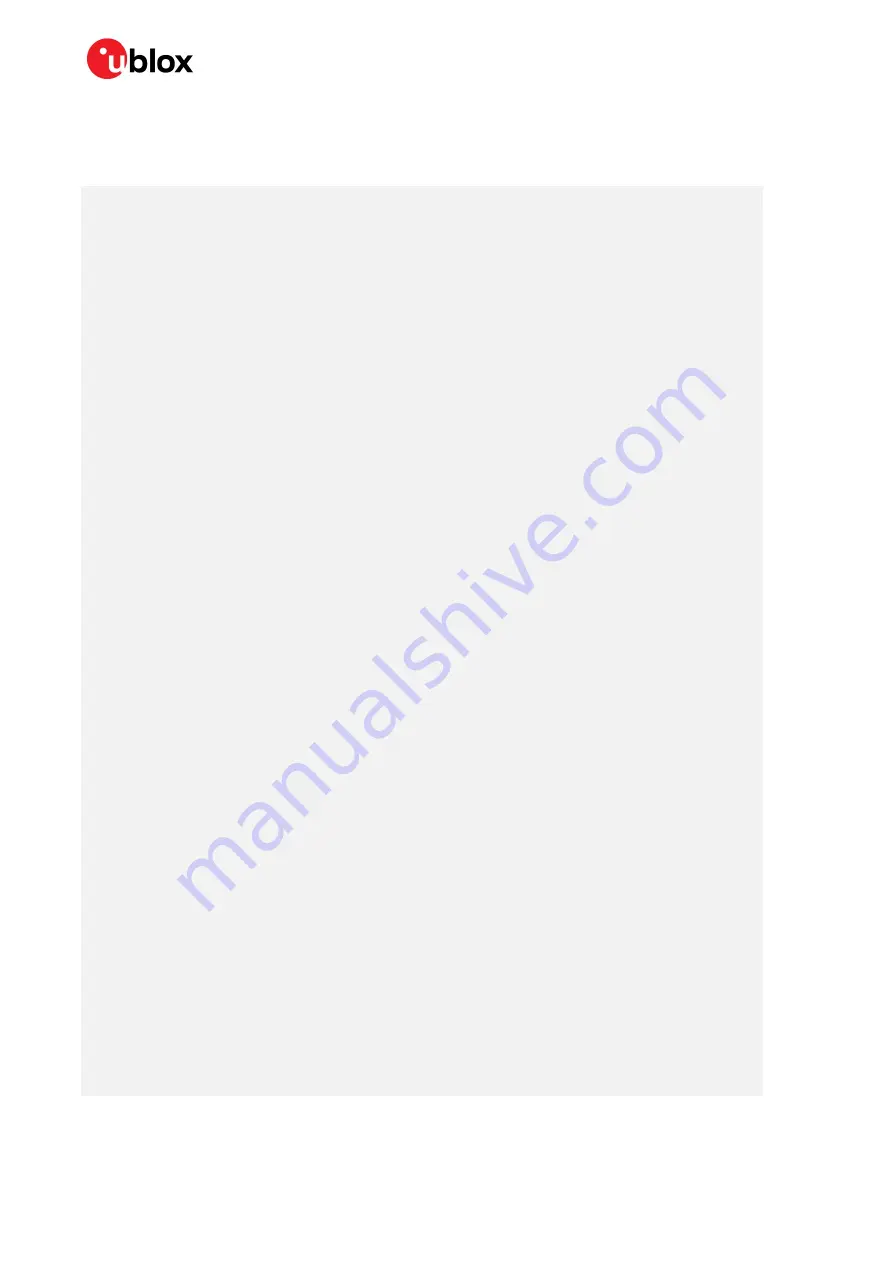
NINA-B1 series - System integration manual
UBX-15026175 - R16
Appendix
Page 56 of 63
C1-Public
C
XMODEM code example
Example that shows a basic XMODEM implementation and a CRC calculation in C#.
private
const
int
XMODEM_ACK
= 6;
private
const
int
XMODEM_NAK
= 21;
private
const
int
XMODEM_EOT
= 4;
public
Boolean
XModemSend
(
Stream
inputStream
)
{
byte
blockCnt
= 0;
Boolean
result
=
false
;
BufferedStream
bufferedInput
=
new
BufferedStream
(
inputStream
);
byte
[]
block
=
new
Byte
[133];
int
bytesRead
;
do
{
for
(
int
i
= 0;
i
< 133;
i
++) {
block
[
i
] = 0;
}
bytesRead
=
bufferedInput
.
Read
(
block
, 3, 128);
blockCnt
++;
block
[0] = 1;
block
[1] =
blockCnt
;
block
[2] = (
byte
)(255 -
blockCnt
);
ushort
crc
=
xmodemCalcrc
(
block
, 3, 128);
block
[131] = (
Byte
)((
crc
>> 8) & 0xFF);
block
[132] = (
Byte
)(
crc
& 0xFF);
try
{
this
.
Write
(
block
, 0,
block
.
Length
);
}
catch
(
Exception
) {
result
=
false
;
break
;
}
try
{
Byte
res
= (
Byte
)
this
.
ReadByte
();
while
(
res
==
'C'
) {
res
= (
Byte
)
this
.
ReadByte
();
}
if
(
res
==
XMODEM_ACK
) {
DebugPrint
(
“Bytes sent {0}”,
bytesRead
));
if
(
bytesRead
< 128) {
byte
[]
eot
=
new
byte
[1];
eot
[0] =
XMODEM_EOT
;
this
.
Write
(
eot
, 0, 1);
res
= (
Byte
)
this
.
ReadByte
();
if
(
res
==
XMODEM_ACK
) {
/* File sent ok */
result
=
true
;
}
else
{
result
=
false
;
}
}
}
else
{
// No ACK received, stop transmission no retransmission implemented
result
=
false
;
break
;
}
}
catch
(
TimeoutException
) {
result
=
false
;
break
;
}
}
while
(
bytesRead
== 128);
DebugPrint
(
“Bytes sent {0}”,
bytesRead
));
bufferedInput
.
Close
();