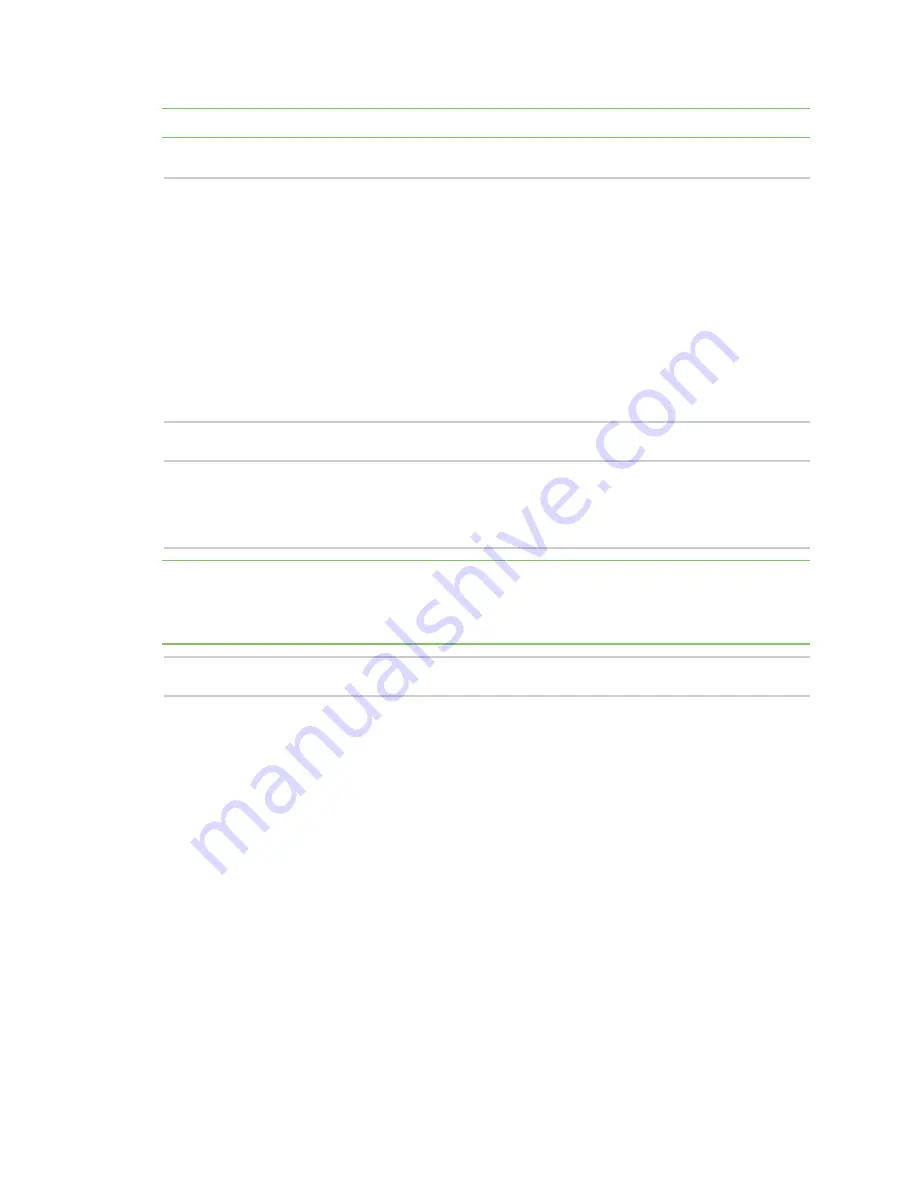
Get started with MicroPython
MicroPython examples
Digi XBee® 3 802.15.4 RF Module User Guide
41
Note
The
xbee.atcmd()
method does not support the following AT commands:
IS
,
AS
,
ED
,
ND
, or
DN
.
The following is example code that queries and sets a variety of AT commands using
xbee.atcmd()
:
import xbee
# Set the NI string of the radio
xbee.atcmd("NI", "XBee3 module")
# Configure a destination address using two different data types
xbee.atcmd("DH", 0x0013A200)
# Hex
xbee.atcmd("DL", b'\x12\x25\x89\xF5')
# Bytes
# Read some AT commands and display the value and data type:
print("\nAT command parameter values:")
commands =["DH", "DL", "NI", "CK"]
for cmd in commands:
val = xbee.atcmd(cmd)
print("{}: {:20} of type {}".format(cmd, repr(val), type(val)))
This example code outputs the following:
AT command parameter values:
DH: b'\x00\x13\xa2\x00'
of type <class 'bytes'>
DL: b'\x12%\x89\xf5'
of type <class 'bytes'>
NI: 'XBee3 module'
of type <class 'str'>
CK: 65535
of type <class 'int'>
Note
Parameters that store values larger than 16-bits in length are represented as bytes. Python
attempts to print out ASCII characters whenever possible, which can result in some unexpected
output (such as the "%" in the above output). If you want the output from MicroPython to match
XCTU, you can use the following example to convert bytes to hex:
dl_value = xbee.atcmd("DL")
hex_dl_value = hex(int.from_bytes(dl_value, 'big'))
MicroPython networking and communication examples
This section provides networking and communication examples for using MicroPython with the XBee 3
802.15.4 RF Module.
802.15.4 networks with MicroPython
For small networks, it is suitable to use MicroPython on every node. However, there are some inherit
limitations that may prevent you from using MicroPython on some heavily trafficked nodes:
n
When running MicroPython, any received messages will be stored in a small receive queue. This
queue only has room for 4 packets and must be regularly read to prevent data loss. For
networks that will be generating a lot of traffic, the data aggregator may need to operate in
API mode in order to capture all incoming data.
For the examples in this section, the devices should be pre-configured with identical network settings
so that RF communication is possible. To follow the upcoming examples, we need to configure a
second XBee 3 802.15.4 RF Module to use MicroPython.
XCTU only allows a single MicroPython terminal. We will be running example code on both modules,
which requires a second terminal window.