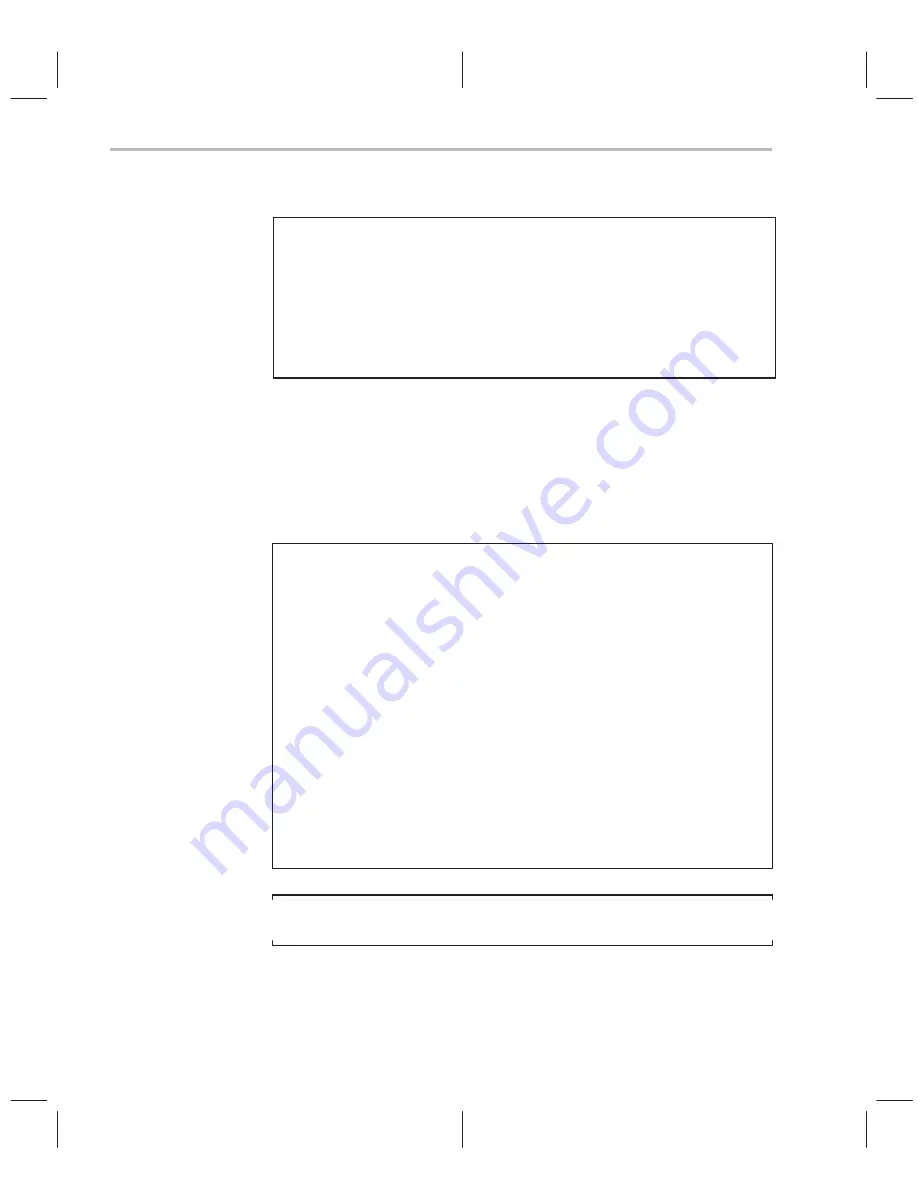
Compiling C/C++ Code
3-10
Example 3–3.Use of the Restrict Type Qualifier With Arrays
void func1(int c[restrict], int d[restrict])
{
int i;
for(i = 0; i < 64; i++)
{
c[i] += d[i];
d[i] += 1;
}
}
Do
not use the restrict keyword with code such as listed in Example 3–4. By
using the restrict keyword in Example 3–4, you are telling the compiler that it
is legal to write to any location pointed to by
a before reading the location
pointed to by
b. This can cause an incorrect program because both a and b
point to the same object —
array.
Example 3–4.Incorrect Use of the restrict Keyword
void func (short *a, short * restrict b)/*Bad!! */
{
int i;
for (i = 11; i < 44; i++) *(––a) = *(––b);
}
void main ()
{
short array[] = {
1, 2, 3, 4, 5, 6, 7, 8, 9, 10,
11, 12, 13, 14, 15, 16, 17, 18,
19, 20, 21, 22, 23, 24, 25, 26,
27, 28, 29, 30, 31, 32, 33, 34,
35, 36, 37, 38, 39, 40, 41, 42,
43, 44};
short *ptr1, *ptr2;
ptr2 = array + 44;
ptr1 = ptr2 – 11;
func(ptr2, ptr1);
/*Bad!! */
}
Note:
Do not use const to tell the compiler that two pointers do not point
to the same object. Use restrict instead.