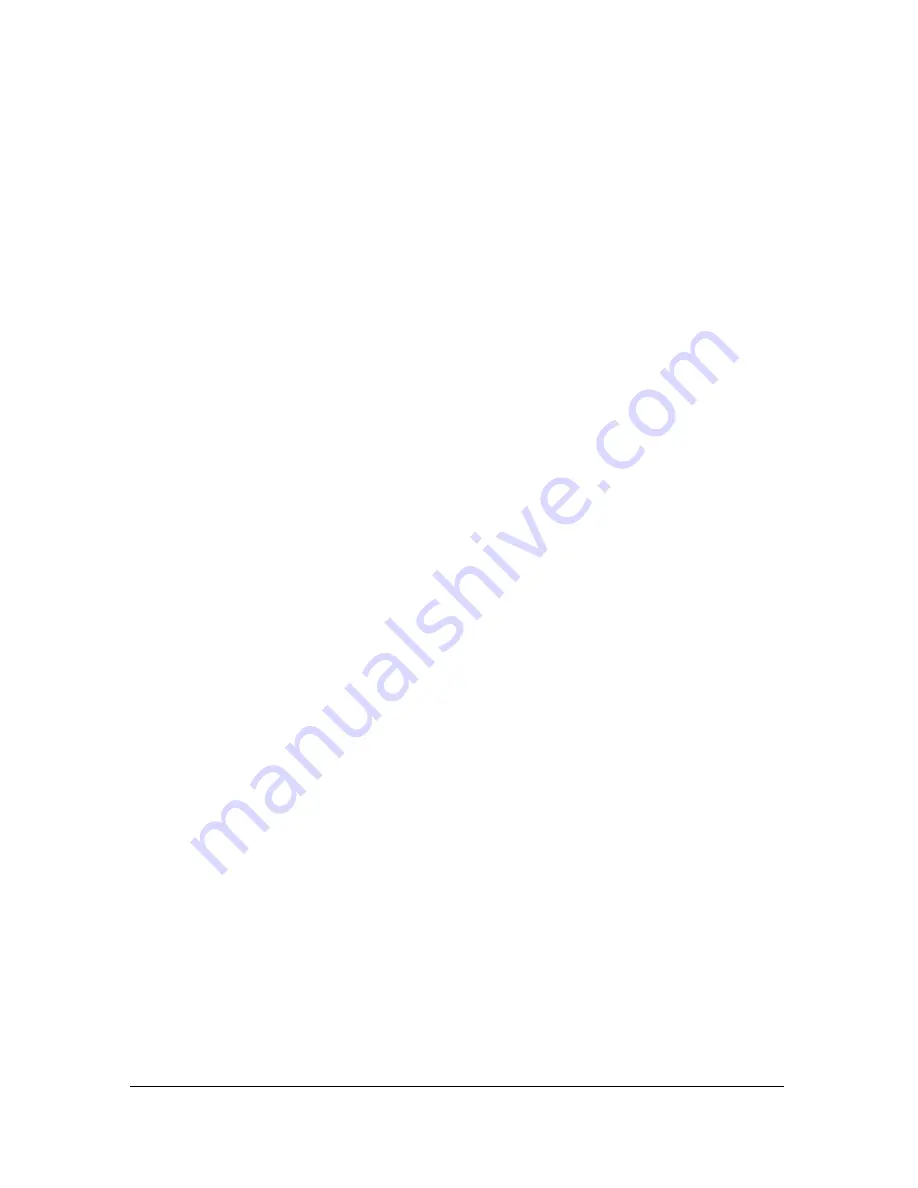
About working with custom classes in an application
251
Similarly, any function declared within a class is considered a method of the class. In the
Person class example, you can create a method called
getInfo()
:
class Person {
public var age:Number;
public var username:String;
public function getInfo():String {
// getInfo() method definition
}
}
In the previous code snippet the Person class’s
getInfo()
method, as well as the
age
and
username
properties, are all public instance members. The
age
property would not be a good
class member, because each person has a different age. Only properties and methods that are
shared by all individuals of the class should be class members.
Suppose that you want every class to have a
species
variable that indicates the proper Latin
name for the species that the class represents. For every Person object, the species is
Homo
sapiens
. It would be wasteful to store a unique copy of the string
"Homo sapiens"
for every
instance of the class, so this member should be a class member.
Class members are declared with the
static
keyword. For example, you could declare the
species class member with the following code:
class Person {
public static var species:String = "Homo sapiens";
// ...
}
You can also declare methods of a class to be static, as shown in the following code:
public static function getSpecies():String {
return Person.species;
}
Static methods can access only static properties, not instance properties. For example, the
following code results in a compiler error because the class method
getAge()
references the
instance variable
age
:
class Person {
public var age:Number = 15;
// ...
public static function getAge():Number {
return age; /* **Error**: Instance variables cannot be accessed in
static functions. */
}
}
To solve this problem, you could either make the method an instance method or make the
variable a class variable.
Summary of Contents for FLASH 8-LEARNING ACTIONSCRIPT 2.0 IN FLASH
Page 1: ...Learning ActionScript 2 0 in Flash...
Page 8: ...8 Contents...
Page 18: ...18 Introduction...
Page 30: ...30 What s New in Flash 8 ActionScript...
Page 66: ...66 Writing and Editing ActionScript 2 0...
Page 328: ...328 Interfaces...
Page 350: ...350 Handling Events...
Page 590: ...590 Creating Interaction with ActionScript...
Page 710: ...710 Understanding Security...
Page 730: ...730 Debugging Applications...
Page 780: ...780 Deprecated Flash 4 operators...
Page 830: ...830 Index...