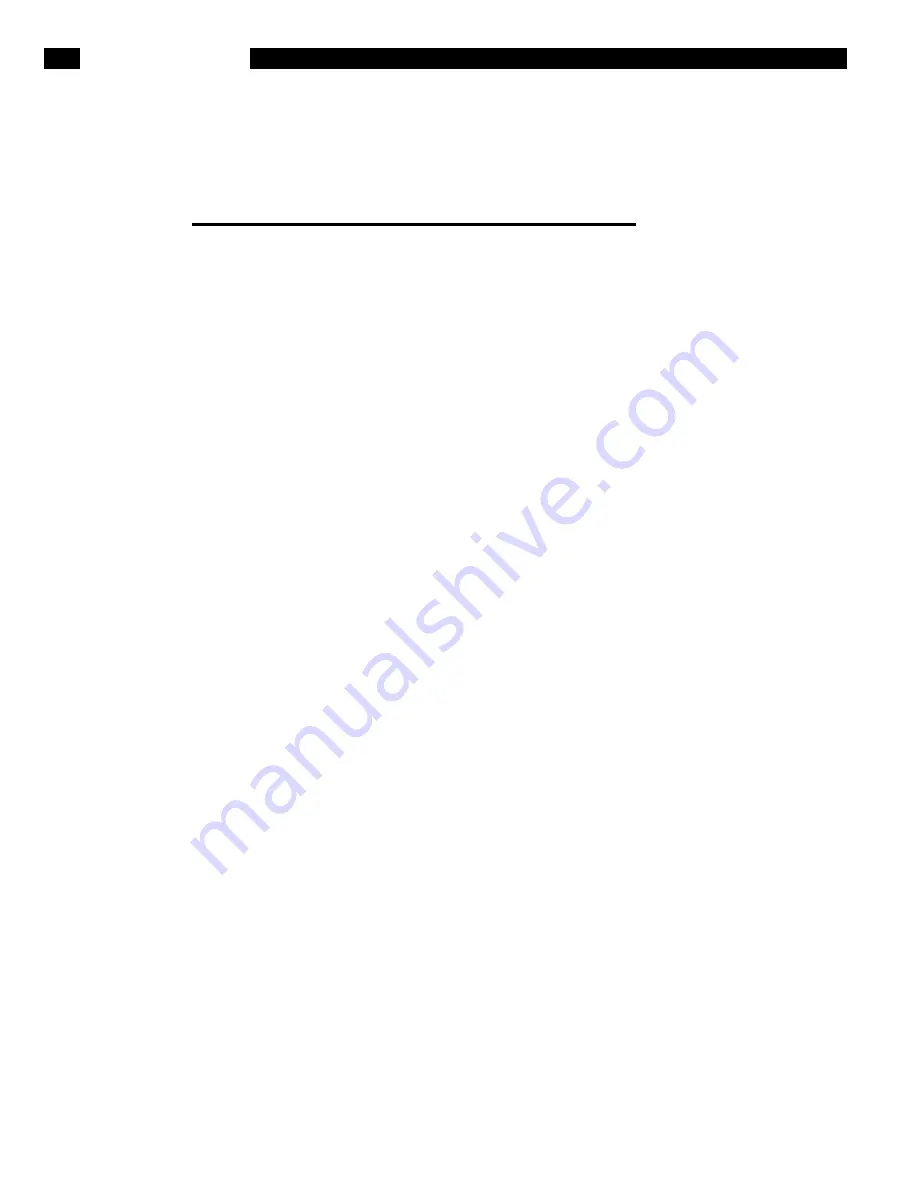
Program Examples
3-16
EXAMPLE 1:
Arbitrary Amplitude Modulation.
This program downloads an arbitrary AM pattern to the DS345. The modulating waveform is a sine wave.
The range of amplitude values will be -100% to +100% of full output, making DSBSC modulation. The pro-
gram calculates the AM pattern values, sets the modulation type to AM, modulation waveform to ARB, down-
loads the pattern, and enables modulation. The program is written in C.
/* program to demonstrate arbitrary AM modulation. Will generate a
DSBSC sine wave signal. Written in Microsoft C and uses National
Instruments GPIB card. Assumes DS345 is installed as device name
DS345. */
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <dos.h>
#include <math.h>
#include <float.h>
#include <decl.h>
/* National Instruments header file */
void main(void);
/* function declaration */
int ds345;
unsigned data[10000]; /* up to 10000 points 2 bytes each */
void main ()
{
char cmd[40];
int i,number,sum;
double t;
if ((ds345 = ibfind("DS345")) < 0) /* open National driver */
{
printf ("Cannot find DS345\n");
exit(1);
}
number = 1000;
/* 1000 points */
sum = 0;
/* initialize checksum */
/* now we will calculate 2-byte amplitude data, each point is
given by value = 32767 * % full amplitude */
for (i = 0 ; i < number ; i++)
{
t = 32767.0 * sin ((6.2831853*(double)i)/(double)number); /* sine wave */
data[i] = (int)(t + 0.5); /* convert to int */
sum += data[i]; /* add to checksum */
}
data[number] = sum; /* store checksum */
sprintf (cmd,"MENA0;MTYP2;MDWF5\n"); /* make sure modulation off until after
loading, set AM, arb WF */
ibwrt (ds345,cmd,strlen(cmd));
/* send commands */
sprintf (cmd,"AMOD?%d\n",number); /* arb modulation command */
ibwrt (ds345,cmd,strlen(cmd));
ibrd (ds345,cmd,40);
/* read back reply before sending data */
ibwrt (ds345,(char *)data,(long)2*2); /* number of bytes = 2 per data
point + 2 for checksum */
Summary of Contents for DS345
Page 2: ......
Page 5: ...DS345 Synthesized Function Generator iii...
Page 20: ...Introduction 2 4...
Page 64: ...Programming Commands 3 14...
Page 72: ...Program Examples 3 22...
Page 78: ...Troubleshooting 4 6...
Page 82: ...Performance Tests 5 4...
Page 101: ...Calibration 6 10...
Page 109: ...Arbitrary Waveform Composer 7 8...
Page 117: ...DS345 Circuitry 8 8...