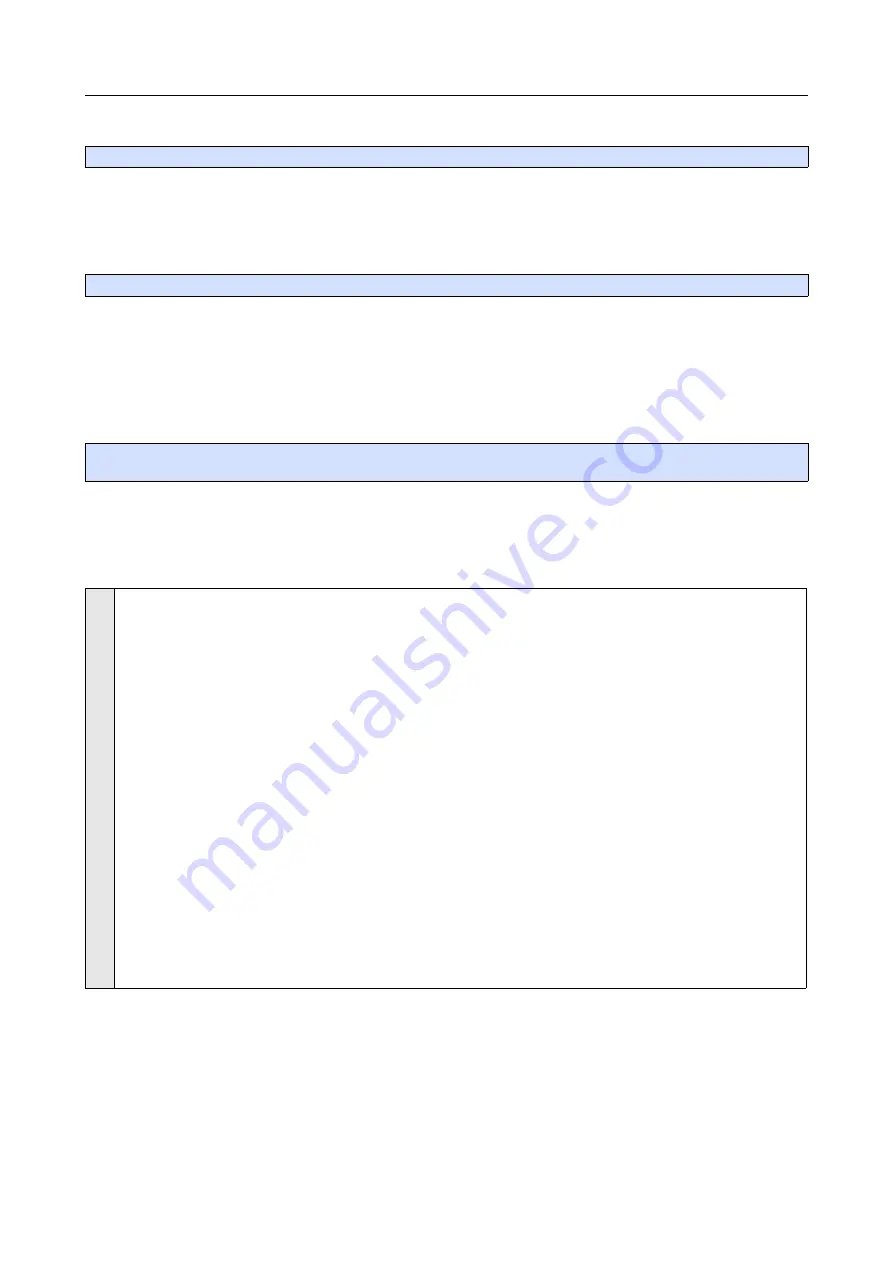
RP6 ROBOT SYSTEM - 4. Programming the RP6
The function:
uint8_t isMovementComplete(void)
can be used to check if movement-commands are completed. If there are unfinished
movement-commands, the return value will be “false”.
Whenever a move-command has to be aborted, e.g. at detection of an obstacle, you
can terminate all movements by calling:
void stop(void)
which will stop all movements.
It would be already possible to use the move function for rotating by simply setting
the direction parameter to LEFT or RIGHT instead of FWD or BWD and specifying a
suitable distance value corresponding to an angle. This is a rather clumsy method and
does not perform very well. For this reason we provided a dedicated function for rota-
tion on the spot:
void rotate(uint8_t desired_speed, uint8_t dir, uint16_t angle,
uint8_t blocking)
This function behaves just like the “move”-command, the only difference is that you
need to specify an angle instead of a distance. The blocking parameter can be used
with this function, too.
The following example program shows how to use both functions:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
#include "RP6RobotBaseLib.h"
int
main
(
void
)
{
initRobotBase
();
setLEDs
(
0b111111
);
mSleep
(
1500
);
powerON
();
// Activate encoders and motor current sensors!
while
(
true
)
{
setLEDs
(
0b100100
);
move
(
60
,
FWD
,
DIST_MM
(
300
),
true
);
// Move 30cm forward
setLEDs
(
0b100000
);
rotate
(
50
,
LEFT
,
180
,
true
);
// Rotate 180° to the left
setLEDs
(
0b100100
);
move
(
60
,
FWD
,
DIST_MM
(
300
),
true
);
// Move 30cm forward
setLEDs
(
0b000100
);
rotate
(
50
,
RIGHT
,
180
,
true
);
// Rotate 180° to the right
}
return
0
;
}
The Robot will move 30cm forward, rotate 180° to the left, move 30cm backwards, ro-
tate 180° to the right and start from the beginning. If you would set all of the blocking
parameters to false, the program would not work at all. The main loop does not call
the task_motionControl function and all movement function calls are in one sequence.
Changing only one blocking parameter to false, the program will not work as intended
anymore. One of the movement phases will be skipped completely then.
- 102 -