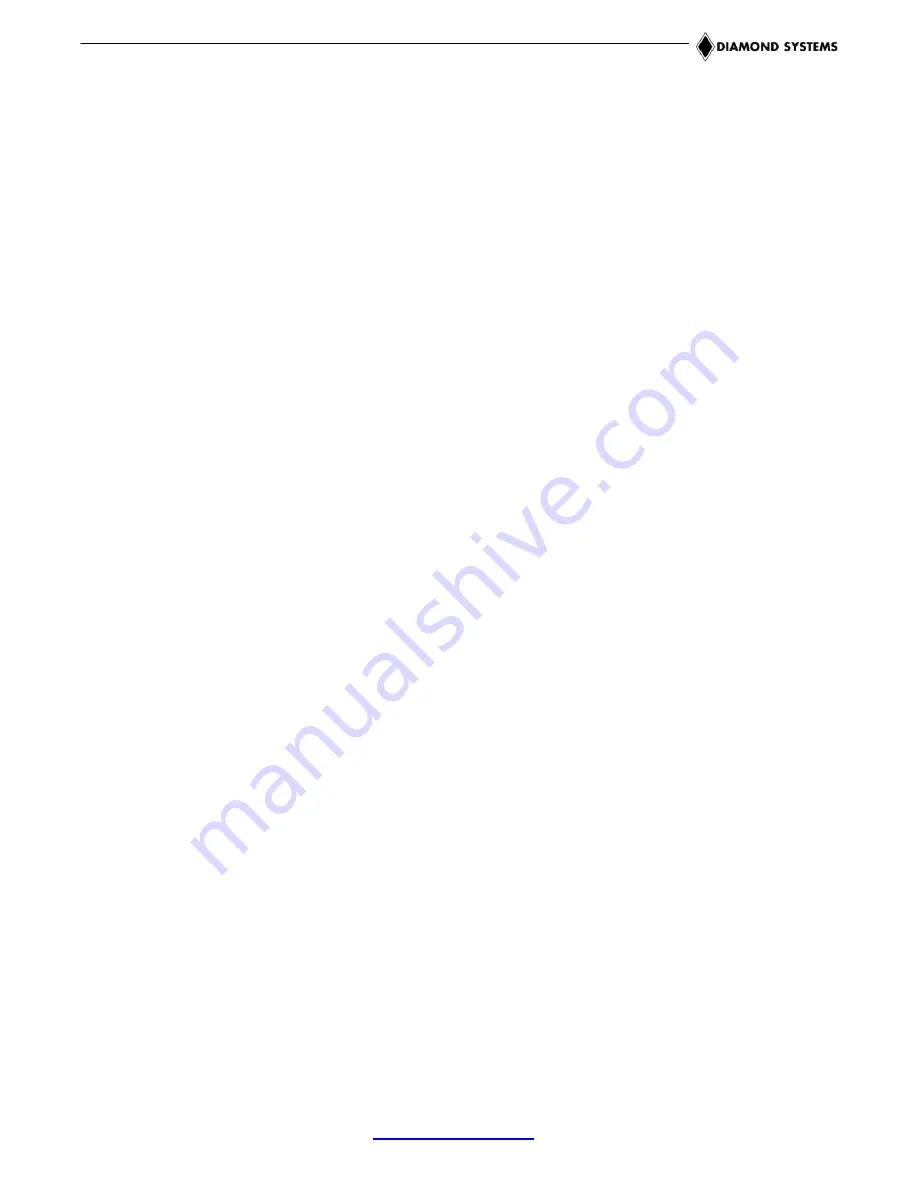
Athena III User Manual Rev A.03
www.diamondsystems.com
Page
63
14.6
Wait for the Conversion to Finish
The A/D converter chip takes up to five microseconds to complete one A/D conversion. Most processors and
software can operate fast enough so that if you try to read the A/D converter immediately after starting the
conversion, the read will occur faster than the A/D conversion and return invalid data. Therefore, the A/D
converter provides a status signal to indicate whether it is busy or idle. This bit can be read back from the status
register at Base+3, bit 7. When the A/D converter is busy (performing an A/D conversion), the bit value is 1 and
the program must wait. When the A/D converter is idle (conversion is done and data is available), this bit value is
0 and the program may read the data.
The following statement is a simple example of this operation.
while (inp(base+3) & 0x80); // Wait for conversion to finish before proceeding
The above example could hang your program if there is a hardware fault and the bit is stuck at 1. A better
solution is to use a loop with a timeout, as shown below.
int checkstatus() // returns 0 if ok, -1 if error
int i;
for (i = 0; i < 10000; i++)
{
if !(inp(base+3) & 0x80) then return(0);
// conversion completed
}
return(-1);
// conversion did not complete
14.7
Read the Data from the Board
Once the conversion is complete, you can read the data back from the A/D converter. The data is a 16-bit value
and is read back in two 8-bit bytes. The LSB must be read from the board before the MSB because the data is
inserted into the board’s FIFO in that order. Unlike other registers on the board, the A/D data may only be read
one time, because each time a byte is read from the FIFO the internal FIFO pointer advances and that byte is no
longer available. Reading data from an empty FIFO returns unpredictable results.
The following pseudo-code illustrates how to read and construct the 16-bit A/D value.
LSB = inp(base);
MSB = inp(base+1);
Data = MSB * 256 + LSB; // combine the 2 bytes into a 16-bit value
The final data are interpreted as a 16-bit signed integer in the range -32768 to +32767.
Note:
The data range always includes both positive and negative values, even if the board is set to a
unipolar input range. The data must now be converted to volts or other engineering units by using a
conversion formula, as discussed below.
In scan mode, the behavior is the same except when the program initiates a conversion, all channels in the
programmed channel range will be sampled once and the data will be stored in the FIFO. The FIFO depth
register increments by the scan size. When STS goes low, the program should read out the data for all channels.