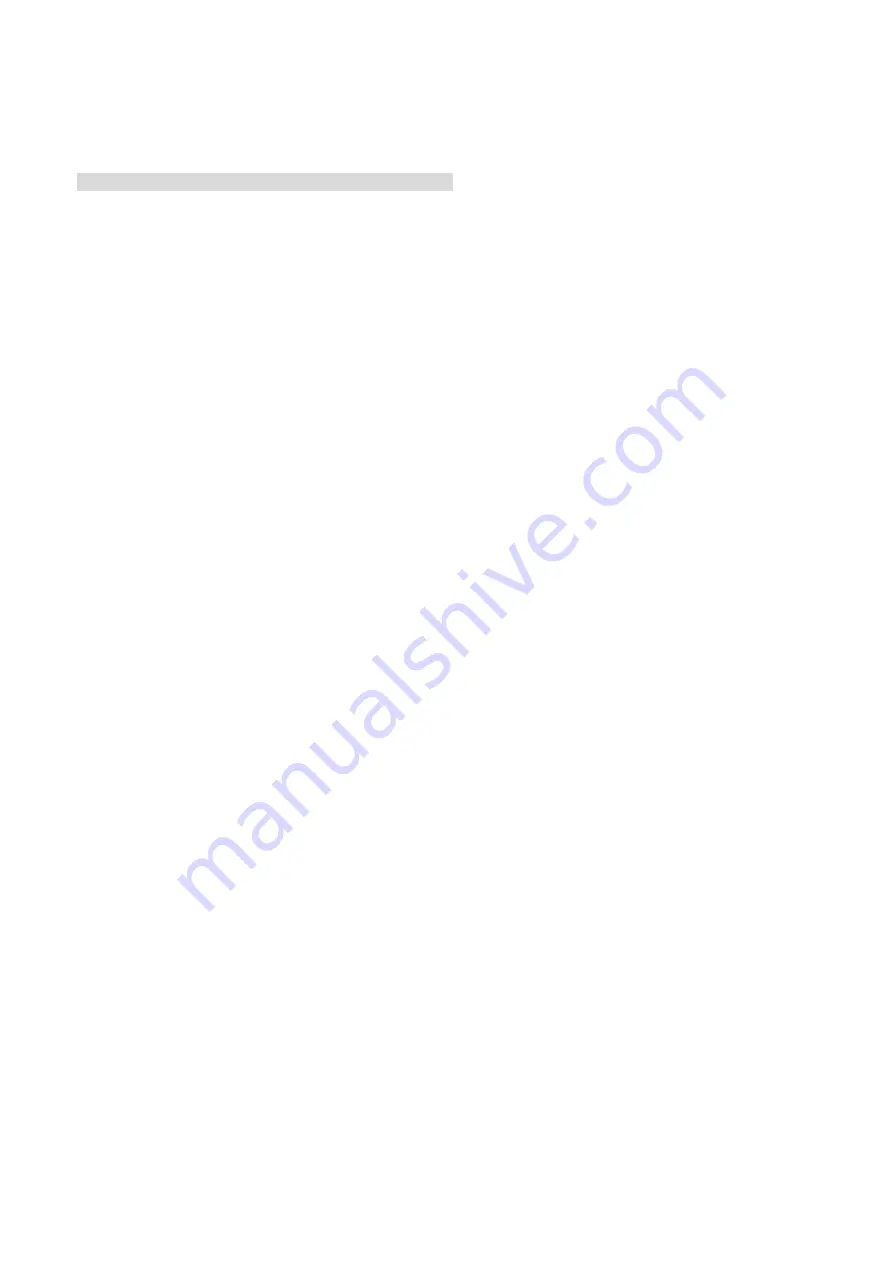
Temposonics
®
R-Series Powerlink V2
Operation Manual
I 45 I
8.5 Program executed by PLC cyclically
This program implements the state machine and accesses the
LossSoC threshold of R-Series Powerlink (source code below).
Source code “SdoAccessCyclic.c”
/********************************************************************
* COPYRIGHT --
********************************************************************
* Program: SdoAccess
* File: SdoAccessCyclic.c
* Author: SSchumacher
* Created: November 18, 2014
********************************************************************
* Implementation of program SdoAccess
********************************************************************/
#include <bur/plctypes.h>
#ifdef _DEFAULT_INCLUDES
#include <AsDefault.h>
#endif
void
_CYCLIC SdoAccessCyclic(
void
)
{
if
(fbSdoRead.status != ERR_FUB_BUSY && fbSdoWrite.status != ERR_FUB_BUSY)
{
//currently there is no SDO operation in progress
//initiate SDO operation
switch
(iState)
{
case
STATE_READ_INITIAL_VALUE:
fbSdoRead.pDevice
=
“SS1.IF1”;
//interface sensor is connected to
fbSdoRead.node
=
1;
//node id of sensor
fbSdoRead.index
=
0x1c0b;
//index of LossSoC Threshold
fbSdoRead.subindex
=
3;
//subindex of LossSoC Threshold
fbSdoRead.pData
=
&u32LossSocThrInitialValue;
//variable to store value to
fbSdoRead.datalen
=
sizeof(u32LossSocThrInitialValue);
//size of the variable to store value to
fbSdoRead.enable
=
1;
//enable the read operation
fbSdoWrite.enable
=
0;
//disable write operation
//go
to
next
step
+;
break
;
case
STATE_WRITE_NEW_VALUE:
fbSdoWrite.pDevice
=
“SS1.IF1”;
//interface sensor is connected to
fbSdoWrite.node
=
1;
//node id of sensor
fbSdoWrite.index
=
0x1c0b;
//index of LossSoC Threshold
fbSdoWrite.subindex
=
3;
//subindex of LossSoC Threshold
fbSdoWrite.pData
=
&u32LossSocThrToSet;
//variable containing value to set
fbSdoWrite.datalen
=
sizeof(u32LossSocThrToSet);
//size of the variable containing value to set
fbSdoWrite.enable
=
1;
//enable write operation
fbSdoRead.enable
=
0;
//disable read operation
//go to next step
+;
break
;
case
STATE_READ_CHANGED_VALUE:
fbSdoRead.pDevice
=
“SS1.IF1”;
//interface sensor is connected to
fbSdoRead.node
=
1;
//node id of sensor
fbSdoRead.index
=
0x1c0b;
//index of LossSoC Threshold
fbSdoRead.subindex
=
3;
//subindex of LossSoC Threshold
fbSdoRead.pData
=
&u32LossSocThrChangedValue;
//variable to store value to
fbSdoRead.datalen
=
sizeof(u32LossSocThrChangedValue);
//size of the variable to store value to
fbSdoRead.enable
=
1;
//enable the read operation
fbSdoWrite.enable
=
0;
//disable write operation
//go to next step
+;
break
;
default
:
fbSdoRead.enable
=
0;
//disable read operation
fbSdoWrite.enable
=
0;
//disable write operation
break
;
}
}
//execute SDO read if enabled
EplSDORead(&fbSdoRead);
//execute SDO write if enabled
EplSDOWrite(&fbSdoWrite);
}