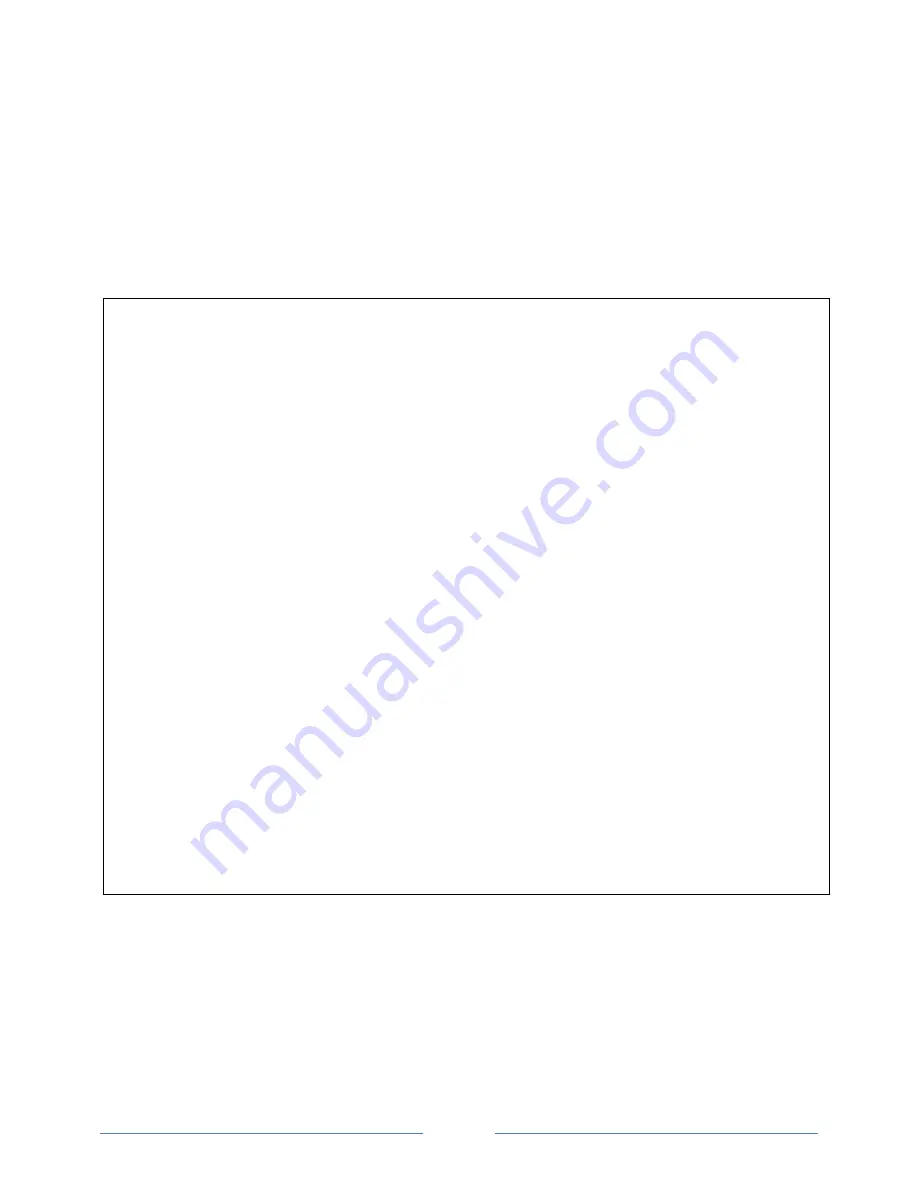
Page 45
Example
The following program includes a function that uses
create_write_byte
to define and send a script to
the iRobot Create byte by byte. The script is designed to move the Create a specified distance at a
specified speed and illustrates the low level coding involved in defining a script.
Code
When an
int
in the range -32,768-32,767 is provided as the argument for
create_write_byte
, the value
assigned to the byte is the low order byte (bits 0-7). To change this to bits 8-15, first right shift (>>) the
int
by 8 bits.
#define RUN_SCRIPT create_write_byte(153)
void
make_drive_script(
int
speed,
int
dist) {
create_write_byte(
152
);
// specifies start of script definition
create_write_byte(
13
);
// remaining number of bytes in script
create_write_byte(
137
);
// drive command, speed & radius follow
create_write_byte(speed >>
8
);
// send bits 8-15 of speed
create_write_byte(speed);
// and then send bits 0-7
create_write_byte(
128
);
// send hex 80 (X8000 specs drive straight)
create_write_byte(
0
);
// and then send hex 00 (no turn radius)
create_write_byte(
156
);
// wait for distance command, dist follows
create_write_byte(dist >>
8
);
// send the 2 bytes
create_write_byte(dist);
// for distance in mm
create_write_byte(
137
);
// stop by dropping speed and radius to 0
create_write_byte(
0
);
// speed = 0
create_write_byte(
0
);
create_write_byte(
0
);
// turn radius = 0
create_write_byte(
0
);
// end of script (15 bytes)
}
int
main() {
create_connect();
set_create_distance(
0
);
set_create_total_angle(
0
);
make_drive_script(
500
,
500
);
// script to move 0.5m at 500 mm/sec
msleep(
500
);
// give serial connection some time
RUN_SCRIPT;
msleep(
1500
);
// allow time for the script to finish (+ some extra)
printf(
" distance traveled = %d mm\n"
, get_create_distance());
printf(
" angle turned = %d degrees\n"
, get_create_total_angle());
create_disconnect();
}