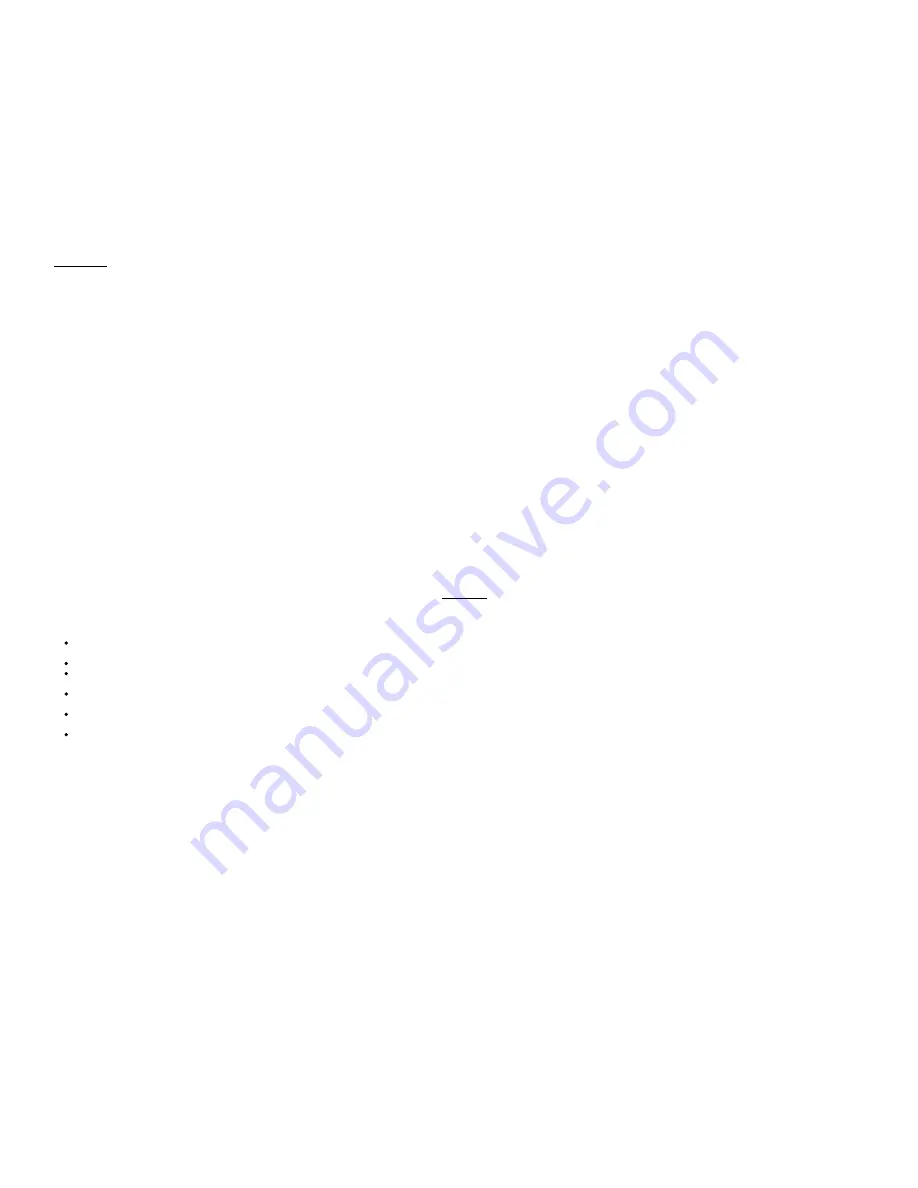
//Use the following line to open the first found LabJack U3
//over USB and get a handle to the device.
//The general form of the open function is:
//OpenLabJack (DeviceType, ConnectionType, Address, FirstFound, *Handle)
//Open the first found LabJack U3 over USB.
lngErrorcode = OpenLabJack (LJ_dtU3, LJ_ctUSB, "1", TRUE, &lngHandle);
Second, a list of requests is built in the UD driver using AddRequest calls. This does not involve any low-level communication with
the device, and thus the execution time is relatively instantaneous:
//Request that DAC0 be set to 2.5 volts.
//The general form of the AddRequest function is:
//AddRequest (Handle, IOType, Channel, Value, x1, UserData)
lngErrorcode = AddRequest (lngHandle, LJ_ioPUT_DAC, 0, 2.50, 0, 0);
//Request a read from AIN3 (FIO3), assuming it has been enabled as
//an analog line.
lngErrorcode = AddRequest (lngHandle, LJ_ioGET_AIN, 3, 0, 0, 0);
Third, the list of requests is processed and executed using a Go call. In this step, the driver determines which low-level commands
must be executed to process all the requests, calls those low-level functions, and stores the results. This example consists of two
requests, one analog input read and one analog output write, which can both be handled in a single low-level Feedback call
(Section 5.2.5):
//Execute the requests.
lngErrorcode = GoOne (lngHandle);
Finally, GetResult calls are used to retrieve the results (errorcodes and values) that were stored by the driver during the Go call.
This does not involve any low-level communication with the device, and thus the execution time is relatively instantaneous:
//Get the result of the DAC0 request just to check for an errorcode.
//The general form of the GetResult function is:
//GetResult (Handle, IOType, Channel, *Value)
lngErrorcode = GetResult (lngHandle, LJ_ioPUT_DAC, 0, 0);
//Get the AIN3 voltage. We pass the address to dblValue and the
//voltage will be returned in that variable.
lngErrorcode = GetResult (lngHandle, LJ_ioGET_AIN, 3, &dblValue);
The AddRequest/Go/GetResult method is often the most efficient. As shown above, multiple requests can be executed with a
single Go() or GoOne() call, and the driver might be able to optimize the requests into fewer low-level calls. The other option is to
use the eGet or ePut functions which combine the AddRequest/Go/GetResult into one call. The above code would then look like
(assuming the U3 is already open):
//Set DAC0 to 2.5 volts.
//The general form of the ePut function is:
//ePut (Handle, IOType, Channel, Value, x1)
lngErrorcode = ePut (lngHandle, LJ_ioPUT_DAC, 0, 2.50, 0);
//Read AIN3.
//The general form of the eGet function is:
//eGet (Handle, IOType, Channel, *Value, x1)
lngErrorcode = eGet (lngHandle, LJ_ioGET_AIN, 3, &dblValue, 0);
In the case of the U3, the first example using add/go/get handles both the DAC command and AIN read in a single low-level call,
while in the second example using ePut/eGet two low-level commands are used. Examples in the following documentation will use
both the add/go/get method and the ePut/eGet method, and they are generally interchangeable. See Section 4.3 for more
pseudocode examples.
All the request and result functions always have 4 common parameters, and some of the functions have 2 extra parameters:
Handle
– This is an input to all request/result functions that tells the function what LabJack it is talking to. The handle is
obtained from the OpenLabJack function.
IOType
– This is an input to all request/result functions that specifies what type of action is being done.
Channel
– This is an input to all request/result functions that generally specifies which channel of I/O is being written/read,
although with the config IOTypes special constants are passed for channel to specify what is being configured.
Value
– This is an input or output to all request/result functions that is used to write or read the value for the item being
operated on.
x1
– This parameter is only used in some of the request/result functions, and is used when extra information is needed for
certain IOTypes.
UserData
– This parameter is only used in some of the request/result functions, and is data that is simply passed along with
the request, and returned unmodified by the result. Can be used to store any sort of information with the request, to allow a
generic parser to determine what should be done when the results are received.
4.1.1 - Function Flexibility
The driver is designed to be flexible so that it can work with various different LabJacks with different capabilities. It is also
designed to work with different development platforms with different capabilities. For this reason, many of the functions are
repeated with different forms of parameters, although their internal functionality remains mostly the same. In this documentation, a
group of functions will often be referred to by their shortest name. For example, a reference to Add or AddRequest most likely
refers to any of the three variations: AddRequest(), AddRequestS() or AddRequestSS(). In the sample code, alternate functions (S
or SS versions) can generally be substituted as desired, changing the parameter types accordingly. All samples here are written in
pseudo-C. Functions with an “S” or “SS” appended are provided for programming languages that can’t include the LabJackUD.h
file and therefore can’t use the constants included. It is generally poor programming form to hardcode numbers into function calls, if
for no other reason than it is hard to read. Functions with a single “S” replace the IOType parameter with a const char * which is a
string. A string can then be passed with the name of the desired constant. Functions with a double “SS” replace both the IOType
and Channel with strings. OpenLabJackS replaces both DeviceType and ConnectionType with strings since both take constants.
For example: In C, where the LabJackUD.h file can be included and the constants used directly:
AddRequest(Handle, LJ_ioGET_CONFIG, LJ_chHARDWARE_VERSION,0,0,0);
The bad way (hard to read) when LabJackUD.h cannot be included:
AddRequest(Handle, 1001, 10, 0, 0, 0);
The better way when LabJackUD.h cannot be included, is to pass strings:
AddRequestSS(Handle, “LJ_ioGET_CONFIG”, “LJ_chHARDWARE_VERSION”,0,0,0);
Continuing on this vein, the function StringToConstant() is useful for error handling routines, or with the GetFirst/Next functions
which do not take strings. The StringToConstant() function takes a string and returns the numeric constant. So, for example:
LJ_ERROR err;
err = AddRequestSS(Handle, “LJ_ioGETCONFIG”, “LJ_chHARDWARE_VERSION”,0,0,0);
if (err == StringToConstant(“LJE_INVALID_DEVICE_TYPE”))
do some error handling..
Once again, this is much clearer than:
25