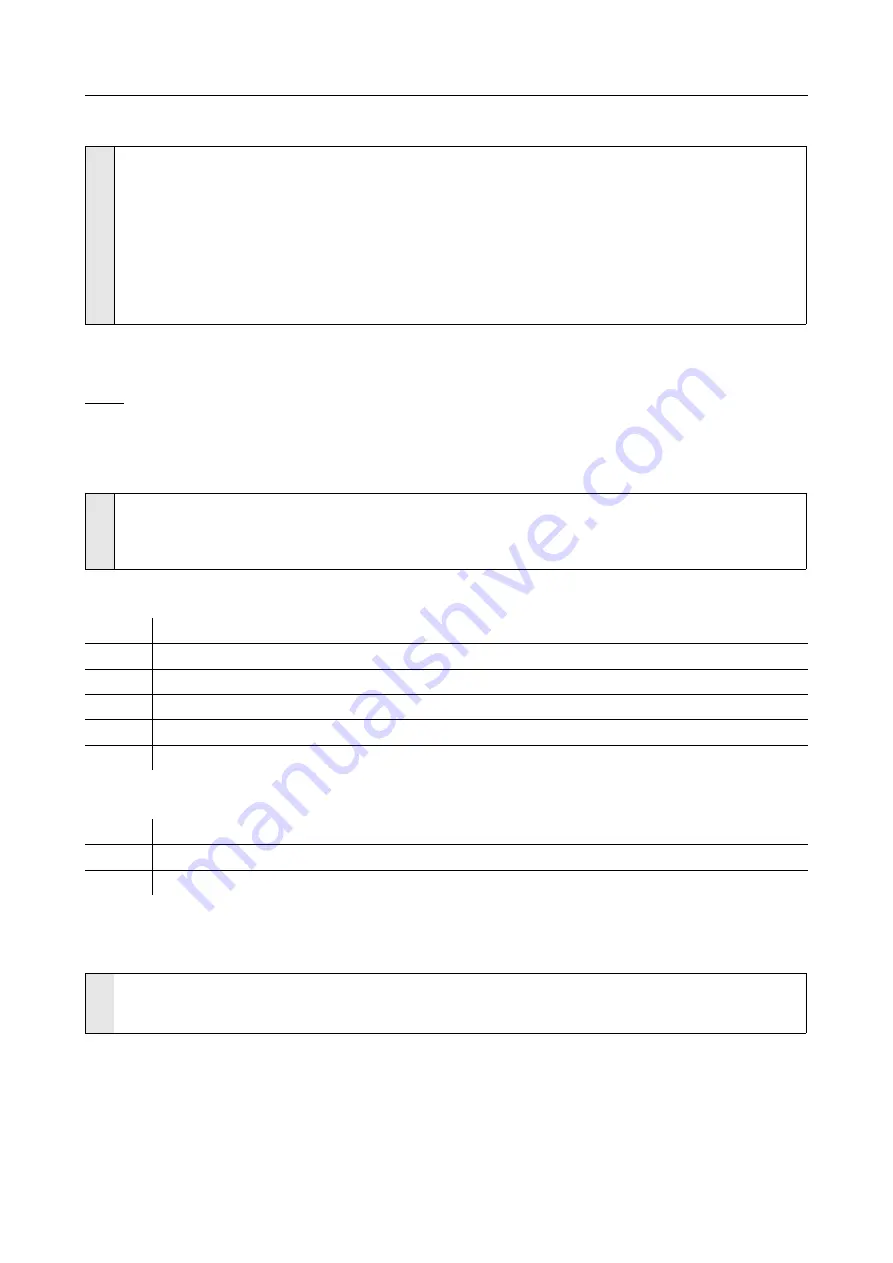
RP6 ROBOT SYSTEM - 4. Programming the RP6
One more example:
1
2
3
4
5
6
7
8
9
10
uint16_t
myFavoriteVariable
=
16447
;
if
(
myFavoriteVariable
<
16000
)
// If myFavoriteVariable < 16000
{
// then:
writeString
(
"myFavoriteVariable is less than 16000!\n"
);
}
else
// else:
{
writeString
(
"myFavoriteVariable is greater than or equal to 16000!\n"
);
}
“myFavoriteVariable” is set to
16447, which will result in an output “myFavoriteVari-
able is greater than or equal to 16000!“. In this example, the conditional statement is
false and will cause the else-branch to be executed.
As you can see on the name “myFavoriteVariable”, you can use all names for your
variables you can think of, as long as they meet the naming conventions.
We may also use If-then-else-constructs to create complex conditional branches:
1
2
3
4
if
(
x
==
1
)
{
writeString
(
"x is 1!\n"
);
}
else
if
(
x
==
5
)
{
writeString
(
"x is 5!\n"
);
}
else
if
(
x
==
244
)
{
writeString
(
"x is 244!\n"
);
}
else
{
writeString
(
"x has a different value!\n"
);}
Conditional statements may be using the following comparison operators:
x == y Logical comparison for equality
x != y Logical comparison for inequality
x < y Logical comparison for “less than”
x <= y Logical comparison for “less than or equal to”
x > y Logical comparison for “greater than”
x >= y Logical comparison for “greater than or equal to”
Additionally the language provides logical conjunctions:
x && y true, if x is true and y is true
x || y true, if x is true and/or y is true
!x
true, if x is false
We are allowed to link, to combine and nest these structures by using conjunctions
and any number of accolade-pairs:
1
1
2
if
( ((
x
!=
0
)
&&
(!(
x
>
10
))) || (y
>
=
200
)) {
writeString
(
"OK!\n"
);
}
The previously listed conditional statement is true, if x is not equal to zero
(x != 0)
AND
x
is
not greater than 10
(!(x > 10))
OR
if y is greater than or equal to 200
(y
>= 200)
. If necessary we could add any number of other conditions, as required in our
program.
- 69 -