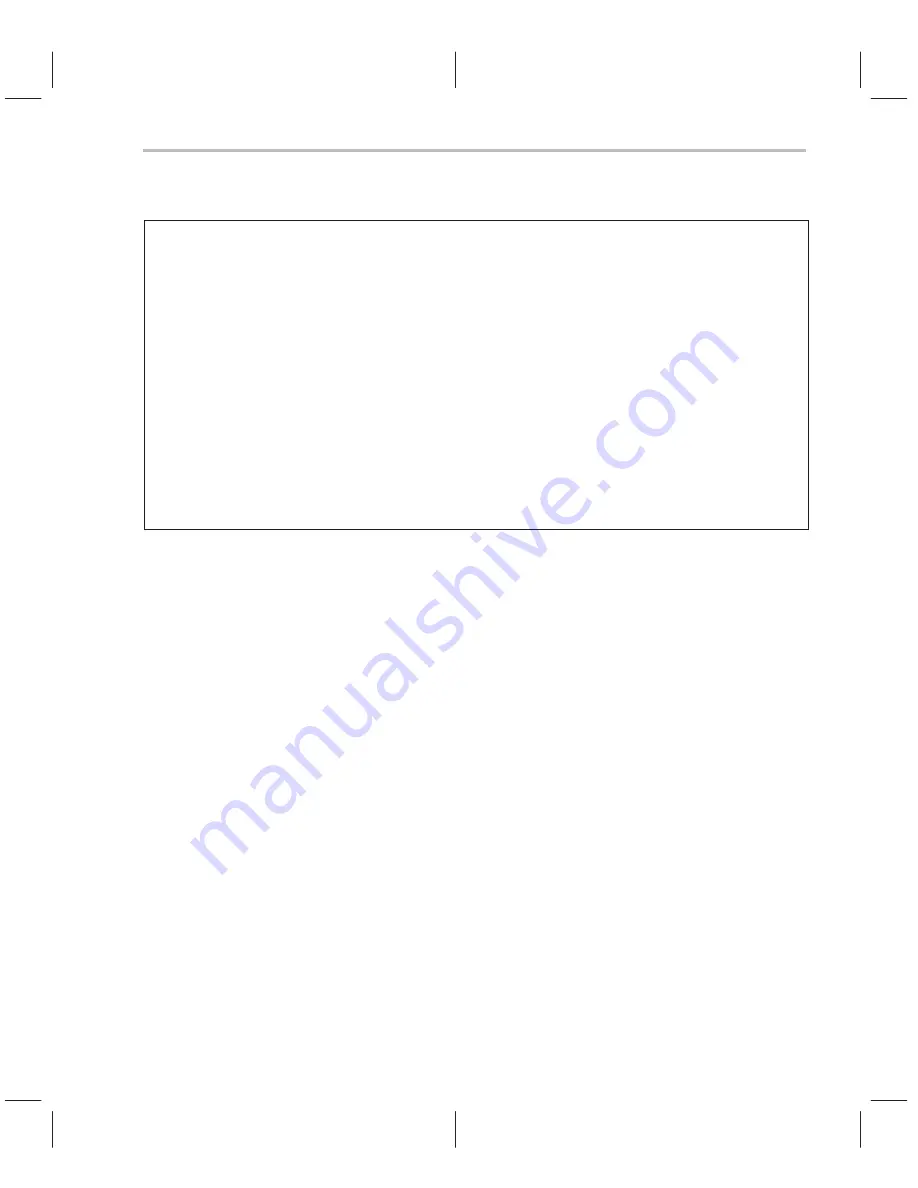
Refining C/C++ Code
3-51
Optimizing C/C++ Code
Example 3–33. Use of If Statements in Float Collision Detection (Modified Code)
int colldet_new(const float *restrict x, const float *restrict p, float
point, float distance)
{
int I, retval = 0;
float sum0, sum1, dist0, dist1;
for (I = 0; I < (28 * 3); I += 6)
{
sum0 = x[I+0]*p[0] + x[I+1]*p[1] + x[I+2]*p[2];
sum1 = x[I+3]*p[0] + x[I+4]*p[1] + x[I+5]*p[2];
dist0 = sum0 - point;
dist1 = sum1 - point;
dist0 = fabs(dist0);
dist1 = fabs(dist1);
if ((dist0<distance)&&!retval) retval = (int)&x[I+0];
if ((dist1<distance)&&!retval) retval = (int)&x[I+3];
}
return retval;
}
-
The loop cannot have an incrementing loop counter. Run the optimizer
with the –o2 or –o3 option to convert as many loops as possible into down-
counting loops.
-
If the trip counter is modified within the body of the loop, it typically cannot
be converted into a downcounting loop. If possible, rewrite the loop to not
modify the trip counter. For example, the following code will not software
pipeline:
for (i = 0; i < n; i++)
{
. . .
i += x;
}
-
A conditionally incremented loop control variable is not software pipelined.
Again, if possible, rewrite the loop to not conditionally modify the trip count-
er. For example the following code will not software pipeline:
for (i = 0; i < x; i++)
{
. . .
if (b > a)
i += 2
}
-
If the code size is too large and requires more than the 32 registers in the
‘C62x and ’C67x, or 64 registers on the ’C64x, it is not software pipelined.
Either try to simplify the loop or break the loop up into multiple smaller
loops.