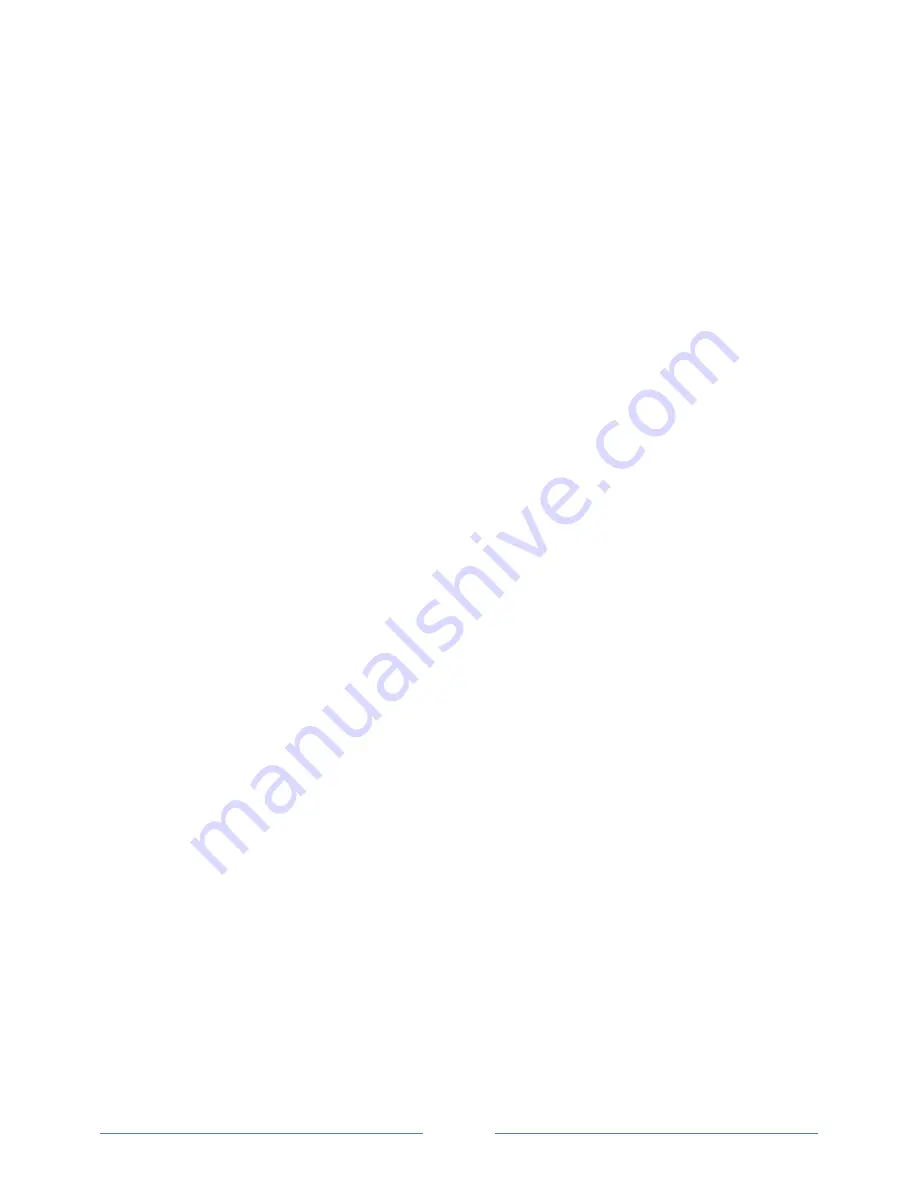
Page 50
File I/O for a USB Flash Drive Plugged into the KIPR Link
Before the Linux operating system can access a file system, it has to "mount" the file system. When a
USB flash drive is plugged into the KIPR Link, it is automatically mounted. When the USB flash drive is
unplugged it is automatically unmounted. The C Library has a number of functions designed to access
files located in mounted file systems. The library functions
fprintf
and
fscanf
respectively provide a
straight forward means for writing formatted output to a file on a USB drive plugged into the KIPR Link,
and for reading formatted data from a file on the USB drive. There are a number of file processing
commands, including ones for accessing files byte by byte. For a full description of the range of functions
available consult a standard C reference book.
To access a file, in addition to the file name, the directory "path" leading to the file has to be known. For
the KIPR Link, the directory path to a mounted Flash drive in a USB port is
/kovan/media/sda1/
Files are accessed in C via a pointer of type FILE, which is defined in the system header file
<stdio.h>
.
The pointer for a file is established when the file is "opened" for access. If the
fopen
function returns a
NULL pointer, it indicates that either the file doesn't exist for the specified file path, or its file system
hasn't been mounted (e.g., the USB drive has not been plugged in). Both cases are illustrated in the
following program for a USB drive plugged into a KIPR Link. The example otherwise is a program
designed to send data to a file, close the file, then reopen the file and retrieve the data to verify a
successful write operation. If the file doesn't exist it is created. If it does exist, it is appended to. A user
defined preprocessor macro (USB) is constructed to set the file path for the USB drive, illustrating how
the preprocessor can be used to potentially simplify program code.
Example program using
fprintf
and
fscanf
:
#include <stdio.h>
// make sure file I/O is defined
/* USB is a Macro defined to preface a file name with the directory path for
a mounted USB drive, turning the result into a character string */
#ifndef USB
/* An auxiliary macro is employed that converts its argument into a string
(by surrounding it with double quote marks) */
#define _STRINGIFY_(x) #x
/* the USB macro appends x to the path for the USB drive, then uses
_STRINGIFY_ to turn it into a string */
#define USB(x) _STRINGIFY_(/kovan/media/sda1/x)
#endif
int
main() {
FILE *f;
// file pointer f (the FILE data type is in <stdio.h>)
// A file name "myUSBfile" for the USB drive is set up using macro USB
char
s[
81
], chkf[] = USB(myUSBfile);
// file path as a string variable
int
x, data =
2
;
// try opening for read ("r") to see if the file exists
if
((f = fopen(chkf,
"r"
)) != NULL) {
fclose(f);
// file chkf already exists
printf(
"Will be appending to USB %s\n"
, chkf);
}
// (file given by chkf is not open at this point)