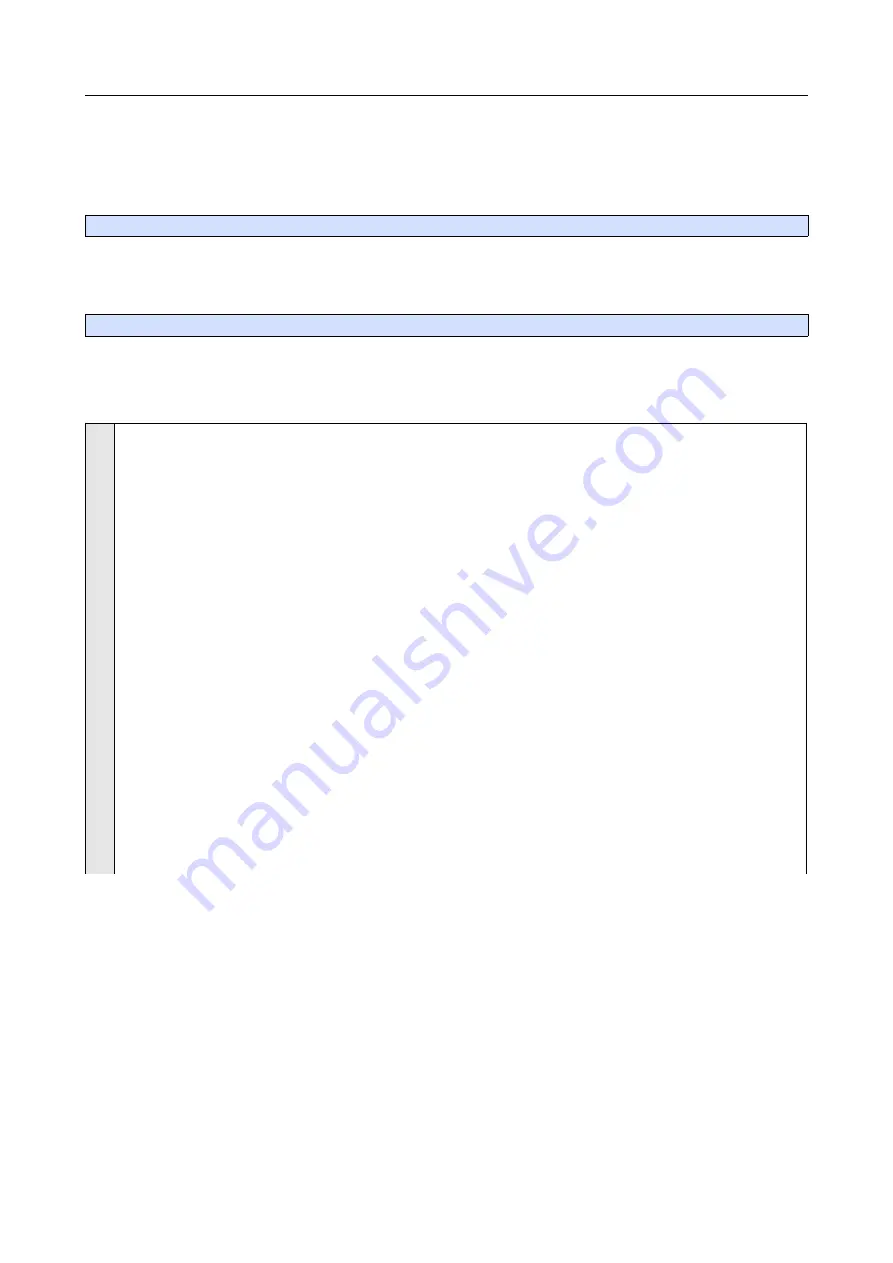
RP6 ROBOT SYSTEM - 4. Programming the RP6
Now, as we are performing background tasks anyway, we can run some other (smal-
ler) tasks as well along with the bigger things – such as bumper evaluation. This is a
simple and fast task which you would usually perform in the main loop anyway.
To automatically check the bumpers you have to call this function:
void task_Bumpers(void)
frequently from the main loop (s. a. chapter about driving functions, in which we will
discuss this in detail). This function will automatically check the bumper sensors at in-
tervals of 50ms (pressed or not) and writes their current state into the variables:
bumper_left and bumper_right
You may use these variables anywhere in the program e.g. in if-conditions, loops etc.,
or assign them to other variables.
Example:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
#include "RP6RobotBaseLib.h"
int
main
(
void
)
initRobotBase
();
// Initialize the Microcontroller
setLEDs
(
0b001001
);
// Turn LEDs 1 and 4 on (both green)
while
(
true
)
{
// Set the LEDs depending on which
// bumpers are pressed down:
statusLEDs
.
LED6
=
bumper_left
;
// Left bumper pressed
statusLEDs
.
LED4
=
!bumper_left
;
// Left bumper released
statusLEDs
.
LED3
=
bumper_right
;
// Right bumper pressed
statusLEDs
.
LED1
=
!bumper_right
;
// Right bumper released
// Both bumpers pressed down:
statusLEDs
.
LED2
=
(
bumper_left && bumper_right
);
statusLEDs
.
LED5
=
statusLEDs
.
LED2
;
updateStatusLEDs
();
// update LEDs...
// Check bumper status:
task_Bumpers
();
// Frequently call this from the main loop!
}
return
0
;
}
The sample program is using the Status LEDs to show the bumper status. Pressing
down the left bumper will turn LED6 on and turn LED4 off. In contrast releasing the
left bumper will turn LED6 off and turn LED4 on. Pressing down the left bumper turns
LED6 on anyway, but here we want to demonstrate the LED usage in general and you
could use anything else to control the LEDs like shown above!
The example works similar for the right bumper with LED3 and LED1. Pressing down
both bumper sensors will turn LED2 and LED5 on.
Equipped with such an automatic check for the Bumpers, it was self-evident to create
something that calls a self defined function automatically everytime the state of the
bumpers changes. Usually the Bumpers will be hit very rarely only and it makes sense
to check this in the main program only if necessary.
- 88 -