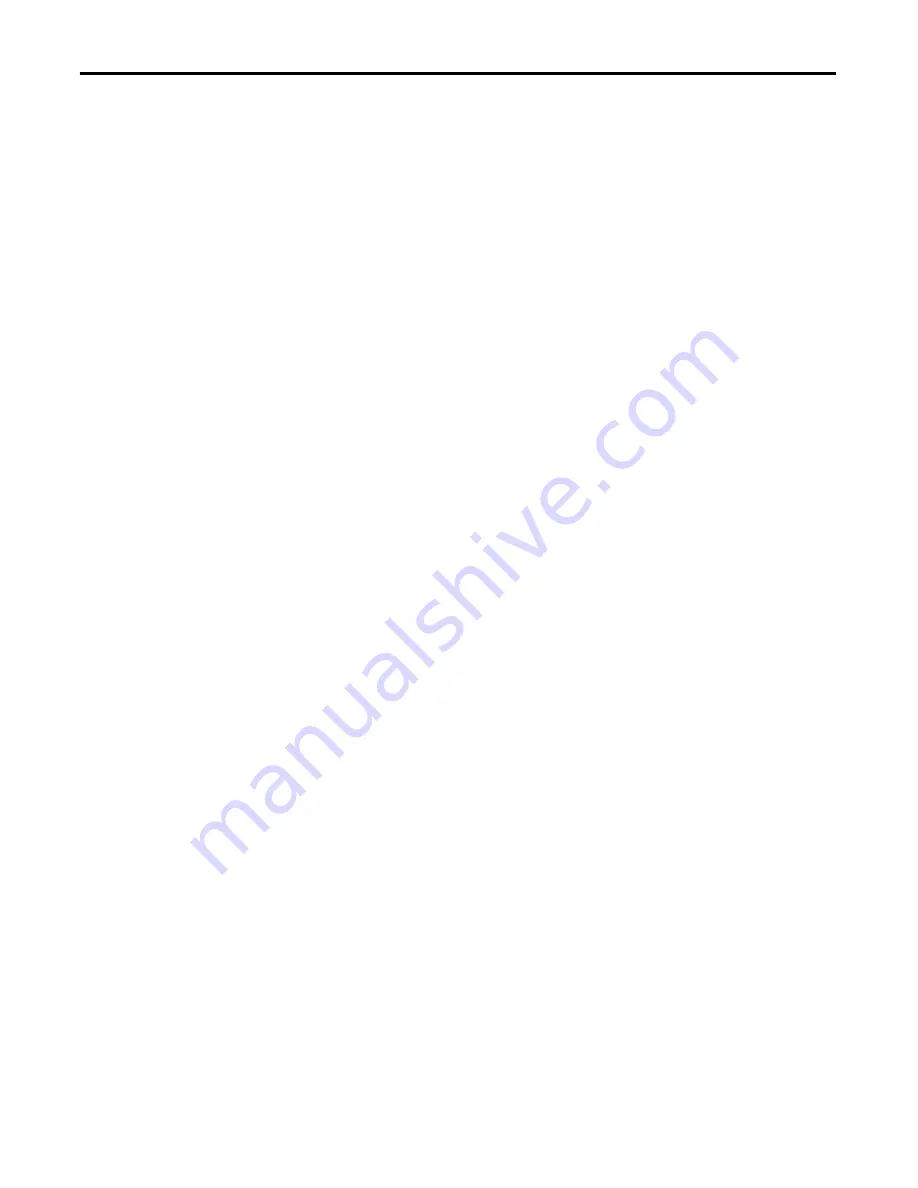
132
Rockwell Automation Publication 1789-UM002K-EN-P - January 2015
Chapter 7
Develop External Routines
// By building the sound resourced into the dll and doing a load
// library on the dll we ensure that the resources are written
// down to the controller with the dll. This means that we do not
// have to worry about copying the resources to the controller on our own.
HINSTANCE MyLib = LoadLibrary("Sounds.dll");
if (MyLib == NULL)
{
return;
}
hRes = FindResource(MyLib, Snd, "SOUNDS");
if (hRes == NULL)
{
return;
}
hResLoad = LoadResource(MyLib, hRes);
if (hResLoad == NULL)
{
return;
}
lpResLock = (LPTSTR)LockResource(hResLoad);
if (lpResLock == NULL)
{
return;
}
sndPlaySound(lpResLock, SND_SYNC | SND_MEMORY);
FreeLibrary(MyLib);
}
void RungStateThread(void *p )
{
bool
exitThread = FALSE;
rungStates
oldRungState = rungState;
DWORD
status;
HANDLE
hController = p;
HANDLE
hArrayHandles [2];
hArrayHandles[0] = hController;
hArrayHandles[1] = hTerminate; // used to check if thread should terminate.
while (!exitThread)
{
// This example uses an arbitrary timeout of 5 seconds
// to determine whether the controller is still in run mode.
// If this time expires, we can assume that the controller
// is no longer in run mode, and we can terminate this thread.
// Note: The 5000 millisecond timeout value can be adjusted
// to a value that fits the requirements for your specific
// application.
status = WaitForMultipleObjects (2, hArrayHandles, FALSE, 5000);
switch (status)
{
case WAIT_OBJECT_0: