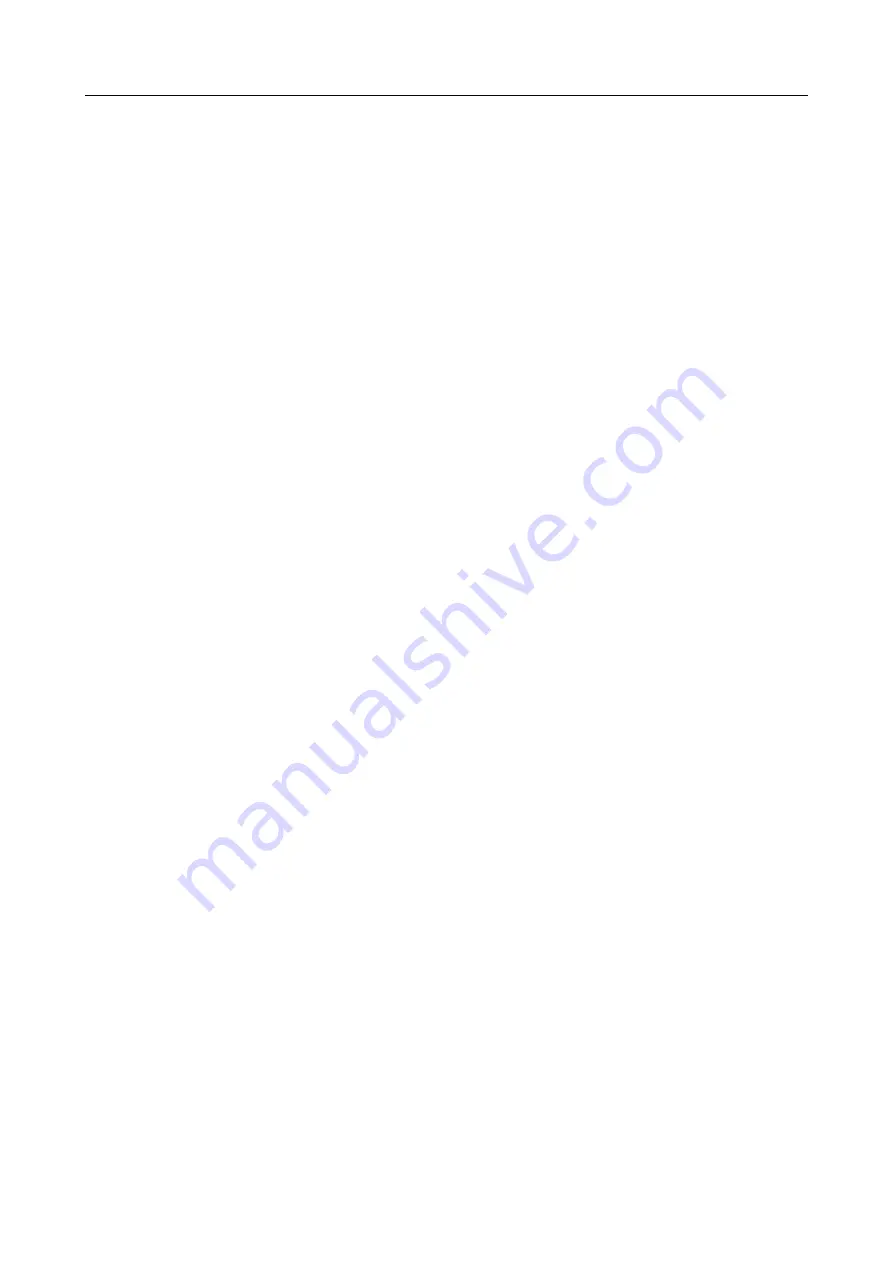
RP6 ROBOT SYSTEM - 4. Programming the RP6
Anyone feeling bored by the tiny sample program may find a more attractive "Hello
World" program in the RP6 example directory, including a running light with the LEDs
and some more text outputs!
Now let's discuss the program in Listing 1 and explain it line by line!
Line 1 - 3:
/* A small and simple "Hello World" C Program for the RP6! */
These are comment lines and will not be interpreted by the compiler. Comments are
used for documenting the source code and they start with /* and end with */.
Documentation will help understanding programs written by other people, but it will
also be helpful in understanding your own programs as well, especially the source
codes you have written years ago!
You may write comments with any length, or “comment out“ parts of your source code
in order to test another program variant without having to delete the original code.
Apart from these standard multi-line comments GCC also supports single-line com-
ments initiated by “//”. AFTER “//” any text will be interpreted as a comment until the
end of the line.
Line 5:
#include "RP6RobotBaseLib.h"
This includes the RP6 function library, providing a great number of useful functions
and predefined things for low level hardware control. To include such a library we use
so-called header files (with extension “*.h”) to inform the compiler where to look for
these functions. Headers are used for all things in external C-files that should be ac-
cessible in other C-files. Please take a look at the contents of RP6RobotBaseLib.h and
RP6RobotBaseLib.c – this should clarify the basic principle. We will discuss more de-
tails of the “#include”-feature in the pre-processor chapter.
Line 7:
int
main
(
void
)
This line defines the most important function in the sample program: the main func-
tion. We still have to learn about what functions are in detail, but right now we may
accept the idea that the program starts at this line.
Line 8 and 12:
{ }
In C, so-called “blocks” can be defined with accolades '{' and '}'.
A block combines several commands.
Line 9:
initRobotBase
();
A function from the RP6Library gets called here. It will initialize the AVR microcontrol-
ler and configure the AVR's hardware modules. Most of the microcontroller's functions
would not work as expected, if we do not initialize them with
initRobotBase
()
, so
please do not forget to always call this function at the beginning of a program!
Line 10:
writeString
(
"Hello World!\n"
);
This calls the function "writeString" from the RP6 Library with the parameter String
"Hello World!\n"
. The function will output the text to the serial interface.
Zeile 11:
return
0
;
Our program ends here. We leave the main-function and return zero. A return code is
usually used in larger systems (with operating system) as an error code or for similar
- 64 -