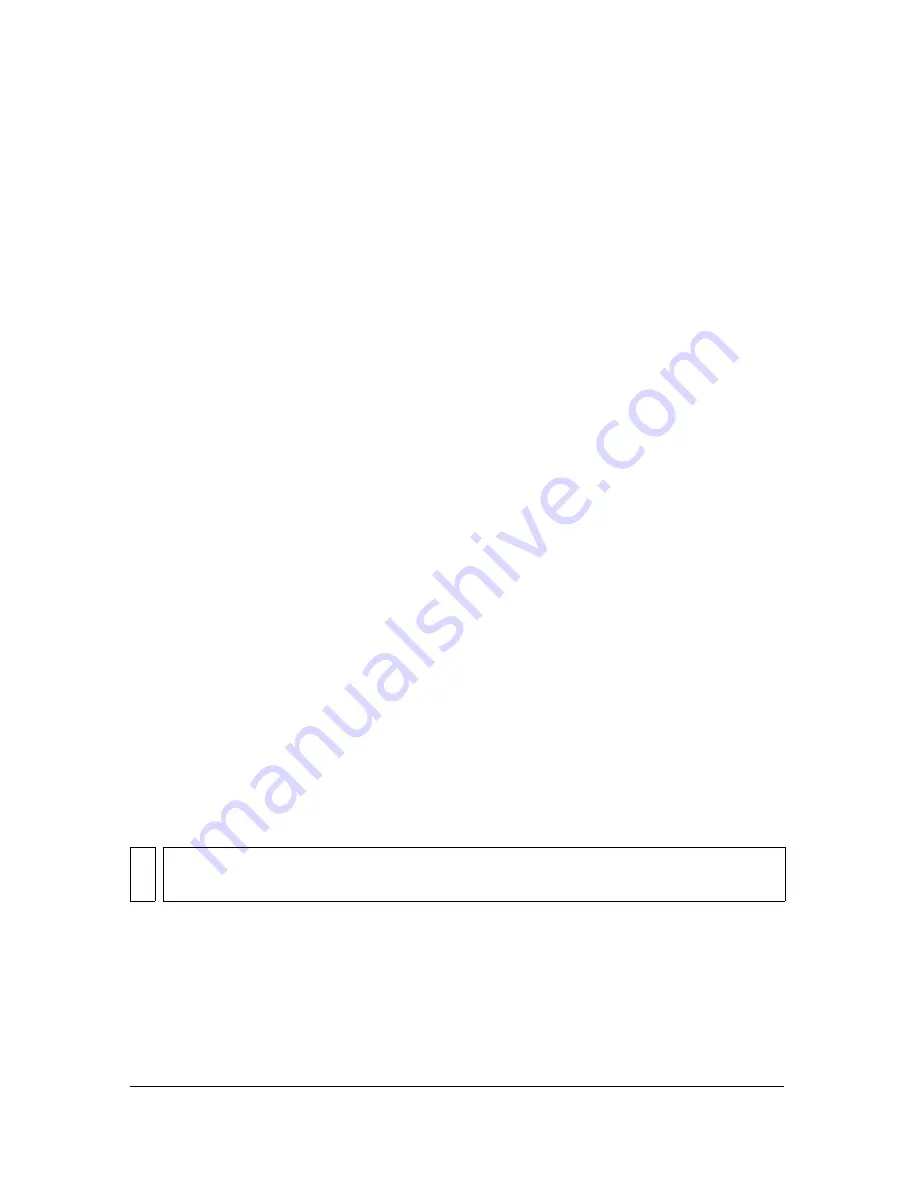
About casting
111
About casting objects
The syntax for casting is
type(item)
, where you want the compiler to behave as if the data
type of the item is
type
. Casting is essentially a function call, and the function call returns
null
if the cast fails at runtime (this occurs in files published for Flash Player 7 or later; files
published for Flash Player 6 do not have runtime support for failed casts). If the cast succeeds,
the function call returns the original object. However, the compiler cannot determine whether
a cast will fail at runtime and won’t generate compile-time errors in those cases.
The following code shows an example:
// Both the Cat and Dog classes are subclasses of the Animal class
function bark(myAnimal:Animal) {
var foo:Dog = Dog(myAnimal);
foo.bark();
}
var curAnimal:Animal = new Dog();
bark(curAnimal); // Will work
curAnimal = new Cat();
bark(curAnimal); // Won't work
In this example, you asserted to the compiler that
foo
is a Dog object, and therefore the
compiler assumes that
foo.bark()
; is a legal statement. However, the compiler doesn’t know
that the cast will fail (that is, that you tried to cast a Cat object to an Animal type), so no
compile-time error occurs. However, if you include a check in your script to make sure that
the cast succeeds, you can find casting errors at runtime, as shown in the following example.
function bark(myAnimal:Animal) {
var foo:Dog = Dog(myAnimal);
if (foo) {
foo.bark();
}
}
You can cast an expression to an interface. If the expression is an object that implements the
interface or has a base class that implements the interface, the cast succeeds. If not, the cast
fails.
You can’t override primitive data types that have a corresponding global conversion function
with a cast operator of the same name. This is because the global conversion functions have
precedence over the cast operators. For example, you can’t cast to Array because the
Array()
conversion function takes precedence over the cast operator.
NOT
E
Casting to null or undefined returns
undefined
.
Summary of Contents for FLASH 8-LEARNING ACTIONSCRIPT 2.0 IN FLASH
Page 1: ...Learning ActionScript 2 0 in Flash...
Page 8: ...8 Contents...
Page 18: ...18 Introduction...
Page 30: ...30 What s New in Flash 8 ActionScript...
Page 66: ...66 Writing and Editing ActionScript 2 0...
Page 328: ...328 Interfaces...
Page 350: ...350 Handling Events...
Page 590: ...590 Creating Interaction with ActionScript...
Page 710: ...710 Understanding Security...
Page 730: ...730 Debugging Applications...
Page 780: ...780 Deprecated Flash 4 operators...
Page 830: ...830 Index...