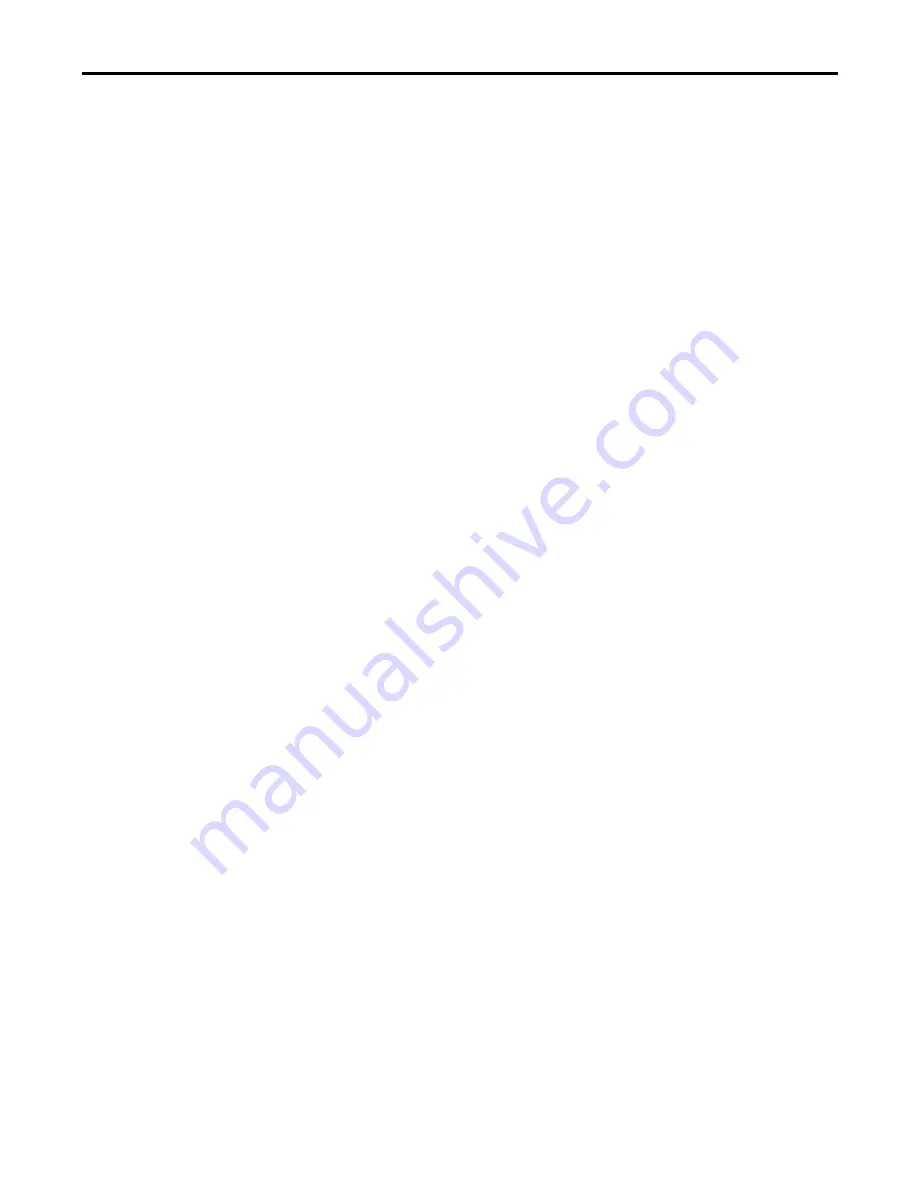
Rockwell Automation Publication 1789-UM002K-EN-P - January 2015
121
Develop External Routines
Chapter 7
InlineExample.cpp
// InlineExample.cpp : Defines the entry point for the DLL application.
#include "stdafx.h"
//Include file for External Routine interface
#include "RA_ExternalRoutines.h"
BOOL APIENTRY DllMain( HANDLE hModule,
DWORD ul_reason_for_call,
LPVOID lpReserved
)
{
switch (ul_reason_for_call)
{
case DLL_PROCESS_ATTACH:
case DLL_THREAD_ATTACH:
case DLL_THREAD_DETACH:
case DLL_PROCESS_DETACH:
break;
}
return TRUE;
}
// This is an example of an exported function.
extern "C" __declspec(dllexport
int SumArray(
EXT_ROUTINE_CONTROL* pERCtrl,
int Val[])
{
// Add all array elements provided, then return the Sum.
pERCtrl->ctrlWord.EN = pERCtrl->ctrlWord.EnableIn;
pERCtrl->ctrlWord.EnableOut = pERCtrl->ctrlWord.EnableIn;
BOOL bFail = FALSE;
// Number of parameters expected is 1 (exclude ctrl struct*), and
// Check type of parameter against what is expected, and
// Check size of array elements to make sure they agree with int type.
if (pERCtrl->ctrlWord.NumParams != 1)
bFail = TRUE;
else if ( (pERCtrl->paramDefs[0].paramType != ArrayAddress) ||
(pERCtrl->paramDefs[0].bitsPerElement != 8*sizeof(int)) )
bFail = TRUE;
// Check number of array elements
int nNoElems = pERCtrl->paramDefs[0].numberOfElements;
if (nNoElems == 0)
bFail = TRUE;
int itemp = 0; // Initialize sum to zero
if (pERCtrl->ctrlWord.EnableIn)
{
// Rung enabled, run the function's implementation
if (!bFail)
{
// Sum all array elements
for (int j = 0; j < nNoElems; j++)
itemp += Val[j];