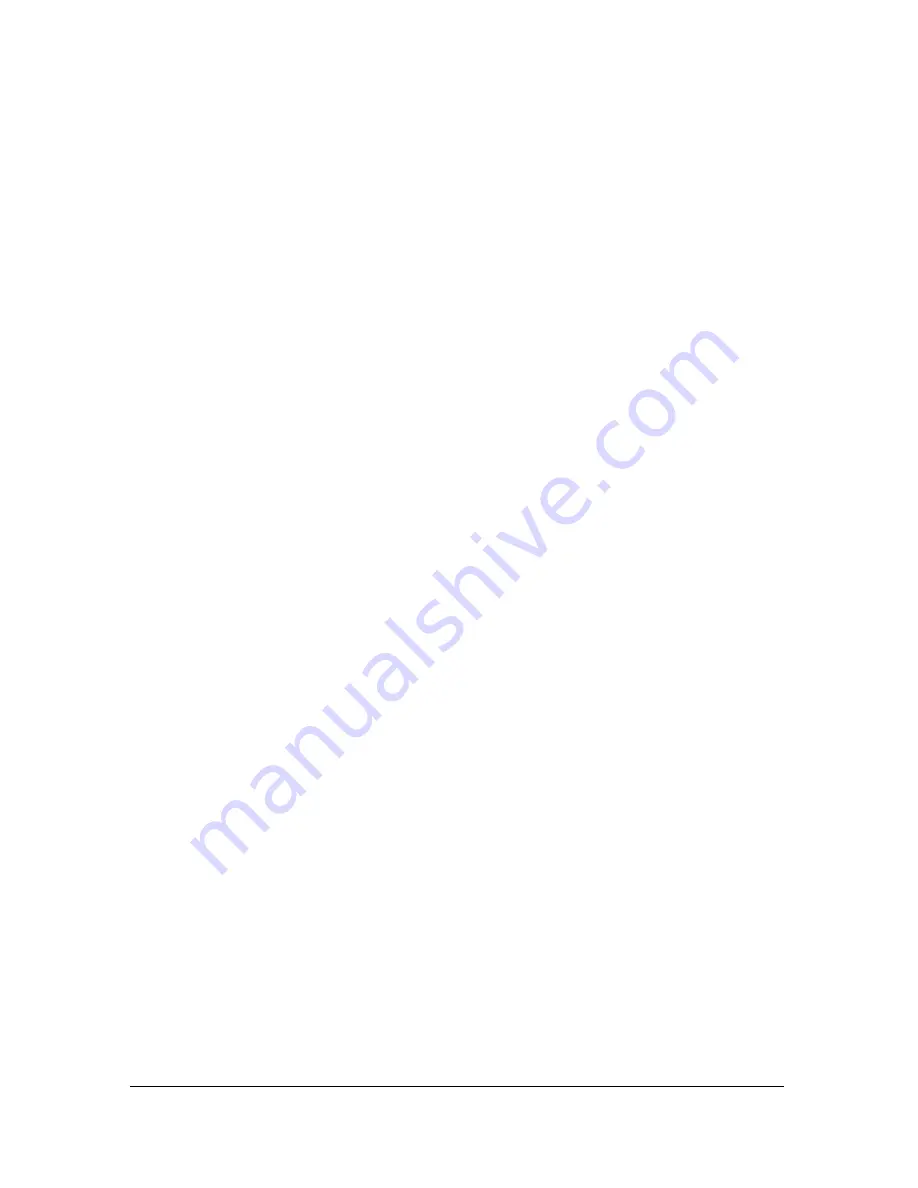
Creating inheritance in ActionScript 1.0
799
All constructor functions have a
prototype
property that is created automatically when the
function is defined. The
prototype
property indicates the default property values for objects
created with that function. You can use the
prototype
property to assign properties and
methods to a class. (For more information, see
“Assigning methods to a custom object in
ActionScript 1.0” on page 795
.)
All instances of a class have a
__proto__
property that tells you the object from which they
inherit. When you use a constructor function to create an object, the
__proto__
property is
set to refer to the
prototype
property of its constructor function.
Inheritance proceeds according to a definite hierarchy. When you call an object’s property or
method, ActionScript looks at the object to see if such an element exists. If it doesn’t exist,
ActionScript looks at the object’s
__proto__
property for the information
(
myObject.__proto__
). If the property is not a property of the object’s
__proto__
object,
ActionScript looks at
myObject.__proto__.__proto__
, and so on.
The following example defines the constructor function
Bike()
:
function Bike(length, color) {
this.length = length;
this.color = color;
this.pos = 0;
}
The following code adds the
roll()
method to the Bike class:
Bike.prototype.roll = function() {return this.pos += 20;};
Then, you can trace the position of the bike with the following code:
var myBike = new Bike(55, "blue");
trace(myBike.roll()); // traces 20.
trace(myBike.roll()); // traces 40.
Instead of adding
roll()
to the MountainBike class and the Tricycle class, you can create the
MountainBike class with Bike as its superclass, as shown in the following example:
MountainBike.prototype = new Bike();
Now you can call the
roll()
method of MountainBike, as shown in the following example:
var myKona = new MountainBike(20, "teal");
trace(myKona.roll()); // traces 20
Movie clips do not inherit from each other. To create inheritance with movie clips, you can
use
Object.registerClass()
to assign a class other than the MovieClip class to movie clips.
Summary of Contents for FLASH 8-LEARNING ACTIONSCRIPT 2.0 IN FLASH
Page 1: ...Learning ActionScript 2 0 in Flash...
Page 8: ...8 Contents...
Page 18: ...18 Introduction...
Page 30: ...30 What s New in Flash 8 ActionScript...
Page 66: ...66 Writing and Editing ActionScript 2 0...
Page 328: ...328 Interfaces...
Page 350: ...350 Handling Events...
Page 590: ...590 Creating Interaction with ActionScript...
Page 710: ...710 Understanding Security...
Page 730: ...730 Debugging Applications...
Page 780: ...780 Deprecated Flash 4 operators...
Page 830: ...830 Index...