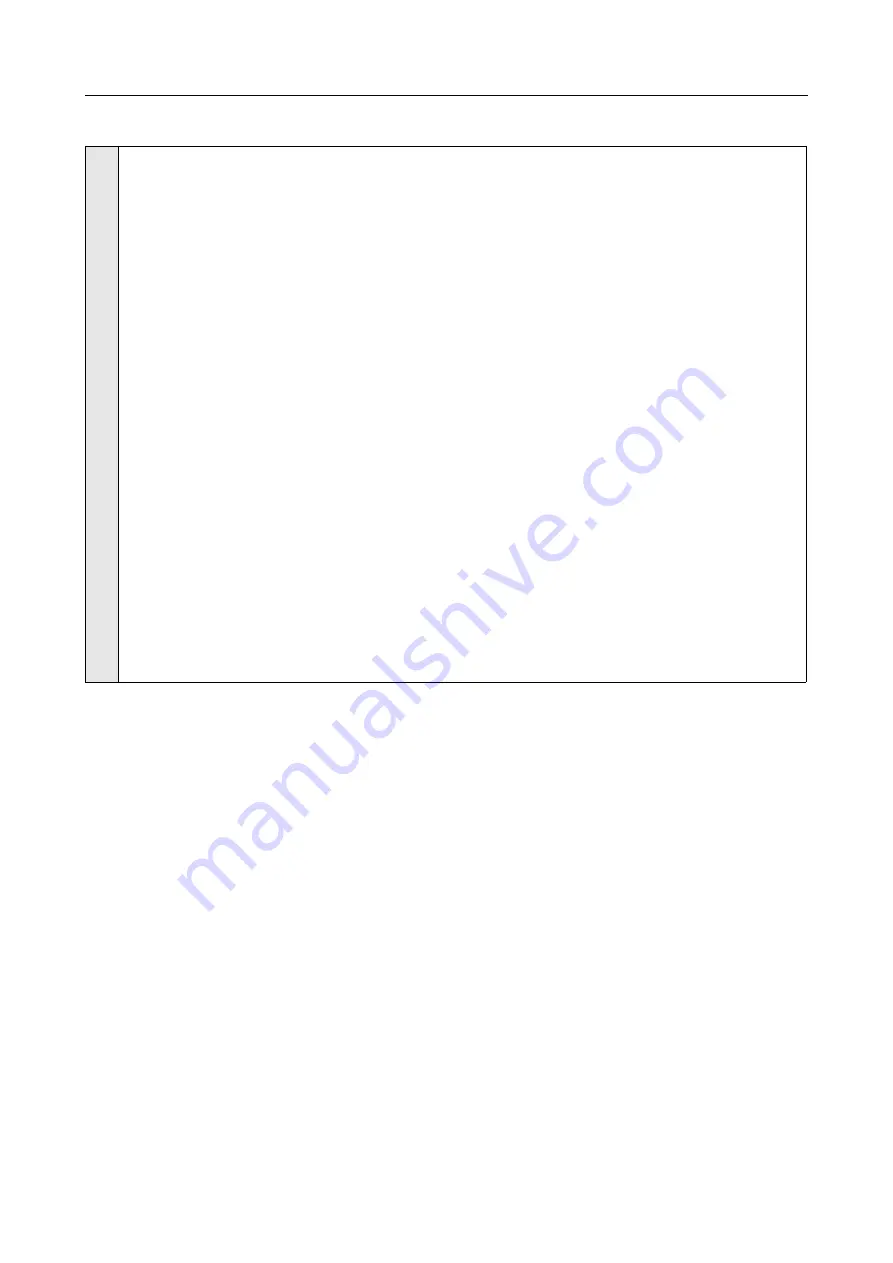
RP6 ROBOT SYSTEM - 4. Programming the RP6
This example shows how a very simple slave program could look like:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
#include "RP6RobotBaseLib.h"
#include "RP6I2CslaveTWI.h"
// Include the I2C Library file (!!!)
// ATTENTION: do not forget to add this to the Makefile (!!!)
#define CMD_SET_LEDS 3
// LED command, which should be received
// through the I2C Bus
int
main
(
void
)
{
initRobotBase
();
I2CTWI_initSlave
(
10
);
// Initialise TWI set slave address to 10
powerON
();
while
(
true
)
{
// did we receive some command and is there NO write access?
if
(
I2CTWI_writeRegisters
[
0
]
&&
!
I2CTWI_writeBusy
)
{
// save register contents:
uint8_t
cmd
=
I2CTWI_writeRegisters
[
0
];
I2CTWI_writeRegisters
[
0
]
=
0
;
// and reset cmd reg (!!!)
uint8_t
param
=
I2CTWI_writeRegisters
[
1
];
// Parameter
if
(
cmd
==
CMD_SET_LEDS
)
// LED command received?
setLEDs
(
param
);
// set LEDs with the parameter
}
if
(!
I2CTWI_readBusy
)
// No read activities?
// Proceed by writing current LED state to register 0:
I2CTWI_readRegisters
[
0
]
=
statusLEDs
.
byte
;
}
return
0
;
}
The program itself will not perform anything (visible), thus you need a master to con-
trol the slave device. In this case, the slave can be accessed with address 10 on the
I²C Bus (see line 10). It provides two registers for writing and one register for reading
data. The first register (= Register number 0) is used for receiving commands. This
simplified example uses the command “3” to set the LEDs (can be any number). On
reception of any command - and no write access (see line 16) - the program will
store the contents of command register 0 to the variable “cmd” (line 19) and reset the
command register 0 to prevent repeated command execution! The program proceeds
by storing the parameter from register 1 to another temporary variable and check the
reception of command 3 (line 23). If the comparison is true, the LEDs will be set by
applying the received parameter value.
Line 26 checks if there is no read access in progress and stores the current LED re-
gister value to the readable register 0.
When the Controller on the Mainboard is programmed like this, a Master controller can
set the LEDs on the Mainboard through the I²C Bus and read back their current state.
The program will not perform anything else – a more detailed example program,
which allows control of virtually all available robot funtions can be found on the CD.
The sample program above only demonstrates the basic principles.
Basically there are 16 writeable and 48 readable registers. Whoever needs more or
less registers may adapt the corresponding definitions in the RP6Library file RP6I2C-
SlaveTWI.h.
- 106 -