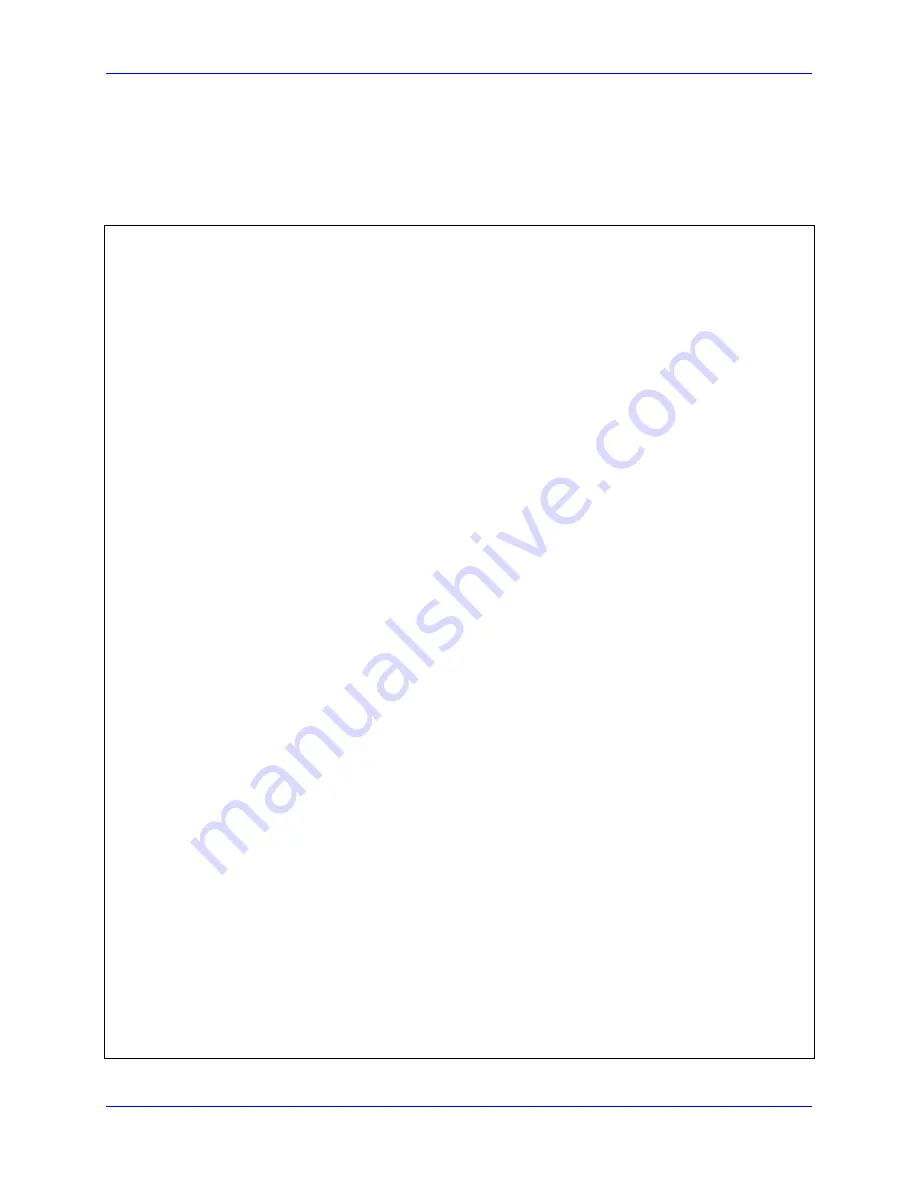
ACC-72EX User Manual
Software setup
90
C Programming Access to ACC-72EX Structures
One can use the following header file full of functions to read from and write to the aforementioned
Acc72EX[
i
]
structures from a C program. The input argument CardIndex is
i
and ArrayIndex is
j
as
above. Use the “Get” functions to retrieve the structure values; use the “Set” functions to write to the
structures. In the “Set” functions, the Input argument is the value to which to set the structure.
int
Acc72EX_GetIdata32(
unsigned
int
CardIndex,
unsigned
int
ArrayIndex);
unsigned
int
Acc72EX_GetUdata32(
unsigned
int
CardIndex,
unsigned
int
ArrayIndex);
short
Acc72EX_GetIdata16(
unsigned
int
CardIndex,
unsigned
int
ArrayIndex);
char
Acc72EX_GetData8(
unsigned
int
CardIndex,
unsigned
int
ArrayIndex);
unsigned
short
Acc72EX_GetUdata16(
unsigned
int
CardIndex,
unsigned
int
ArrayIndex);
void
Acc72EX_SetIdata16(
unsigned
int
CardIndex,
unsigned
int
ArrayIndex,
short
Input);
void
Acc72EX_SetUdata16(
unsigned
int
CardIndex,
unsigned
int
ArrayIndex,
unsigned
short
Input);
void
Acc72EX_SetIdata32(
unsigned
int
CardIndex,
unsigned
int
ArrayIndex,
int
Input);
void
Acc72EX_SetUdata32(
unsigned
int
CardIndex,
unsigned
int
ArrayIndex,
unsigned
int
Input);
void
Acc72EX_SetData8(
unsigned
int
CardIndex,
unsigned
int
ArrayIndex,
char
Input);
short
Acc72EX_GetIdata16(
unsigned
int
CardIndex,
unsigned
int
ArrayIndex)
{
unsigned
int
*myptr = (
unsigned
int
*)piom + (DPR CardIndex *
0x100000
) /
4
;
return
(
short
)((myptr[ArrayIndex] <<
8
) >>
16
);
}
unsigned
short
Acc72EX_GetUdata16(
unsigned
int
CardIndex,
unsigned
int
ArrayIndex)
{
unsigned
int
*myptr = (
unsigned
int
*)piom + (DPR CardIndex *
0x100000
) /
4
;
return
(
unsigned
short
)((myptr[ArrayIndex] <<
8
) >>
16
);
}
char
Acc72EX_GetData8(
unsigned
int
CardIndex,
unsigned
int
ArrayIndex)
{
unsigned
int
*myptr = (
unsigned
int
*)piom + (DPR CardIndex *
0x100000
) /
4
;
return
(myptr[ArrayIndex /
2
] << (
16
/ (
1
+ (ArrayIndex %
4
) %
2
))) >>
24
;
}
unsigned
int
Acc72EX_GetUdata32(
unsigned
int
CardIndex,
unsigned
int
ArrayIndex)
{
unsigned
int
i = ArrayIndex *
4
, j, k =
0
;
unsigned
int
out =
0
;
for
(j = i; j <= i +
3
; j++)
{
out |= (
unsigned
int
)((
unsigned
int
)Acc72EX_GetData8(CardIndex, j) << (
8
* k));
k++;
}
return
out;
}
int
Acc72EX_GetIdata32(
unsigned
int
CardIndex,
unsigned
int
ArrayIndex)
{
return
(
int
)Acc72EX_GetUdata32(CardIndex, ArrayIndex);
}
void
Acc72EX_SetIdata16(
unsigned
int
CardIndex,
unsigned
int
ArrayIndex,
short
Input)
{
unsigned
int
*myptr = (
unsigned
int
*)piom + (DPR CardIndex *
0x100000
) /
4
;
myptr[ArrayIndex] = (Input <<
8
) &
0x00FFFF00
;
}
void
Acc72EX_SetUdata16(
unsigned
int
CardIndex,
unsigned
int
ArrayIndex,
unsigned
short
Input)
{
unsigned
int
*myptr = (
unsigned
int
*)piom + (DPR CardIndex *
0x100000
) /
4
;
myptr[ArrayIndex] = (Input <<
8
) &
0x00FFFF00
;
}