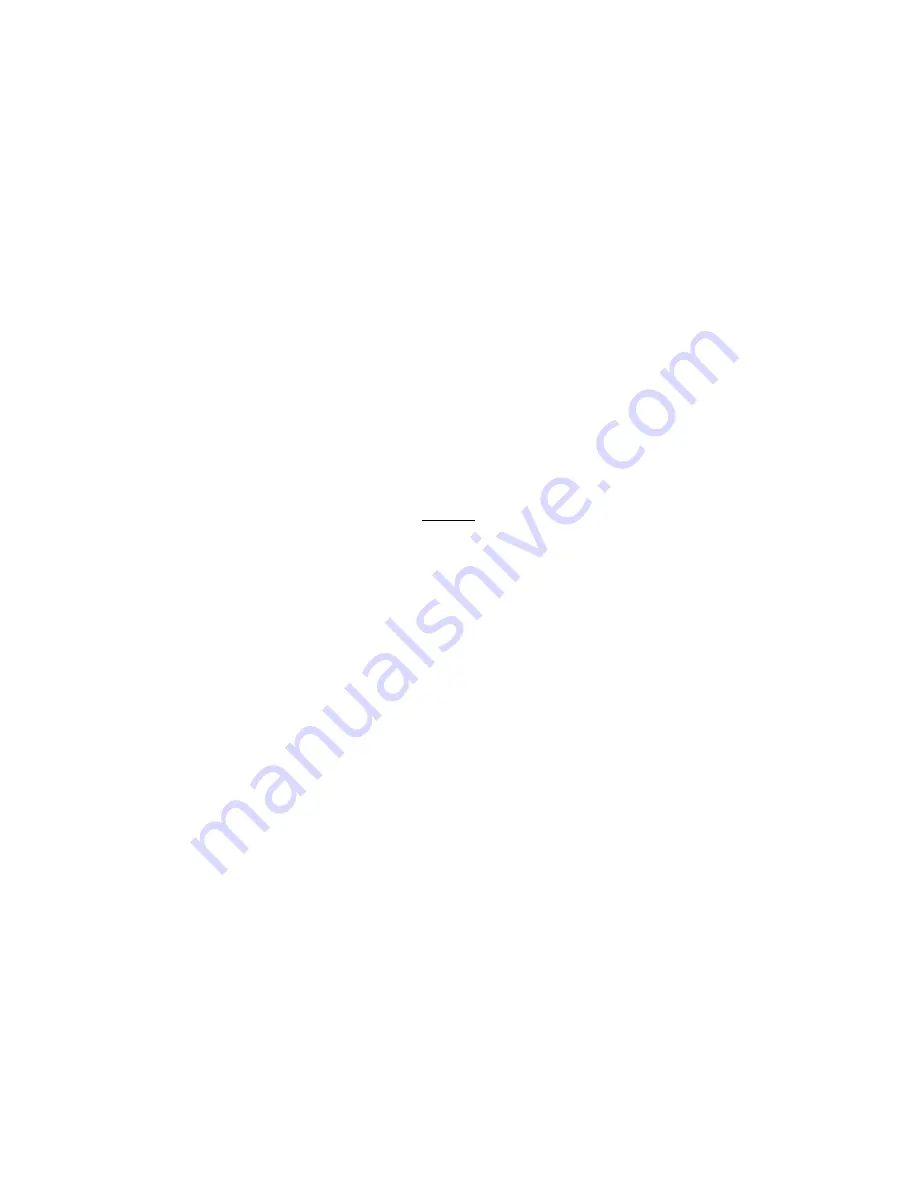
//Read data until done.
while(!done)
{
//Must set the number of scans to read each iteration, as the read
//returns the actual number read.
numScans = 1000;
//Read the data. Note that the array passed must be sized to hold
//enough SAMPLES, and the Value passed specifies the number of SCANS
//to read.
eGet(lngHandle, LJ_ioGET_STREAM_DATA, LJ_chALL_CHANNELS, &numScans, array);
actualNumberRead = numScans;
//When all channels are retrieved in a single read, the data
//is interleaved in a 1-dimensional array. The following lines
//get the first sample from each channel.
channelA = array[0];
channelB = array[1];
//Retrieve the current U3 backlog. The UD driver retrieves
//stream data from the U3 in the background, but if the computer
//is too slow for some reason the driver might not be able to read
//the data as fast as the U3 is acquiring it, and thus there will
//be data left over in the U3 buffer.
eGet(lngHandle, LJ_ioGET_CONFIG, LJ_chSTREAM_BACKLOG_COMM, &dblCommBacklog, 0);
//Retrieve the current UD driver backlog. If this is growing, then
//the application software is not pulling data from the UD driver
//fast enough.
eGet(lngHandle, LJ_ioGET_CONFIG, LJ_chSTREAM_BACKLOG_UD, &dblUDBacklog, 0);
}
Finally, stop the stream:
//Stop the stream.
errorcode = ePut (Handle, LJ_ioSTOP_STREAM, 0, 0, 0);
4.3.8 - Raw Output/Input
There are two IOTypes used to write or read raw data. These can be used to make low-level function calls (Section 5) through the
UD driver. The only time these generally might be used is to access some low-level device functionality not available in the UD
driver.
LJ_ioRAW_OUT
LJ_ioRAW_IN
When using these IOTypes, channel # specifies the desired communication pipe. For the U3, 0 is the normal pipe while 1 is the
streaming pipe. The number of bytes to write/read is specified in value (1-16384), and x1 is a pointer to a byte array for the data.
When retrieving the result, the value returned is the number of bytes actually read/written.
Following is example pseudocode to write and read the low-level command ConfigTimerClock (Section 5.2.4).
writeArray[2] = {0x05,0xF8,0x02,0x0A,0x00,0x00,0x00,0x00,0x00,0x00};
numBytesToWrite = 10;
numBytesToRead = 10;
//Raw Out. This command writes the bytes to the device.
eGet(lngHandle, LJ_ioRAW_OUT, 0, &numBytesToWrite, pwriteArray);
//Raw In. This command reads the bytes from the device.
eGet(lngHandle, LJ_ioRAW_IN, 0, &numBytesToRead, preadArray);
4.3.9 - Easy Functions
The easy functions are simple alternatives to the very flexible IOType based method normally used by this driver. There are 6
functions available:
eAIN() //Read 1 analog input.
eDAC() //Write to 1 analog output.
eDI() //Read 1 digital input.
eDO() //Write to 1 digital output.
eTCConfig() //Configure all timers and counters.
eTCValues() //Update/reset and read all timers and counters.
In addition to the basic operations, these functions also automatically handle configuration as needed. For example, eDO() sets
the specified line to digital output if previously configured as analog and/or input, and eAIN() sets the line to analog if previously
configured as digital. Passing a -1 to any of the configuration parameters (resolution, range, etc) will use the driver's current value
instead of having to specify it. This is useful so that you can use the driver's default values for those properties, which will work in
most cases.
The first 4 functions should not be used when speed is critical with multi-channel reads. These functions use one low-level function
per operation, whereas using the normal Add/Go/Get method with IOTypes, many operations can be combined into a single low-
level call. With single channel operations, however, there will be little difference between using an easy function or Add/Go/Get.
The last two functions handle almost all functionality related to timers and counters, and will usually be as efficient as any other
method. These easy functions are recommended for most timer/counter applications.
Following is example pseudocode:
//Take a single-ended measurement from AIN3.
//eAIN (Handle, ChannelP, ChannelN, *Voltage, Range, Resolution,
// Settling, Binary, Reserved1, Reserved2)
//
eAIN(lngHandle, 3, 31, &dblVoltage, 0, 0, 0, 0, 0, 0);
printf("AIN3 value = %.3f\n",dblVoltage);
//Set DAC0 to 3.1 volts.
//eDAC (Handle, Channel, Voltage, Binary, Reserved1, Reserved2)
//
eDAC(lngHandle, 0, 3.1, 0, 0, 0);
//Read state of FIO2.
//eDI (Handle, Channel, *State)
//
eDI(lngHandle, 2, &lngState);
printf("FIO2 state = %.0f\n",lngState);
//Set FIO3 to output-high.
//eDO (Handle, Channel, State)
//
eDO(lngHandle, 3, 1);
//Enable and configure 1 output timer and 1 input timer, and enable Counter0.
//Fill the arrays with the desired values, then make the call.
alngEnableTimers = {1,1}; //Enable Timer0-Timer1
38