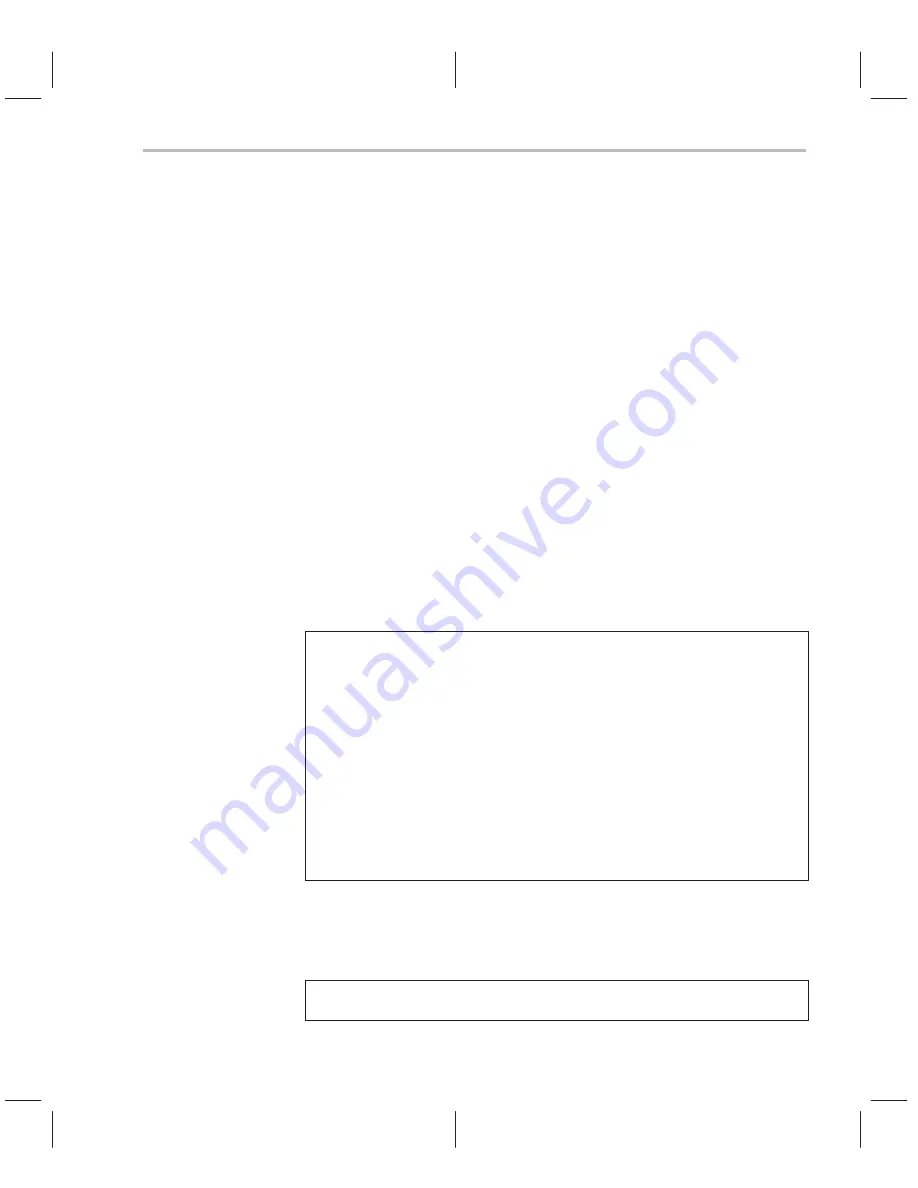
Refining C/C++ Code
3-15
Optimizing C/C++ Code
3.4
Refining C/C++ Code
You can realize substantial gains from the performance of your C/C++ code
by refining your code in the following areas:
-
Using intrinsics to replace complicated C/C++ code
-
Using word access to operate on 16-bit data stored in the high and low
parts of a 32-bit register
-
Using double access to operate on 32-bit data stored in a 64-bit register
pair (’C64x and ’C67x only)
3.4.1
Using Intrinsics
The ’C6000 compiler provides intrinsics, special functions that map directly to
inlined ’C62x/’C64x/’C67x instructions, to optimize your C/C++ code quickly.
All instructions that are not easily expressed in C/C++ code are supported as
intrinsics. Intrinsics are specified with a leading underscore ( _ ) and are ac-
cessed by calling them as you call a function.
For example, saturated addition can be expressed in C/C++ code only by writ-
ing a multicycle function, such as the one in Example 3–6.
Example 3–6. Saturated Add Without Intrinsics
int sadd(int a, int b)
{
int result;
result = a + b;
if (((a ^ b) & 0x80000000) == 0)
{
if ((result ^ a) & 0x80000000)
{
result = (a < 0) ? 0x80000000 : 0x7fffffff;
}
}
return (result);
}
This complicated code can be replaced by the _sadd( ) intrinsic, which results
in a single ’C6x instruction (see Example 3–7).
Example 3–7. Saturated Add With Intrinsics
result = _sadd(a,b);