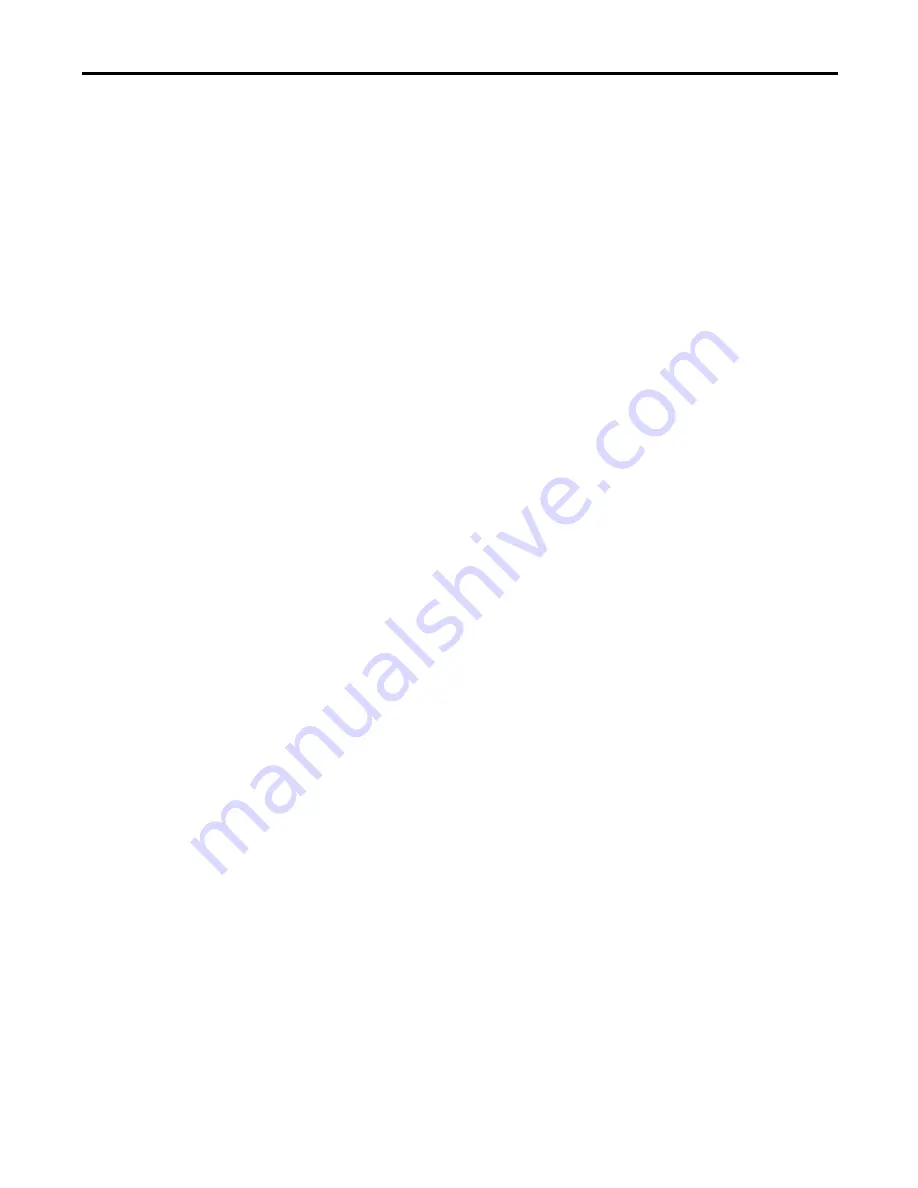
Rockwell Automation Publication 1789-UM002K-EN-P - January 2015
119
Develop External Routines
Chapter 7
RA_ExternalRoutines.h
#ifndef __RA_EXTROUTINE_H__
#define __RA_EXTROUTINE_H__
#define MAX_PARAMS
10
/*
MSC assumes LSB first, 32 bit integers */
#pragma pack(push,1)
struct RoutineControlWord
// 4 bytes (32 bit word) total
{
unsigned ErrorCode : 8;
// Error code if ER bit is set.
// -- end byte 0 --
unsigned NumParams : 8;
// From 0 to MAX_PARAMS,
// -- end byte 1 --
excludes control structure
unsigned ScanType : 2;
// 16-17 Normal, Pre, Post (0, 1, 2)
unsigned ReservedA : 2;
// 18-19 Reserved Set A: DO NOT USE
unsigned User : 2;
// 20-21 Defined by Ext Rtn developer
unsigned ReservedC : 2;
// 22-23 Reserved Set C: DO NOT USE
// -- end byte2 --
unsigned EnableIn : 1;
// 24 Incoming rung status
unsigned EnableOut : 1;
// 25 Returning rung status
unsigned FirstScan : 1;
// 26 First Normal Scan occurring
unsigned ER : 1;
// 27 Control ERROR
unsigned ReservedB : 1;
// 28 Reserved Set B: DO NOT USE
unsigned DN : 1;
// 29 Control DONE
unsigned ReturnsValue : 1;
// 30 Indicates if routine returns anything
unsigned EN : 1;
// 31 Control ENABLE
// -- end byte 3 --
};
#pragma pack(pop)
enum EXT_ROUTINE_PARAM_TYPE_E // 4 bytes long
{
FloatingPointValue = 0,
// e.g., float p
FloatingPointAddress,
// e.g., float* p
IntegerValue,
// e.g., short p
IntegerAddress,
// e.g., long* p
ArrayAddress,
// e.g., int p[]
StructureAddress,
// e.g., MyStructT* p
VoidAddress,
// e.g., void* p
Void,
// e.g., "No return value"
LastEntryInEnum
};
// Structure representing the type of the parameter defined
// for the External Routine.
struct EXT_ROUTINE_PARAMETERS
// 12 bytes long
{
// Size of parameter/array element in bits
unsigned long bitsPerElement;
// If array, number of elements else 1.
unsigned long numberOfElements;
// Numeric representation and reference type.
EXT_ROUTINE_PARAM_TYPE_E paramType;
};