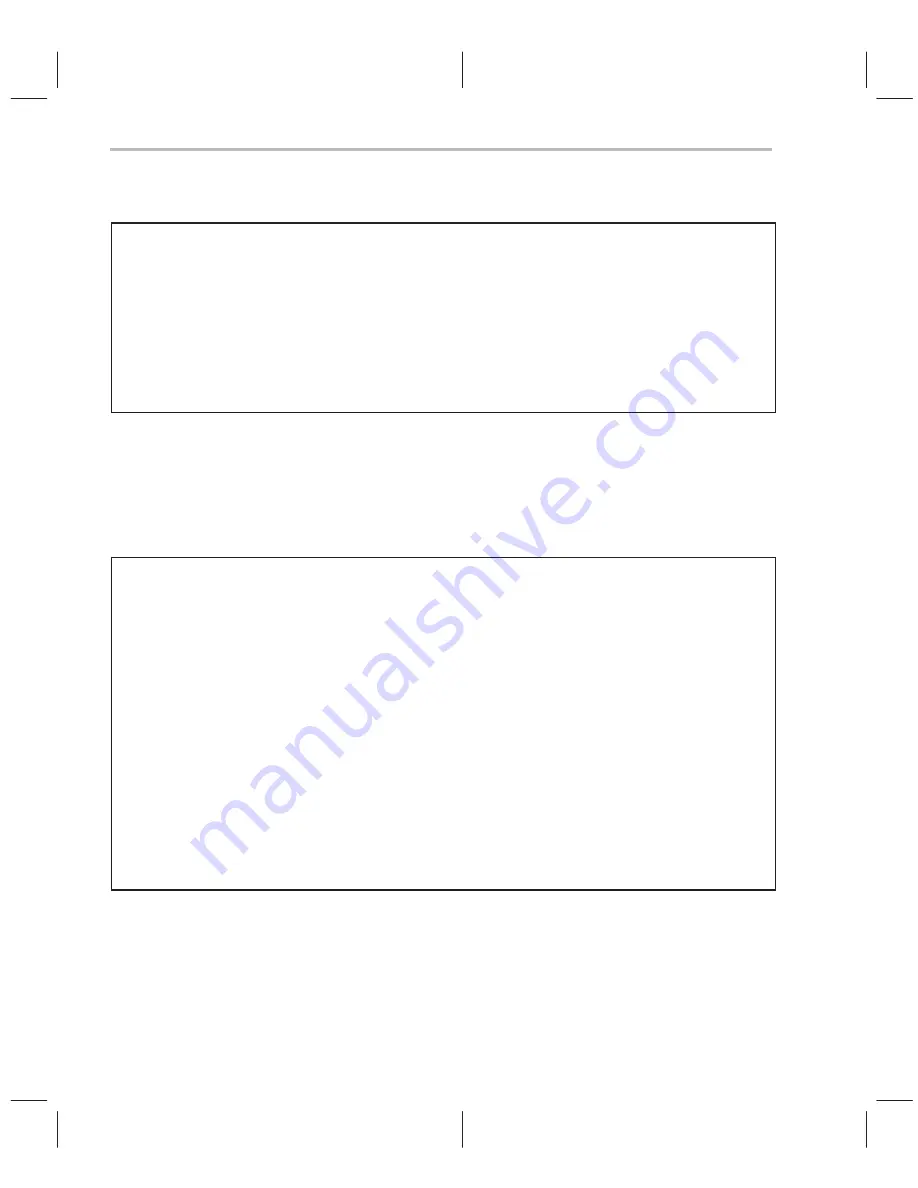
Linear Assembly Considerations
8-46
Example 8–18. Loop Trip Count in C
int count_loop_iterations(int count)
{
int iters, i;
iters = 0;
for (i = count; i > 0; i––)
iters++;
return iters;
}
Without BDEC and BPOS, this loop would be written as shown in
Example 8–19 below. This example uses branches to test whether the loop
iterates at all, as well as to perform the loop iteration itself. This loop iterates
exactly the number of times specified by the argument ’count’.
Example 8–19. Loop Trip Count in Linear Assembly without BDEC
.global _count_loop_iterations
_count_loop_iterations .cproc count
.reg i, iters, flag
ZERO iters ; Initialize our return value to 0.
CMPLT count, 1, flag
[flag] B does_not_iterate ; Do not iterate if count
MV count, i ; i = count
loop: .trip 1 ; This loop is guaranteed to iterate at
; least once.
ADD iters, 1, iters ; iters++
SUB i, 1, i ; i––
[i] B loop ; while (i > 0);
does_not_iterate:
.return iters ; Return our number of iterations.
.endproc
Using BDEC , the loop is written similarly. However, the loop counter needs to
be adjusted, since BDEC terminates the loop after the loop counter becomes
negative. Example 8–20 illustrates using BDEC to conditionally execute the
loop, as well as to iterate the loop. In the typical case, the loop count needs
to be decreased by 2 before the loop. The SUB and BDEC before the loop per-
form this update to the loop counter.