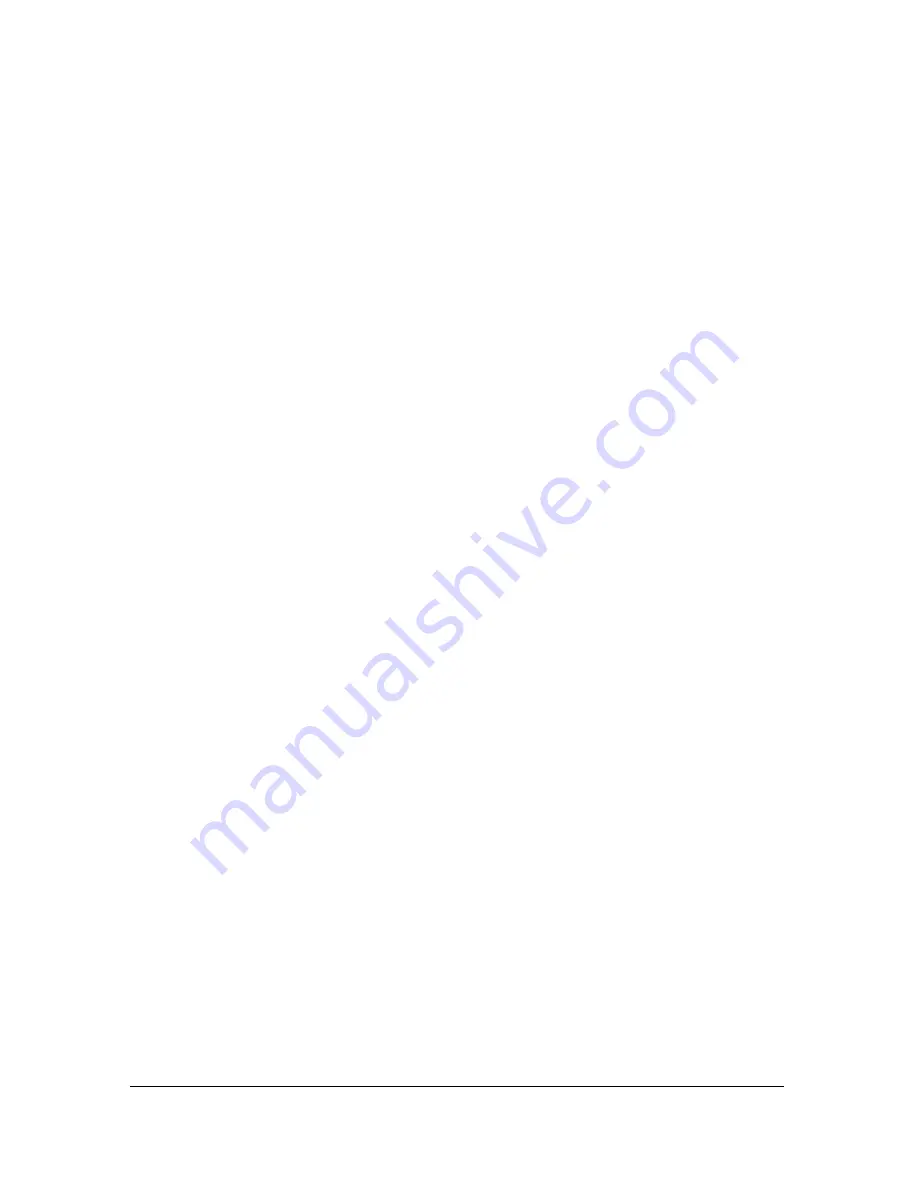
About data types
83
To assign a specific data type to an item, specify its type using the
var
keyword and post-
colon syntax, as shown in the following example:
// Strict typing of variable or object
var myNum:Number = 7;
var birthday:Date = new Date();
// Strict typing of parameters
function welcome(firstName:String, age:Number) {
}
// Strict typing of parameter and return value
function square(myNum:Number):Number {
var squared:Number = myNum * myNum;
return squared;
}
You can declare the data type of objects based on built-in classes (Button, Date, and so on) as
well as classes and interfaces that you create. In the following example, if you have a file
named Student.as in which you define the Student class, you can specify that objects you
create are of type Student:
var myStudent:Student = new Student();
For this example, suppose you type the following code:
// in the Student.as class file
class Student {
public var status:Boolean; // property of Student objects
}
// in the FLA file
var studentMaryLago:Student = new Student();
studentMaryLago.status = "enrolled"; /* Type mismatch in assignment
statement: found String where Boolean is required. */
When Flash compiles this script, a type mismatch error is generated because the SWF file
expects a Boolean value.
If you write a function that doesn’t have a return type, you can specify a return type of Void
for that function. Or if you create a shortcut to a function, you can assign a data type of
Function to the new variable. To specify that objects are of type Function or Void, see the
following example:
function sayHello(name_str:String):Void {
trace("Hello, " + name_str);
}
sayHello("world"); // Hello, world
var greeting:Function = sayHello;
greeting("Augustus"); // Hello, Augustus
Summary of Contents for FLASH 8-LEARNING ACTIONSCRIPT 2.0 IN FLASH
Page 1: ...Learning ActionScript 2 0 in Flash...
Page 8: ...8 Contents...
Page 18: ...18 Introduction...
Page 30: ...30 What s New in Flash 8 ActionScript...
Page 66: ...66 Writing and Editing ActionScript 2 0...
Page 328: ...328 Interfaces...
Page 350: ...350 Handling Events...
Page 590: ...590 Creating Interaction with ActionScript...
Page 710: ...710 Understanding Security...
Page 730: ...730 Debugging Applications...
Page 780: ...780 Deprecated Flash 4 operators...
Page 830: ...830 Index...